TableView
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Header
#include <gui/tableview.h>
Functions
TableViews are data views that display tabulated information arranged in rows and columns (Figure 1), (Figure 2), (Figure 3). The control enables scroll bars and allows keyboard navigation. In Hello TableView! you have an example of use.
- Use tableview_create to create a table view.
- Use tableview_new_column_text to add a column.
- Use tableview_size to set the default size.
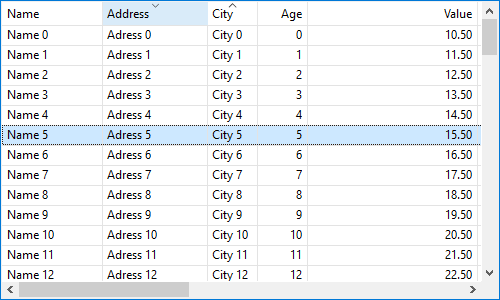
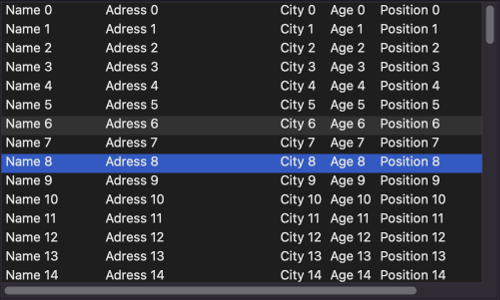
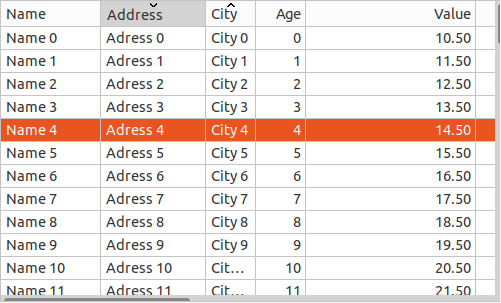
1. Data connection
Let's think that a table can contain thousands of records and these can change at any time from different data sources (disk, network, DBMS, etc). For this reason, the TableView will not maintain any internal cache. It has been designed with the aim of making a quick visualization of the data, but without going into their management. Ultimately, it is the application that must provide this information in a fluid manner.
- Use tableview_OnData to bind the table to the data source.
- Use tableview_update to force an update of the table data.
When a table needs to draw its contents, in response to an OnDraw
event, it will first ask the application for the total number of records via a ekGUI_EVENT_TBL_NROWS notification. With this it can calculate the size of the document and configure the scroll bars (Figure 4). Subsequently, it will launch successive ekGUI_EVENT_TBL_CELL events, where it will ask the application for the content of each cell (Figure 5). All these requests will be made through the callback function set in tableview_OnData (Listing 1).
TableView will only ask for the content of the visible part at any time.
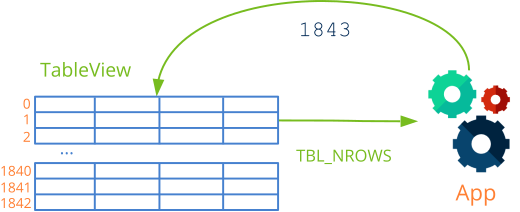
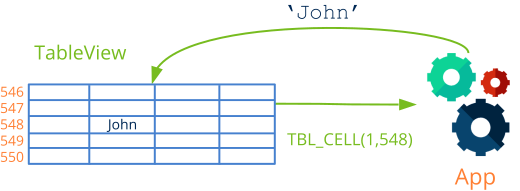
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
static void i_OnTableData(App *app, Event *e) { uint32_t etype = event_type(e); unref(app); switch(etype) { case ekGUI_EVENT_TBL_NROWS: { uint32_t *n = event_result(e, uint32_t); *n = app_num_rows(app); break; } case ekGUI_EVENT_TBL_CELL: { const EvTbPos *pos = event_params(e, EvTbPos); EvTbCell *cell = event_result(e, EvTbCell); switch(pos->col) { case 0: cell->text = app_text_column0(app, pos->row); break; case 1: cell->text = app_text_column1(app, pos->row); break; case 2: cell->text = app_text_column2(app, pos->row); break; } break; } } } TableView *table = tableview_create(); tableview_OnData(table, listener(app, i_OnTableData, App)); tableview_update(table); |
2. Data cache
As we have already commented, at each instant the table will only show a small portion of the data set. In order to supply this data in the fastest possible way, the application can keep a cache with those that will be displayed next. To do this, before starting to draw the view, the table will send an ekGUI_EVENT_TBL_BEGIN type event where it will indicate the range of rows and columns that need updating (Figure 6). This event will precede any ekGUI_EVENT_TBL_CELL seen in the previous section. In the same way, once all the visible cells have been updated, the ekGUI_EVENT_TBL_END event will be sent, where the application will be able to free the resources in the cache (Listing 2).
