Gui
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
void | gui_start (void) |
void | gui_finish (void) |
void | gui_respack (...) |
void | gui_language (...) |
const char_t* | gui_text (...) |
const Image* | gui_image (...) |
const byte_t* | gui_file (...) |
bool_t | gui_dark_mode (void) |
color_t | gui_alt_color (...) |
color_t | gui_label_color (void) |
color_t | gui_view_color (void) |
color_t | gui_line_color (void) |
color_t | gui_link_color (void) |
color_t | gui_border_color (void) |
S2Df | gui_resolution (void) |
V2Df | gui_mouse_pos (void) |
void | gui_update (void) |
void | gui_OnThemeChanged (...) |
void | gui_update_transitions (...) |
void | gui_OnNotification (...) |
void | gui_OnIdle (...) |
uint32_t | gui_info_window (...) |
type* | evbind_object (...) |
bool_t | evbind_modify (...) |
Types and Constants
enum | gui_orient_t |
enum | gui_state_t |
enum | gui_mouse_t |
enum | gui_cursor_t |
enum | gui_close_t |
enum | gui_scale_t |
enum | gui_scroll_t |
enum | gui_focus_t |
enum | gui_tab_t |
enum | gui_event_t |
enum | window_flag_t |
enum | gui_notif_t |
struct | GuiControl |
struct | Label |
struct | Button |
struct | PopUp |
struct | Edit |
struct | Combo |
struct | ListBox |
struct | UpDown |
struct | Slider |
struct | Progress |
struct | View |
struct | TextView |
struct | ImageView |
struct | TableView |
struct | SplitView |
struct | Layout |
struct | Cell |
struct | Panel |
struct | Window |
struct | Menu |
struct | MenuItem |
struct | EvButton |
struct | EvSlider |
struct | EvText |
struct | EvTextFilter |
struct | EvDraw |
struct | EvMouse |
struct | EvWheel |
struct | EvKey |
struct | EvPos |
struct | EvSize |
struct | EvWinClose |
struct | EvMenu |
struct | EvScroll |
struct | EvTbPos |
struct | EvTbRow |
struct | EvTbRect |
struct | EvTbSel |
struct | EvTbCell |
struct | FocusInfo |
The Gui library allows you to create graphical user interfaces in a simple and intuitive way. Only available for desktop applications for obvious reasons (Figure 1), unlike the rest of libraries that can also be used in command line applications.
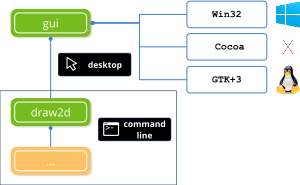
Like Draw2D and Osbs Gui relies on the APIs of each operating system. In addition to the advantages already mentioned in these two cases, native access to interface elements will cause our programs to be fully integrated in the desktop and according to the visual theme present in each machine (Figure 2).
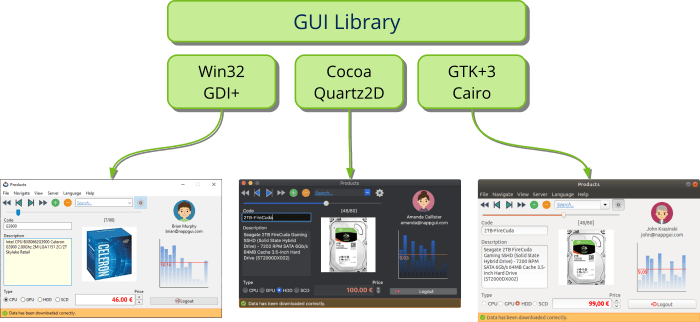
1. Declarative composition
The Gui library moves away from the concept of treating windows (or dialog boxes) as an external resource of the program. On the contrary, these are created directly from the source code avoiding layout by visual editors (Figure 3). We must bear in mind that window managers use different fonts and templates, so specifying specific positions and sizes for the elements will not be portable between platforms (Figure 4). On the contrary, in Gui the controls are located in a virtual grid called Layout, which will calculate its location and final size at runtime and depending on the platform (Figure 5).
