Edit
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
Edit* | edit_create (void) |
Edit* | edit_multiline (void) |
void | edit_OnFilter (...) |
void | edit_OnChange (...) |
void | edit_OnFocus (...) |
void | edit_text (...) |
void | edit_font (...) |
void | edit_align (...) |
void | edit_passmode (...) |
void | edit_editable (...) |
void | edit_autoselect (...) |
void | edit_select (...) |
void | edit_tooltip (...) |
void | edit_color (...) |
void | edit_color_focus (...) |
void | edit_bgcolor (...) |
void | edit_bgcolor_focus (...) |
void | edit_phtext (...) |
void | edit_phcolor (...) |
void | edit_phstyle (...) |
void | edit_vpadding (...) |
const char_t* | edit_get_text (...) |
real32_t | edit_get_height (...) |
void | edit_copy (...) |
void | edit_cut (...) |
void | edit_paste (...) |
EditBox are small text boxes with editing capabilities. Like the Label they are of uniform format: The typeface and colors will affect the entire text (Figure 1). They are usually used to edit fields in forms, normally restricted to a single line, although they can also be extended to several of them. To edit texts with multiple attributes use TextView. In Hello Edit and UpDown! you have an example of use.
- Use edit_create to create an edit box.
- Use edit_multiline to create a multi-line editing box.
- Use edit_passmode to hide the text of the control.
- Use edit_phtext to set a placeholder.
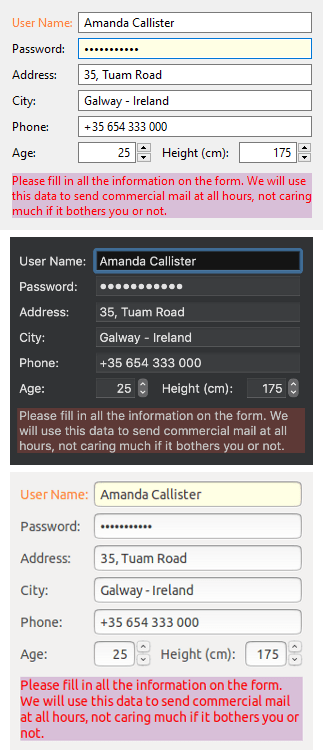
1. Validate texts
- Use edit_OnChange to validate the text.
Depending on the value we are editing, it may be necessary to validate the entered text. Just before losing keyboard focus, the control will receive an OnChange
event, for which we can provide a specialized handler (Listing 1) (Figure 2). If the text is not valid, said handler must return FALSE
, thus preventing the focus from changing to the next control, remaining in the editbox and forcing the user to correct it.
1 2 3 4 5 6 7 8 9 10 11 12 |
static void i_OnChange(App *app, Event *e) { const EvText *p = event_params(e, EvText); if (is_valid_text(data, p->text) == FALSE) { // Force the focus remain in editbox bool_t *r = event_result(e, bool_t); *r = FALSE; } } ... edit_OnChange(edit, listener(NULL, i_OnChange, void)); |
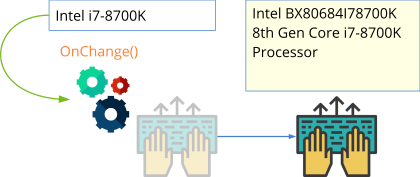
For example, in Hello Edit and UpDown! activating the Field validations check will show a modal window within the OnChange event allowing you to validate or reject the text (Figure 3).
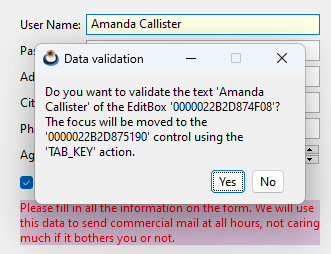
It will not be possible to move keyboard focus to another control while the text is invalid.
2. Filter texts
- Use edit_OnFilter to detect and correct each user keystroke.