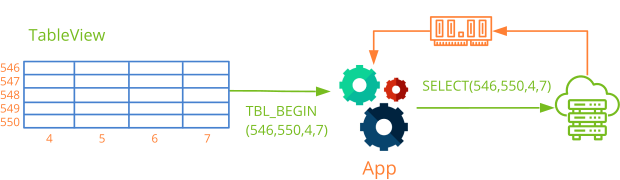
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
static void i_OnTableData(App *app, Event *e) { uint32_t etype = event_type(e); unref(app); switch(etype) { case ekGUI_EVENT_TBL_NROWS: { uint32_t *n = event_result(e, uint32_t); *n = app_num_rows(app); break; } case ekGUI_EVENT_TBL_BEGIN: { const EvTbRect *rect = event_params(e, EvTbRect); app->cache = app_fill_cache(app, rect->strow, rect->edrow, rect->stcol, rect->edcol); break; } case ekGUI_EVENT_TBL_CELL: { const EvTbPos *pos = event_params(e, EvTbPos); EvTbCell *cell = event_result(e, EvTbCell); cell->text = app_get_cache(app->cache, pos->row, pos->col); break; } case ekGUI_EVENT_TBL_END: app_delete_cache(app->cache); break; } TableView *table = tableview_create(); tableview_OnData(table, listener(app, i_OnTableData, App)); tableview_update(table); |
3. Multiple selection
When we navigate through a TableView
we can activate the multiple selection, which will allow us to mark more than one row of the table (Figure 7).
- Use tableview_multisel to turn multiselect on or off.
- Use tableview_selected to get the selected rows.
- Use tableview_select to select a set of rows.
- Use tableview_deselect to deselect.
- Use tableview_deselect_all to uncheck all rows.
- Use tableview_OnSelect to receive an event when the selection changes.
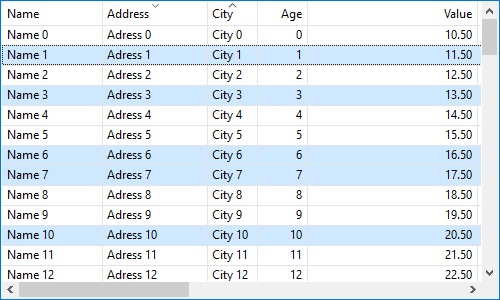
4. Table navigation
Navigating a TableView
works the same as other similar controls, such as the file explorer. We can use the keyboard when the table has focus. It will also respond to mouse events to select rows and move scroll bars.
- Use tableview_focus_row to move keyboard focus to a row.
- Use tableview_get_focus_row to get the row that has keyboard focus.
- Use tableview_hkey_scroll to set horizontal scrolling.
[UP]/[DOWN]
to move row by row.[LEFT]/[RIGHT]
to scroll horizontally.[PAGEUP]/[PAGEDOWN]
advance or reverse a page.[HOME]
goes to the beginning of the table.[END]
goes to the end of the table.[CTRL]+click
multiple selection with the mouse.[SHIFT]+[UP]/[DOWN]
multiple selection with the keyboard.
In multiple selection, an automatic de-selection of the rows will occur whenever we click releasing [CTRL]
or press any navigation key releasing [SHIFT]
. If we want to navigate without losing the previous selection, we must activate the preserve
flag in tableview_multisel.
5. Configure columns
We have different options to configure the interaction with the different columns of the table:
- Use tableview_header_title to set the title of a column. Multiple lines are accepted including
'\n'
characters (Figure 9). - Use tableview_header_align to set the alignment of a column header.
- Use tableview_header_resizable to allow or disallow column resizing.
- Use tableview_column_width to set the width of a column.
- Use tableview_column_limits to set limits on the width.
- Use tableview_column_resizable to allow the column to be stretched or collapsed.
- Use tableview_column_freeze to freeze columns (Figure 8).
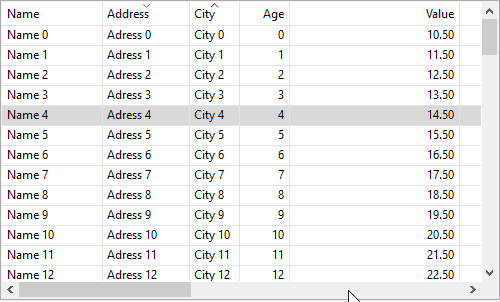
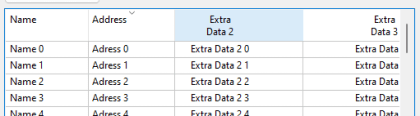
6. Notifications in tables
We have different events to capture actions that the user could perform on the (Listing 3) table.