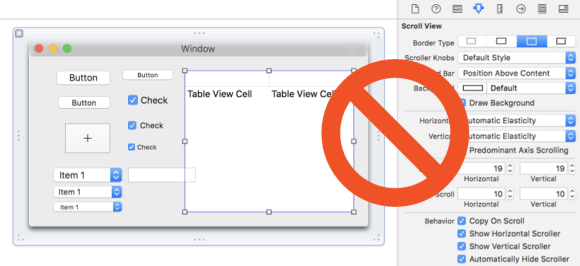
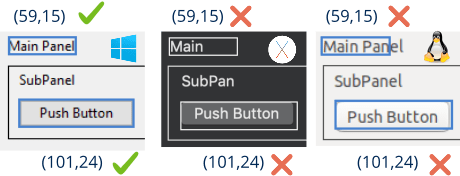
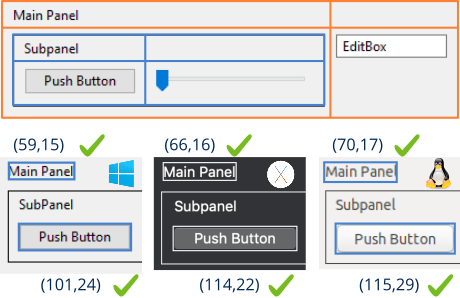
In addition, another relevant fact is that interfaces are living objects subject to constant changes. A clear example is the translations, which alter the location of the elements due to the new dimension of the text (Figure 6). Gui will adapt to these events automatically, recalculating positions to maintain a consistent layout.
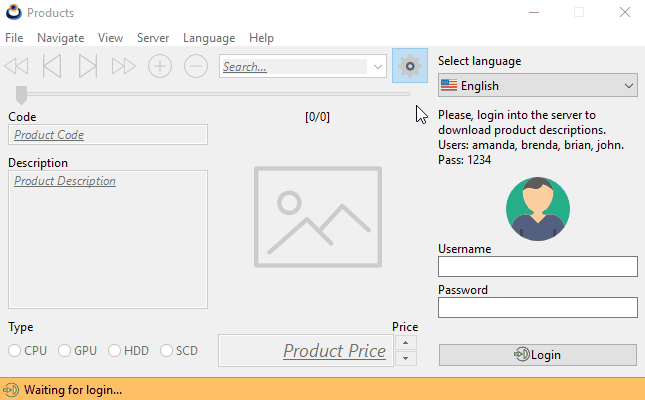
2. Anatomy of a window.
In (Figure 8) we have the main parts of a window. Controls are the final elements with which the user interacts to enter data or launch actions. The views are rectangular regions of relatively large size where information is represented by text and graphics, being able to respond to keyboard or mouse events. Finally, all these elements will be grouped into panels and will be layout by layouts.
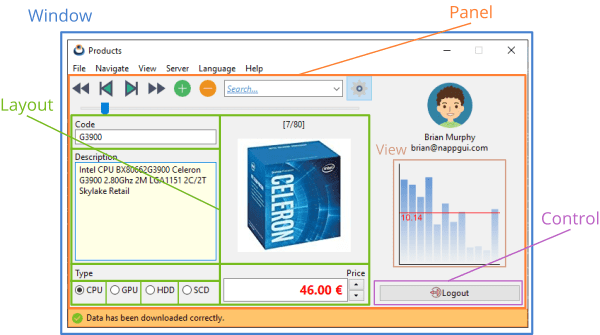
- GuiControl. Different types of controls and views.
- Layout. Virtual and invisible grid where the controls will be located.
- Window. Main window with title bar and frame.
- Menu. Drop-down list with options.
- MenuItem. Each of the menu items.
3. GUI Events
Desktop applications are event driven, which means that they are continually waiting for the user to perform some action on the interface: Press a button, drag a slider, write a text, etc. When this occurs, the window manager detects the event and notifies the application (Figure 9), which must provide an event handler with the code to execute. For example in (Listing 1) we define a handler to respond to the press of a button. Obviously, if there is no associated handler, the application will ignore the event.
- Use event_params to obtain the parameters associated with the event. Each type of event has its own parameters. See (Table 1).
- Use event_result to write the response to the event. Very few events require sending a response.
