Pixel Buffer
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
Pixbuf* | pixbuf_create (...) |
Pixbuf* | pixbuf_copy (...) |
Pixbuf* | pixbuf_trim (...) |
Pixbuf* | pixbuf_convert (...) |
void | pixbuf_destroy (...) |
pixformat_t | pixbuf_format (...) |
uint32_t | pixbuf_width (...) |
uint32_t | pixbuf_height (...) |
uint32_t | pixbuf_size (...) |
uint32_t | pixbuf_dsize (...) |
const byte_t* | pixbuf_cdata (...) |
byte_t* | pixbuf_data (...) |
uint32_t | pixbuf_format_bpp (...) |
uint32_t | pixbuf_get (...) |
void | pixbuf_set (...) |
A pixel buffer (Pixbuf
) is a memory area that represents a grid of color dots or pixels. They allow direct access to information but are not optimized for drawing on the screen, so we must create an Image object to view them. They are very efficient for procedural generation or the application of filters, since reading or writing a value does not require more than accessing its position within the buffer.
- Use pixbuf_create to create a new pixel buffer.
- Use image_pixels to get the pixels of an image.
- Use pixbuf_width to get the width of the grid.
- Use pixbuf_height to get the height of the grid.
All operations on pixel buffers are performed on the CPU. They are efficient to the extent that we directly access memory, but they cannot be compared with alternatives that use the GPU for digital image processing.
1. Pixel formats
The format refers to how the value of each pixel is encoded within the buffer (Table 1) (Figure 1).
- Use pixbuf_format to get the pixel format.
- Use pixbuf_format_bpp to get the number of bits wanted for each pixel.
Value | Description |
ekRGB24 | True color +16 million simultaneous, 24 bits per pixel. |
ekRGBA32 | True color with alpha channel (transparencies), 32 bits per pixel. |
ekGRAY8 | 256 shades of gray, 8 bits per pixel. |
ekINDEX1 | Indexed, 1 bit per pixel. |
ekINDEX2 | Indexed, 2 bits per pixel. |
ekINDEX4 | Indexed, 4 bits per pixel. |
ekINDEX8 | Indexed, 8 bits per pixel. |
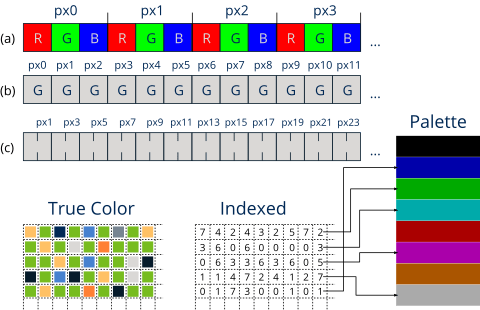
2. Procedural images
One way to "fill" buffers is through algorithms that calculate the value of each pixel. A clear example is found in the representation of fractal sets (Figure 2), an area of mathematics dedicated to the study of certain dynamic systems. In Fractals you have the complete application.
- Use pixbuf_data to get a pointer to the contents of the buffer.
- Use pixbuf_set to write the value of a pixel.
- Use pixbuf_get to read the value of a pixel.
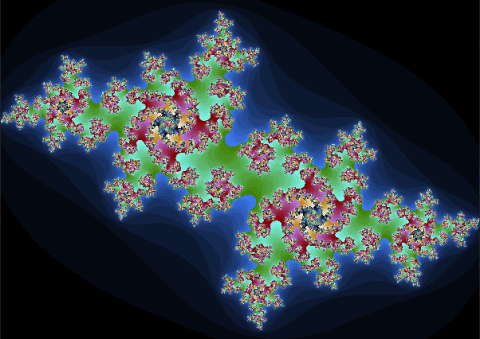
While pixbuf_set
and pixbuf_get
allow safe pixel manipulation, it may sometimes be necessary to get a little extra in terms of performance. In (Listing 1) we have some macros for direct access to the memory area returned by pixbuf_data. Use them with great care and knowing what you are doing, since they do not have error control methods, so segmentation failures are likely if they are not used correctly.
ekINDEX1
(1 bit per pixel).
1 2 3 4 5 6 7 8 9 10 |
3. Copy and conversion
During the digital processing of an image, we may have to chain several operations, so it will be useful to be able to make copies of the buffers or format conversions.
- Use pixbuf_copy to make a copy.
- Use pixbuf_convert to convert to another format (Table 2).