In case we want to implement more elaborate filters, which correct the text while it is written, we will use the edit_OnFilter
event (Figure 4). We have an example in Hello TextSel and Clipboard!. We will receive, through the EvText structure, a copy of the current text (EvText::text
), the position of the cursor (EvText::cpos
) and the number of characters added or removed (EvText::len
). From here, if the text must be modified, we must obtain the result structure EvTextFilter, setting its apply
field to TRUE
. In EvTextFilter::text
we must copy the new text and, in EvTextFilter::cpos
, the new position of the cursor. For example, in (Listing 2) only the inserted characters are converted to uppercase, leaving the rest of the text intact.
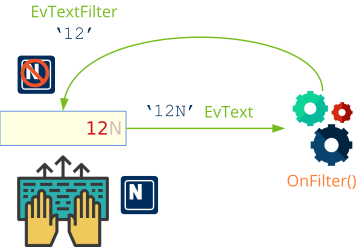
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
static void i_OnFilter(SelData *data, Event *e) { const EvText *p = event_params(e, EvText); cassert_no_null(data); /* * Convert the inserted text in caps. * p->text the control current text (const). * r->text the new filterd text. * p->cpos current caret position. * r->apply = TRUE means the editbox text has to be updated. * p->len number of chars inserted at left of caret (to caps). * We are working with UTF8-Strings. Sequential access. */ if (button_get_state(data->caps) == ekGUI_ON && p->len > 0) { EvTextFilter *r = event_result(e, EvTextFilter); const char_t *src = p->text; char_t *dest = r->text; uint32_t cp = unicode_to_u32(src, ekUTF8); uint32_t pos = 0; while (cp != 0) { uint32_t offset = 0; if (pos >= p->cpos - p->len && pos < p->cpos) { if (cp >= 'a' && cp <= 'z') cp -= 32; } offset = unicode_to_char(cp, dest, ekUTF8); dest += offset; src = unicode_next(src, ekUTF8); cp = unicode_to_u32(src, ekUTF8); pos += 1; } *dest = 0; r->cpos = p->cpos; r->apply = TRUE; } textview_printf(data->info_text, "Edit: Pos %d Len %d\n", p->cpos, p->len); textview_scroll_caret(data->info_text); } ... edit_OnFilter(edit, listener(NULL, i_OnFilter, void)); |
3. Text selection
- Use edit_select to select text.
- Use edit_autoselect to automatically select all text whenever the control receives keyboard focus.
It is possible through code to change the text selection (Figure 5) and the position of the cursor (caret), using this logic.
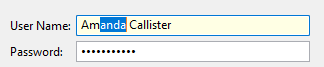
- If
start == -1
andend == 0
, all text is deselected, leaving the caret in its current position. - If
start == -1
andend == -1
, all text is deselected, moving the caret to the end of the text. - If
start == 0
andend == -1
all the text is selected, moving the caret to the end of the text. - If
start > 0
andend == -1
is selected until the end, moving the caret to the end of the text. - If
start == end
the caret is moved to the position, deselecting all text.
4. Clipboard operations
As they are native components, the Edit
controls support typical clipboard operations: Copy, Paste, Cut, etc., as well as their keyboard shortcuts. However, it can be useful to access these operations from the program code, allowing, for example, the text selected in the control to be copied to the clipboard.
- Use edit_copy to copy the selected text to the clipboard.
- Use edit_cut to cut the selected text, copying it to the clipboard.
- Use edit_paste to paste the clipboard text at the caret position.
edit_create ()
Create a text edit control.
Edit* edit_create(void);
Return
The edit.
edit_multiline ()
Create a text editing control that allows multiple lines.
Edit* edit_multiline(void);
Return
The edit.
edit_OnFilter ()
Set a function to filter the text while editing.
void edit_OnFilter(Edit *edit, Listener *listener);
edit | The edit. |
listener | Callback function to be called after each key press. In EvTextFilter from event_result filtered text will be returned. |
Remarks
See Filter texts and GUI Events.
edit_OnChange ()
Set a function to detect when the text has changed.
void edit_OnChange(Edit *edit, Listener *listener);
edit | The edit. |
listener | Callback function to be called when the control loses focus on the keyboard, indicating the end of the edition. |
Remarks
See Validate texts and GUI Events.
edit_OnFocus ()
Sets a handler for keyboard focus.