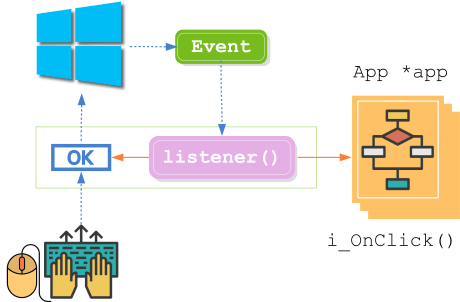
1 2 3 4 5 6 7 8 9 |
static void i_OnClick(App *app, Event *e) { const EvButton *p = event_params(e, EvButton); if (p->state == ekGUI_ON) create_new_file(app); } Button *button = button_check(); button_OnClick(button, listener(app, i_OnClick, App)); |
Sometimes it may be necessary to fire an event while the application is in "standby mode", without processing any other pending events. This occurs when we want to launch a secondary or modal window as a consequence of another event, for example, the pressing of a button. It is advisable, to avoid blocking or unwanted artifacts, to let the first event conclude and schedule the response for a later time, where the application has no pending tasks.
- Use gui_OnIdle to raise an event when there are no other pending tasks.
1 2 3 4 5 6 7 8 9 10 11 |
static void i_OnIdle(App *app, Event *e) { window_modal(app->modal_window, app->main_window); } static void i_OnClick(App *app, Event *e) { // The modal window will be launched after // the OnClick event is totally processed. gui_OnIdle(listener(app, i_OnIdle, App)); } |
Event | Handler | Parameters | Response |
Click in label | label_OnClick | EvText | - |
Click on button | button_OnClick | EvButton | - |
Selection in PopUp | popup_OnSelect | EvButton | - |
A mouse button was pressed | listbox_OnDown | EvMouse | bool_t |
Selection in ListBox | listbox_OnSelect | EvButton | - |
Press key on Edit | edit_OnFilter | EvText | EvTextFilter |
End of edit in Edit | edit_OnChange | EvText | bool_t |
Edit has received or lost keyboard focus | edit_OnFocus | bool_t | - |
Key press on Combo | combo_OnFilter | EvText | EvTextFilter |
End of editing in Combo | combo_OnChange | EvText | bool_t |
Slider movement | slider_OnMoved | EvSlider | - |
Click on UpDown | updown_OnClick | EvButton | - |
Draw the contents of a view | view_OnDraw | EvDraw | - |
Draw the overlay of a view | view_OnOverlay | EvDraw | - |
The size of a view has changed | view_OnSize | EvSize | - |
The mouse enters the area of a view | view_OnEnter | EvMouse | - |
The mouse leaves the area of a view | view_OnExit | - | - |
The mouse moves over a view | view_OnMove | EvMouse | - |
A mouse button was pressed | view_OnDown | EvMouse | - |
A mouse button has been released | view_OnUp | EvMouse | - |
Click on a view | view_OnClick | EvMouse | - |
Dragging on a view | view_OnDrag | EvMouse | - |
Mouse wheel on a view | view_OnWheel | EvWheel | - |
Press key on a view | view_OnKeyDown | EvKey | - |
Release key on a view | view_OnKeyUp | EvKey | - |
View has received or lost keyboard focus | view_OnFocus | bool_t | - |
View resing keyboard focus | view_OnResignFocus | - | bool_t |
View accepts keyboard focus | view_OnAcceptFocus | - | bool_t |
The scroll bars are being manipulated. | view_OnScroll | EvScroll | real32_t |
Keystroke in TextView | textview_OnFilter | EvText | EvTextFilter |
TextView has received or lost keyboard focus | textview_OnFocus | bool_t | - |
Close a window | window_OnClose | EvWinClose | bool_t |
Window moving around the desk | window_OnMoved | EvPos | - |
Window is re-dimensioning | window_OnResize | EvSize | - |
Click on an item menu | menuitem_OnClick | EvMenu | - |
Color change | comwin_color | color_t | - |
Inactivity | gui_OnIdle | - | - |
enum gui_orient_t
Orientation.
enum gui_orient_t
{
ekGUI_HORIZONTAL,
ekGUI_VERTICAL
};
ekGUI_HORIZONTAL | Horizontal. |
ekGUI_VERTICAL | Vertical. |
enum gui_state_t
State values.