- Use tableview_header_clickable to allow clicking on the header.
- Use tableview_OnRowClick to notify the click on a row.
- Use tableview_OnHeaderClick to notify the click on the header.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
static void i_OnRowClick(App *app, Event *e) { const EvTbRow *p = event_params(e, EvRow); on_row_click(app, p->row, p->sel); } static void i_OnHeaderClick(App *app, Event *e) { const EvButton *p = event_params(e, EvButton); on_header_click(app, p->index); } tableview_OnRowClick(table, listener(app, i_OnRowClick, App)); tableview_OnHeaderClick(table, listener(app, i_OnRowClick, App)); |
7. Table appearance
There are different options to change the appearance of the table.
- Use tableview_font to change the font.
- Use tableview_header_visible to show or hide the header.
- Use tableview_scroll_visible to show or hide the scroll bars.
- Use tableview_grid to show or hide the inner lines (Figure 10), (Figure 11).
- Use tableview_header_height to force the header height.
- Use tableview_row_height to force the row height.
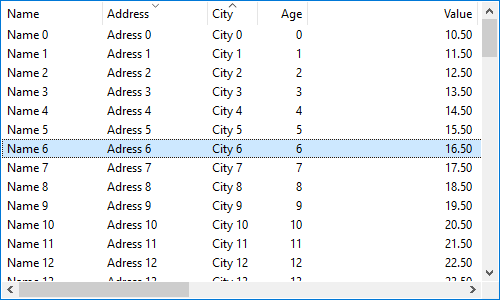
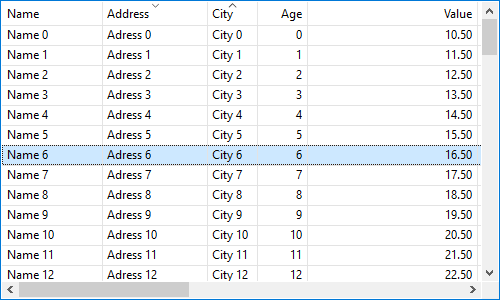
tableview_create ()
Creates a new table view.
TableView* tableview_create(void);
Return
The table.
tableview_OnData ()
Sets up a handler to read data from the application.
void tableview_OnData(TableView *view, Listener *listener);
view | The table. |
listener | A callback function that will be called each time the table needs to update its content. |
Remarks
See Data connection.
tableview_OnSelect ()
Notifies that the selection has changed.
void tableview_OnSelect(TableView *view, Listener *listener);
view | The table. |
listener | A callback function that will be called whenever the selection in the table changes. |
Remarks
See Multiple selection.
tableview_OnRowClick ()
Notify each time a row is clicked.
void tableview_OnRowClick(TableView *view, Listener *listener);
view | The table. |
listener | Callback function that will be called every time a row is clicked. |
Remarks
tableview_OnHeaderClick ()
Notifies each time a header is clicked.
void tableview_OnHeaderClick(TableView *view, Listener *listener);
view | The table. |
listener | Callback function that will be called every time a table header is clicked. |
Remarks
tableview_font ()
Sets the general font for the entire table.
void tableview_font(TableView *view, const Font *font);
view | The table. |
font | Font. |
Remarks
See Table appearance.
tableview_size ()
Sets the default size of the table control.
void tableview_size(TableView *view, const S2Df size);
view | The table. |
size | The size. |
Remarks
Corresponds to the Natural sizing of the control. By default 256x128.
tableview_new_column_text ()
Adds a new column to the table.
uint32_t tableview_new_column_text(TableView *view);
view | The table. |
Return
The column identifier (index).
Remarks
See Configure columns.
tableview_column_width ()
Sets the width of a column.
void tableview_column_width(TableView *view, const uint32_t column_id, const real32_t width);
view | The table. |
column_id | The column id. |
width | The column width. |
Remarks
See Configure columns.
tableview_column_limits ()
Sets the size limits of a column.
void tableview_column_limits(TableView *view, const uint32_t column_id, const real32_t min, const real32_t max);
view | The table. |
column_id | The column id. |
min | The minimum width. |
max | The maximum width. |
Remarks
See Configure columns.
tableview_column_align ()
Sets the default text alignment for the column data.
void tableview_column_align(TableView *view, const uint32_t column_id, const align_t align);
view | The table. |
column_id | The column id. |
align | The alignment. |
Remarks
See Configure columns.
tableview_column_resizable ()
Sets whether a column is resizable or not.
void tableview_column_resizable(TableView *view, const uint32_t column_id, const bool_t resizable);
view | The table. |
column_id | The column id. |
resizable |
|
Remarks
See Configure columns.