Source | Destiny | Observations |
RGB24 | RGB32 | Alpha channel is added with the value 255 |
RGB32 | RGB24 | Alpha channel is removed with possible loss of information. |
RGB(A) | Gray | RGB channels are weighted at a ratio of 77/255, 148/255, 30/255. Alpha channel is lost. |
Gray | RGB(A) | RGB channels (gray, gray, gray) are duplicated. Alpha channel to 255. |
RGB(A) | Indexed | The smallest distance between each pixel and the palette is calculated. Possible loss of information. |
Indexed | RGB(A) | The palette will be used to obtain each RGBA value. |
Indexed | Indexed | If the destination has a lower number of bits, out = in % bpp will be applied with possible loss of information. |
Gray | Indexed | The Gray8 format will be considered indexed for all purposes. |
Indexed | Gray | The Gray8 format will be considered indexed for all purposes. |
pixbuf_create ()
Create a new pixel buffer.
Pixbuf* pixbuf_create(const uint32_t width, const uint32_t height, const pixformat_t format);
width | Width. |
height | Height. |
format | Pixel format. |
Return
The buffer pixel.
Remarks
Initial content will be undefined.
pixbuf_copy ()
Create a copy of the pixel buffer.
Pixbuf* pixbuf_copy(const Pixbuf *pixbuf);
pixbuf | The original buffer. |
Return
The copy.
pixbuf_trim ()
Crop a buffer pixel.
Pixbuf* pixbuf_trim(const Pixbuf *pixbuf, const uint32_t x, const uint32_t y, const uint32_t width, const uint32_t height);
pixbuf | The original buffer. |
x | X coordinate of the upper-left pixel. |
y | Y coordinate of the upper-left pixel. |
width | Number of pixels wide. |
height | Number of pixels high. |
Return
A new buffer pixel with clipping.
Remarks
The function does not check that the limits are valid. You will get a segmentation error in such cases.
pixbuf_convert ()
Change the format of a buffer pixel.
Pixbuf* pixbuf_convert(const Pixbuf *pixbuf, const Palette *palette, const pixformat_t oformat);
pixbuf | The original buffer. |
palette | Color palette required for certain conversions. |
oformat | Result buffer format. |
Return
The converted buffer.
Remarks
See Copy and conversion.
pixbuf_destroy ()
Destroy the buffer.
void pixbuf_destroy(Pixbuf **pixbuf);
pixbuf | The buffer. It will be set to |
pixbuf_format ()
Get the pixel format.
pixformat_t pixbuf_format(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
The format.
Remarks
See Pixel formats.
pixbuf_width ()
Get the width of the buffer.
uint32_t pixbuf_width(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Width.
pixbuf_height ()
Get the height of the buffer.
uint32_t pixbuf_height(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Height.
pixbuf_size ()
Get the buffer size (in pixels).
uint32_t pixbuf_size(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Width x height.
pixbuf_dsize ()
Gets the buffer size (in bytes).
uint32_t pixbuf_dsize(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Number of total bytes in the buffer.
pixbuf_cdata ()
Gets a read-only pointer to the contents of the buffer.
const byte_t* pixbuf_cdata(const Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Pointer to the first element.
Remarks
Correctly manipulating the buffer requires knowing the Pixel formats and sometimes using the operators at the bit level. Use pixbuf_get to correctly read a pixel.
pixbuf_data ()
Gets a read/write pointer to the contents of the buffer.
byte_t* pixbuf_data(Pixbuf *pixbuf);
pixbuf | The buffer. |
Return
Pointer to the first element.
Remarks
Correctly manipulating the buffer requires knowing the Pixel formats and sometimes using the operators at the bit level. Use pixbuf_get to correctly read a pixel.
pixbuf_format_bpp ()
Gets bits per pixel based on format.
uint32_t pixbuf_format_bpp(const pixformat_t format);
format | The format. |
Return
Bits per pixel.
Remarks
See Pixel formats.
pixbuf_get ()
Get the value of a pixel.
uint32_t pixbuf_get(const Pixbuf *pixbuf, const uint32_t x, const uint32_t y);
pixbuf | The buffer. |
x | Pixel x-coordinate. |
y | Pixel y coordinate. |
Return
The color value.
Remarks
See Pixel formats to correctly interpret the value.
pixbuf_set ()
Sets the value of a pixel.
void pixbuf_set(Pixbuf *pixbuf, const uint32_t x, const uint32_t y, const uint32_t value);
pixbuf | The buffer. |
x | Pixel x-coordinate. |
y | Pixel y coordinate. |
value | The color value. |
Remarks
See Pixel formats to correctly interpret the value.