enum gui_state_t
{
ekGUI_OFF,
ekGUI_ON,
ekGUI_MIXED
};
ekGUI_OFF | Off. |
ekGUI_ON | On. |
ekGUI_MIXED | Medium/undetermined. |
enum gui_mouse_t
Mouse buttons.
enum gui_mouse_t
{
ekGUI_MOUSE_LEFT,
ekGUI_MOUSE_RIGHT,
ekGUI_MOUSE_MIDDLE
};
ekGUI_MOUSE_LEFT | Left. |
ekGUI_MOUSE_RIGHT | Right. |
ekGUI_MOUSE_MIDDLE | Center. |
enum gui_cursor_t
Cursors. See window_cursor.
enum gui_cursor_t
{
ekGUI_CURSOR_ARROW,
ekGUI_CURSOR_HAND,
ekGUI_CURSOR_IBEAM,
ekGUI_CURSOR_CROSS,
ekGUI_CURSOR_SIZEWE,
ekGUI_CURSOR_SIZENS,
ekGUI_CURSOR_USER
};
ekGUI_CURSOR_ARROW | Arrow (default). |
ekGUI_CURSOR_HAND | Hand. |
ekGUI_CURSOR_IBEAM | Vertical bar (text editing). |
ekGUI_CURSOR_CROSS | Cross. |
ekGUI_CURSOR_SIZEWE | Horizontal resize (left-right). |
ekGUI_CURSOR_SIZENS | Vertical resize (top-bottom). |
ekGUI_CURSOR_USER | Created from an image. |
enum gui_close_t
Reason for closing a window.
enum gui_close_t
{
ekGUI_CLOSE_ESC,
ekGUI_CLOSE_INTRO,
ekGUI_CLOSE_BUTTON,
ekGUI_CLOSE_DEACT
};
ekGUI_CLOSE_ESC | The |
ekGUI_CLOSE_INTRO | The |
ekGUI_CLOSE_BUTTON | The close button |
ekGUI_CLOSE_DEACT | The main window has been clicked (only received by overlay windows). |
enum gui_scale_t
Scaling modes.
enum gui_scale_t
{
ekGUI_SCALE_AUTO,
ekGUI_SCALE_NONE,
ekGUI_SCALE_ASPECT,
ekGUI_SCALE_ASPECTDW
};
ekGUI_SCALE_AUTO | Automatic scaling, the proportion may change. |
ekGUI_SCALE_NONE | No scaling. |
ekGUI_SCALE_ASPECT | Automatic scaling, but maintaining the proportion (aspect ratio). |
ekGUI_SCALE_ASPECTDW | Same as above, but does not increase the original size, only reduce it if appropriate. |
enum gui_scroll_t
Types of scroll.
enum gui_scroll_t
{
ekGUI_SCROLL_BEGIN,
ekGUI_SCROLL_END,
ekGUI_SCROLL_STEP_LEFT,
ekGUI_SCROLL_STEP_RIGHT,
ekGUI_SCROLL_PAGE_LEFT,
ekGUI_SCROLL_PAGE_RIGHT,
ekGUI_SCROLL_THUMB
};
ekGUI_SCROLL_BEGIN | Jump to start. |
ekGUI_SCROLL_END | Skip to the end. |
ekGUI_SCROLL_STEP_LEFT | Jump one step (or line) to the left or up. |
ekGUI_SCROLL_STEP_RIGHT | Jump one step (or line) to the right or down. |
ekGUI_SCROLL_PAGE_LEFT | Jump a page to the left or up. |
ekGUI_SCROLL_PAGE_RIGHT | Jump a page to the right or down. |
ekGUI_SCROLL_THUMB | Jump to the |
enum gui_focus_t
Result when changing the keyboard focus.
enum gui_focus_t
{
ekGUI_FOCUS_CHANGED,
ekGUI_FOCUS_KEEP,
ekGUI_FOCUS_NO_NEXT,
ekGUI_FOCUS_NO_RESIGN,
ekGUI_FOCUS_NO_ACCEPT
};
ekGUI_FOCUS_CHANGED | The focus has changed to the specified control. |
ekGUI_FOCUS_KEEP | The focus has not moved, it remains on the same control. |
ekGUI_FOCUS_NO_NEXT | Target control not found, hidden, or disabled. |
ekGUI_FOCUS_NO_RESIGN | The current control does not allow changing focus. |
ekGUI_FOCUS_NO_ACCEPT | The new control does not accept focus. |
enum gui_tab_t
Action that has motivated the change of keyboard focus.