void edit_OnFocus(Edit *edit, Listener *listener);
edit | The edit. |
listener | Callback function that will be called when keyboard focus is received or lost. |
Remarks
See GUI Events.
edit_text ()
Set the edit control text.
void edit_text(Edit *edit, const char_t *text);
edit | The edit. |
text | UTF8 C-string terminated in null character |
edit_font ()
Set the font of the edit control.
void edit_font(Edit *edit, const Font *font);
edit | The edit. |
font | Font. |
edit_align ()
Set text alignment.
void edit_align(Edit *edit, const align_t align);
edit | The edit. |
align | Alignment. |
edit_passmode ()
Activate the password mode, which will hide the typed characters.
void edit_passmode(Edit *edit, const bool_t passmode);
edit | The edit. |
passmode | Enable or disable password mode. |
edit_editable ()
Enable or disable editing in the control.
void edit_editable(Edit *edit, const bool_t is_editable);
edit | The edit. |
is_editable |
|
edit_autoselect ()
Activate or deactivate auto-selection of text.
void edit_autoselect(Edit *edit, const bool_t autoselect);
edit | The edit. |
autoselect |
|
Remarks
See Text selection.
edit_select ()
Select text.
void edit_select(Edit *edit, const int32_t start, const int32_t end);
edit | The edit. |
start | Position of the initial character. |
end | Position of the final character. |
Remarks
See Text selection.
edit_tooltip ()
Assigns a tooltip to the edit control.
void edit_tooltip(Edit *edit, const char_t *text);
edit | The edit. |
text | UTF8 C-string terminated in null character |
edit_color ()
Set the text color.
void edit_color(Edit *edit, const color_t color);
edit | The edit. |
color | Text color. |
Remarks
RGB values may not be fully portable. See Colors.
edit_color_focus ()
Sets the color of the text, when the control has the keyboard focus.
void edit_color_focus(Edit *edit, const color_t color);
edit | The edit. |
color | Text color. |
Remarks
RGB values may not be fully portable. See Colors.
edit_bgcolor ()
Set the background color.
void edit_bgcolor(Edit *edit, const color_t color);
edit | The edit. |
color | Background color. |
Remarks
RGB values may not be fully portable. See Colors.
edit_bgcolor_focus ()
Sets the background color, when the control has keyboard focus.
void edit_bgcolor_focus(Edit *edit, const color_t color);
edit | The edit. |
color | Background color. |
Remarks
RGB values may not be fully portable. See Colors.
edit_phtext ()
Set an explanatory text for when the control is blank (placeholder).
void edit_phtext(Edit *edit, const char_t *text);
edit | The edit. |
text | UTF8 C-string terminated in null character |
edit_phcolor ()
Set the color of the placeholder text.
void edit_phcolor(Edit *edit, const color_t color);
edit | The edit. |
color | Text color. |
Remarks
RGB values may not be fully portable. See Colors.
edit_phstyle ()
Set the font style for the placeholder.
void edit_phstyle(Edit *edit, const uint32_t fstyle);
edit | The edit. |
fstyle | Combination of values of fstyle_t. |
edit_vpadding ()
Sets the inner vertical margin.
void edit_vpadding(Edit *edit, const real32_t padding);
edit | The edit. |
padding | If |
edit_get_text ()
Get control text.
const char_t* edit_get_text(const Edit *edit);
edit | The edit. |
Return
UTF8 C-string terminated in null character '\0'
.
edit_get_height ()
Gets the current height of the control.
real32_t edit_get_height(const Edit *edit);
edit | The edit. |
Return
The height of the control, which will change depending on the font size and vpadding
.
edit_copy ()
Copies the selected text to the clipboard.
void edit_copy(const Edit *edit);
edit | The edit. |
Remarks
See Clipboard operations.
edit_cut ()
Cuts the selected text, copying it to the clipboard.
void edit_cut(Edit *edit);
edit | The edit. |
Remarks
See Clipboard operations.
edit_paste ()
Pastes the text from the clipboard into the caret position.
void edit_paste(Edit *edit);
edit | The edit. |
Remarks
See Clipboard operations.