tableview_column_freeze ()
Allows to freeze the first columns of the table. During horizontal movement they will remain fixed.
void tableview_column_freeze(TableView *view, const uint32_t last_column_id);
view | The table. |
last_column_id | The identifier of the last column set. |
Remarks
See Configure columns.
tableview_header_title ()
Sets the text of a column header.
void tableview_header_title(TableView *view, const uint32_t column_id, const char_t *text);
view | The table. |
column_id | The column id. |
text | The text in UTF-8 or the identifier of the resource. Resources. |
Remarks
See Configure columns.
tableview_header_align ()
Sets the alignment of the header text.
void tableview_header_align(TableView *view, const uint32_t column_id, const align_t align);
view | The table. |
column_id | The column id. |
align | The alignment. |
Remarks
See Configure columns.
tableview_header_visible ()
Sets whether the table header is visible or not.
void tableview_header_visible(TableView *view, const bool_t visible);
view | The table. |
visible |
|
Remarks
See Table appearance.
tableview_header_clickable ()
Sets whether the table header can be clicked as a button.
void tableview_header_clickable(TableView *view, const bool_t clickable);
view | The table. |
clickable |
|
Remarks
tableview_header_resizable ()
Sets whether the header allows column resizing.
void tableview_header_resizable(TableView *view, const bool_t resizable);
view | The table. |
resizable |
|
Remarks
See Configure columns.
tableview_header_height ()
Force the height of the header.
void tableview_header_height(TableView *view, const real32_t height);
view | The table. |
height | The height of the header. |
Remarks
The height of the header is automatically calculated from the content. Forcing this value may cause the table to not display correctly. Its use is not recommended. See Table appearance.
tableview_row_height ()
Force the height of the row.
void tableview_row_height(TableView *view, const real32_t height);
view | The table. |
height | The height of the row. |
Remarks
The row height is automatically calculated from the content. Forcing this value may cause the table to not display correctly. its use is not recommended. See Table appearance.
tableview_hkey_scroll ()
Sets the horizontal scrolling when pressing the [LEFT]
and [RIGHT]
keys.
void tableview_hkey_scroll(TableView *view, const bool_t force_column, const real32_t scroll);
view | The table. |
force_column | If |
scroll | If |
Remarks
See Table navigation.
tableview_multisel ()
Sets the row selection mode.
void tableview_multisel(TableView *view, const bool_t multisel, const bool_t preserve);
view | The table. |
multisel |
|
preserve |
|
Remarks
See Multiple selection.
tableview_grid ()
Sets the drawing of the interior lines.
void tableview_grid(TableView *view, const bool_t hlines, const bool_t vlines);
view | The table. |
hlines |
|
vlines |
|
Remarks
See Table appearance.
tableview_update ()
Synchronizes the table with the data source.
void tableview_update(TableView *view);
view | The table. |
Remarks
See Data connection. We must call this function from the application whenever the data linked to the table changes, in order to update the view.
tableview_select ()
Selects rows in the table.
void tableview_select(TableView *view, const uint32_t *rows, const uint32_t n);
view | The table. |
rows | Vector of line indices. |
n | Number of elements in the vector. |
Remarks
See Multiple selection.
tableview_deselect ()
Deselects rows in the table.
void tableview_deselect(TableView *view, const uint32_t *rows, const uint32_t n);
view | The table. |
rows | Vector of line indices. |
n | Number of elements in the vector. |
Remarks
See Multiple selection.
tableview_deselect_all ()
Deselects all rows in the table.
void tableview_deselect_all(TableView *view);
view | The table. |
Remarks
See Multiple selection.
tableview_selected ()
Returns the currently selected rows.
const ArrSt(uint32_t)* tableview_selected(const TableView *view);
view | The table. |
Return
Array with the indices of the selected rows.
Remarks
See Multiple selection.
tableview_focus_row ()
Set keyboard focus to a specific row.
void tableview_focus_row(TableView *view, const uint32_t row, const align_t align);
view | The table. |
row | The row that will receive focus. |
align | Vertical adjustment. |
Remarks
Setting keyboard focus to a row only has effects on navigation, but does not involve selecting the row. The table is automatically scrolled so that the row is visible. In this case, align
indicates where the vertical scroll is adjusted (up, down or centered). See Table navigation.
tableview_get_focus_row ()
Gets the row that has keyboard focus.
uint32_t tableview_get_focus_row(const TableView *view);
view | The table. |
Return
The row that has the focus.
Remarks
See Table navigation.
tableview_scroll_visible ()
Show or hide scroll bars.
void tableview_scroll_visible(TableView *view, const bool_t horizontal, const bool_t vertical);
view | The table. |
horizontal | Horizontal bar. |
vertical | Vertical bar. |
Remarks
See Table appearance.