enum gui_tab_t
{
ekGUI_TAB_KEY,
ekGUI_TAB_BACKKEY,
ekGUI_TAB_NEXT,
ekGUI_TAB_PREV,
ekGUI_TAB_MOVE,
ekGUI_TAB_CLICK
};
ekGUI_TAB_KEY | Pressing the |
ekGUI_TAB_BACKKEY | Pressing |
ekGUI_TAB_NEXT | Call to window_next_tabstop. |
ekGUI_TAB_PREV | Call to window_previous_tabstop. |
ekGUI_TAB_MOVE | Call to window_focus. |
ekGUI_TAB_CLICK | Click on another control. |
enum gui_event_t
Event type. See GUI Events.
enum gui_event_t
{
ekGUI_EVENT_LABEL,
ekGUI_EVENT_BUTTON,
ekGUI_EVENT_POPUP,
ekGUI_EVENT_LISTBOX,
ekGUI_EVENT_SLIDER,
ekGUI_EVENT_UPDOWN,
ekGUI_EVENT_TXTFILTER,
ekGUI_EVENT_TXTCHANGE,
ekGUI_EVENT_FOCUS_RESIGN,
ekGUI_EVENT_FOCUS_ACCEPT,
ekGUI_EVENT_FOCUS,
ekGUI_EVENT_MENU,
ekGUI_EVENT_DRAW,
ekGUI_EVENT_OVERLAY,
ekGUI_EVENT_RESIZE,
ekGUI_EVENT_ENTER,
ekGUI_EVENT_EXIT,
ekGUI_EVENT_MOVED,
ekGUI_EVENT_DOWN,
ekGUI_EVENT_UP,
ekGUI_EVENT_CLICK,
ekGUI_EVENT_DRAG,
ekGUI_EVENT_WHEEL,
ekGUI_EVENT_KEYDOWN,
ekGUI_EVENT_KEYUP,
ekGUI_EVENT_SCROLL,
ekGUI_EVENT_WND_MOVED,
ekGUI_EVENT_WND_SIZING,
ekGUI_EVENT_WND_SIZE,
ekGUI_EVENT_WND_CLOSE,
ekGUI_EVENT_COLOR,
ekGUI_EVENT_THEME,
ekGUI_EVENT_OBJCHANGE,
ekGUI_EVENT_TBL_NROWS,
ekGUI_EVENT_TBL_BEGIN,
ekGUI_EVENT_TBL_END,
ekGUI_EVENT_TBL_CELL,
ekGUI_EVENT_TBL_SEL,
ekGUI_EVENT_TBL_HEADCLICK,
ekGUI_EVENT_TBL_ROWCLICK
};
ekGUI_EVENT_LABEL | Click on a Label control. |
ekGUI_EVENT_BUTTON | Click on a Button control. |
ekGUI_EVENT_POPUP | The selection of a PopUp control has been changed. |
ekGUI_EVENT_LISTBOX | The selection of a control has been changed ListBox. |
ekGUI_EVENT_SLIDER | You are moving an Slidercontrol. |
ekGUI_EVENT_UPDOWN | Click on a UpDown control. |
ekGUI_EVENT_TXTFILTER | |
ekGUI_EVENT_TXTCHANGE | You have finished editing the text of a Edit or Combo control. |
ekGUI_EVENT_FOCUS_RESIGN | Ask a control if it wants to give up keyboard focus. |
ekGUI_EVENT_FOCUS_ACCEPT | Ask a control if it wants to accept keyboard focus. |
ekGUI_EVENT_FOCUS | A control has received or lost keyboard focus. |
ekGUI_EVENT_MENU | Click on a menu. |
ekGUI_EVENT_DRAW | The view content must be redrawn. |
ekGUI_EVENT_OVERLAY | There is draw the overlay layer. |
ekGUI_EVENT_RESIZE | The size of a view has changed. |
ekGUI_EVENT_ENTER | The mouse has entered the view area. |
ekGUI_EVENT_EXIT | The mouse has left the view area. |
ekGUI_EVENT_MOVED | The mouse is moving on the view surface. |
ekGUI_EVENT_DOWN | A mouse button was pressed. |
ekGUI_EVENT_UP | A mouse button has been released. |
ekGUI_EVENT_CLICK | Click on a view. |
ekGUI_EVENT_DRAG | Dragging is being done over. |
ekGUI_EVENT_WHEEL | Mouse wheel has moved. |
ekGUI_EVENT_KEYDOWN | A key has been pressed. |
ekGUI_EVENT_KEYUP | A key has been released. |
ekGUI_EVENT_SCROLL | The scroll bars are being manipulated. |
ekGUI_EVENT_WND_MOVED | The window is moving across the desktop. |
ekGUI_EVENT_WND_SIZING | The window is being resized. |
ekGUI_EVENT_WND_SIZE | The window has been resized. |
ekGUI_EVENT_WND_CLOSE | The window has been closed. |
ekGUI_EVENT_COLOR | An update color of comwin_color. |
ekGUI_EVENT_THEME | Desktop theme has changed. |
ekGUI_EVENT_OBJCHANGE | An object linked to a layout has been edited. Notifications and calculated fields. |
ekGUI_EVENT_TBL_NROWS | A table needs to know the number of rows. |
ekGUI_EVENT_TBL_BEGIN | A table will begin to draw the visible part of the data. |
ekGUI_EVENT_TBL_END | A table has finished drawing. |
ekGUI_EVENT_TBL_CELL | A table needs the data of a cell. |
ekGUI_EVENT_TBL_SEL | The selected rows in a table have changed. |
ekGUI_EVENT_TBL_HEADCLICK | Click on a table header. |
ekGUI_EVENT_TBL_ROWCLICK | Click on a table row. |
enum window_flag_t
Window creation attributes.
enum window_flag_t
{
ekWINDOW_FLAG,
ekWINDOW_EDGE,
ekWINDOW_TITLE,
ekWINDOW_MAX,
ekWINDOW_MIN,
ekWINDOW_CLOSE,
ekWINDOW_RESIZE,
ekWINDOW_RETURN,
ekWINDOW_ESC,
ekWINDOW_MODAL_NOHIDE,
ekWINDOW_STD,
ekWINDOW_STDRES
};
ekWINDOW_FLAG | Default attributes. |
ekWINDOW_EDGE | The window draws an outer border. |
ekWINDOW_TITLE | The window has a title bar. |
ekWINDOW_MAX | The window shows the maximize button. |
ekWINDOW_MIN | The window shows the minimize button. |
ekWINDOW_CLOSE | The window shows the close button. |
ekWINDOW_RESIZE | The window has resizable borders. |
ekWINDOW_RETURN | The window will process the pressing of the [RETURN] key as a possible closing event, sending the message OnClose. |
ekWINDOW_ESC | The window will process the pressing of the [ESC] key as a possible closing event, sending the message OnClose. |
ekWINDOW_MODAL_NOHIDE | Avoids hiding a modal window when the modal cycle has finished. See Modal windows. |
ekWINDOW_STD | Combination |
ekWINDOW_STDRES | Combination |
enum gui_notif_t
Notifications sent by the gui
library.
enum gui_notif_t
{
ekGUI_NOTIF_LANGUAGE,
ekGUI_NOTIF_WIN_DESTROY,
ekGUI_NOTIF_MENU_DESTROY
};
ekGUI_NOTIF_LANGUAGE | The interface language has been changed. |
ekGUI_NOTIF_WIN_DESTROY | A window has been destroyed. |
ekGUI_NOTIF_MENU_DESTROY | A menu has been destroyed. |
struct GuiControl
Interface Control (abstract).
struct GuiControl;
struct Label
Interface control that contains static text, usually limited to a single line. Label.
struct Label;
struct Button
Interface control representing a button. Button.
struct Button;
struct PopUp
Control button with drop-down list. PopUp.
struct PopUp;
struct Edit
Text editing control Edit.
struct Edit;
struct Combo
Control that combines an edit box with a drop-down list. Combo.
struct Combo;
struct ListBox
List control. ListBox.
struct ListBox;
struct UpDown
Control that shows two small increase and decrease buttons. UpDown.
struct UpDown;
struct Slider
Control that shows a bar with a slider. Slider.
struct Slider;
struct Progress
Progress bar. Progress.
struct Progress;
struct View
Custom View that allows to create our own controls, drawing what we want. View
struct View;
struct TextView
Text view with several paragraphs and different attributes. TextView.
struct TextView;
struct ImageView
Image viewer control. ImageView.
struct ImageView;
struct TableView
Table view with multiple rows and columns. TableView.
struct TableView;
struct SplitView
Resizable horizontal or vertical split view. SplitView.
struct SplitView;
struct Layout
Invisible grid where the controls of a Panel are organized. Layout.
struct Layout;
struct Cell
Each of the cells that form a Layout. Cell.
struct Cell;
struct Panel
Internal area of a window, which allows you to group different controls. Panel.
struct Panel;
struct Window
Interface window. Window.
struct Window;
struct Menu
Menu or submenu. Menu.
struct Menu;
struct MenuItem
Item within a menu. MenuItem.
struct MenuItem;
struct EvButton
Parameters of the OnClick event of a button or OnSelect of a popup.
struct EvButton { uint32_t index; gui_state_t state; const char_t* text; };
index | Button or item index. |
state | State. |
text | Text. |
struct EvSlider
Parameters of the OnMoved event of a slider.
struct EvSlider { real32_t pos; real32_t incr; uint32_t step; };
pos | Normalized slider position (0, 1). |
incr | Increase with respect to the previous position. |
step | Interval index (only for discrete ranges). |
struct EvText
Parameters of the OnChange event of the text boxes.
struct EvText { const char_t* text; uint32_t cpos; int32_t len; };
text | Text. |
cpos | Cursor position (caret). |
len | Number of characters inserted or deleted. |
struct EvTextFilter
Result of the OnFilter event of the text boxes.
struct EvTextFilter { bool_t apply; char_t* text; uint32_t cpos; };
apply |
|
text | New control text, which is a revision (filter) of the original text. |
cpos | Cursor position (caret). |
struct EvDraw
OnDraw event parameters.
struct EvDraw { DCtx* ctx; real32_t x; real32_t y; real32_t width; real32_t height; };
ctx | 2D drawing context. |
x | X coordinate of the drawing area (viewport). |
y | Y coordinate of the drawing area. |
width | Width of the drawing area. |
height | Height of the drawing area. |
struct EvMouse
Mouse event parameters.
struct EvMouse { real32_t x; real32_t y; real32_t lx; real32_t ly; gui_mouse_t button; uint32_t count; uint32_t modifiers; uint32_t tag; };
x | X coordinate of the pointer in the drawing area. |
y | Y coordinate of the pointer in the drawing area. |
lx | X coordinate of the pointer on the control. Same as |
ly | Y coordinate of the pointer on the control. Same as |
button | Active button. |
count | Number of clicks. |
modifiers | Combination of values mkey_t. |
tag | Additional value for controls. |
struct EvWheel
OnWheel event parameters.
struct EvWheel { real32_t x; real32_t y; real32_t dx; real32_t dy; real32_t dz; };
x | Pointer x coordinate. |
y | Pointer y coordinate. |
dx | Increase in x of the wheel or trackpad. |
dy | Increase in x of the wheel or trackpad. |
dz | Increase in x of the wheel or trackpad. |
struct EvKey
Keyboard event parameters.
struct EvKey { vkey_t key; uint32_t modifiers; };
key | Referenced key. |
modifiers | Combination of values mkey_t. |
struct EvPos
Parameters of change of position events.
struct EvPos { real32_t x; real32_t y; };
x | X coordinate. |
y | Y coordinate. |
struct EvSize
Resize event parameters.
struct EvSize { real32_t width; real32_t height; };
width | Width (size in x). |
height | Height (size in y). |
struct EvWinClose
Window closing Event Parameters.
struct EvWinClose { gui_close_t origin; };
origin | Origin of the close. |
struct EvMenu
Menu event parameters.
struct EvMenu { uint32_t index; gui_state_t state; const char_t* text; };
index | Pressed item index. |
state | Pressed item status. |
text | Pressed item text. |
struct EvScroll
Scroll event parameters.
struct EvScroll { gui_orient_t orient; gui_orient_t scroll; real32_t cpos; };
orient | Scroll bar orientation. |
scroll | Scroll type. |
cpos | Scroll position. |
struct EvTbPos
Location of a cell in a table.
struct EvTbPos { uint32_t col; uint32_t row; };
col | Column index. |
row | Row index. |
struct EvTbRow
Location of a row in a table.
struct EvTbRow { bool_t sel; uint32_t row; };
sel | Selected or not. |
row | Row index. |
struct EvTbRect
Group of cells in a table.
struct EvTbRect { uint32_t stcol; uint32_t edcol; uint32_t strow; uint32_t edrow; };
stcol | Initial column index. |
edcol | End column index. |
strow | Initial row index. |
edrow | End row index. |
struct EvTbSel
Selection in a table.
struct EvTbSel { ArrSt(uint32_t)* sel; };
sel | Row indices. |
struct EvTbCell
Data from a cell in a table.
struct EvTbCell { const char_t* text; align_t align; };
text | Cell text. |
align | Text alignment. |
struct FocusInfo
Information about changing the keyboard focus.
struct FocusInfo { gui_tab_t action; GuiControl* next; };
action | Action that has motivated the change of keyboard focus. |
next | Next control that will receive keyboard focus. |
gui_start ()
Start the Gui library, reserving space for global internal structures. Internally call draw2d_start. It is called automatically by osmain.
void
gui_start(void);
gui_finish ()
Finish the Gui library, freeing up the space of global internal structures. Internally call draw2d_finish. It is called automatically by osmain.
void
gui_finish(void);
gui_respack ()
Register a resource package.
void gui_respack(FPtr_respack func_respack);
func_respack | Resource constructor. |
Remarks
See Resources.
gui_language ()
Set the language of the registered resources with gui_respack.
void gui_language(const char_t *lang);
lang | Language. |
Remarks
See Resources.
gui_text ()
Get a text string through its resource identifier.
const char_t* gui_text(const ResId id);
id | Resource Identifier. |
Return
The text string or NULL
if it is not found.
Remarks
The resource must belong to a package registered with gui_respack.
gui_image ()
Get an image through its resource identifier.
const Image* gui_image(const ResId id);
id | Resource Identifier. |
Return
The image or NULL
if it is not found.
Remarks
The resource must belong to a package registered with gui_respack. Do not destroy the image as it is managed by Gui.
gui_file ()
Get the contents of a file through its resource identifier.
const byte_t* gui_file(const ResId id, uint32_t *size);
id | Resource Identifier. |
size | Buffer size in bytes. |
Return
File data or NULL
if it is not found.
Remarks
The resource must belong to a package registered with gui_respack. The data is managed by Gui, so there is no need to free memory.
gui_dark_mode ()
Determines if the window environment has a light or dark theme.
bool_t gui_dark_mode(void);
Return
TRUE
for Dark mode, FALSE
for light mode.
gui_alt_color ()
Create a color with two alternative versions.
color_t gui_alt_color(const color_t light_color, const color_t dark_color);
light_color | Color for LIGHT desktop themes. |
dark_color | Color for DARK desktop themes. |
Return
The color.
Remarks
The system will set the final color based on the "lightness" of the window manager colors (Light/Dark). Nested alternate colors ARE NOT ALLOWED. The light
and dark
values must be RGB or system colors.
gui_label_color ()
Returns the default color of text labels Label.
color_t gui_label_color(void);
Return
The color.
gui_view_color ()
Returns the background color in controls View.
color_t gui_view_color(void);
Return
The color.
gui_line_color ()
Returns the color of lines in tables or window separator elements.
color_t gui_line_color(void);
Return
The color.
gui_link_color ()
Returns the color of the text in hyperlinks.
color_t gui_link_color(void);
Return
The color.
gui_border_color ()
Returns the border color in button controls, popups, etc..
color_t gui_border_color(void);
Return
The color.
gui_resolution ()
Returns screen resolution.
S2Df gui_resolution(void);
Return
Resolution.
gui_mouse_pos ()
Returns the position of the mouse cursor.
V2Df gui_mouse_pos(void);
Return
Position.
gui_update ()
Refreshes all application windows, after a theme change.
void
gui_update(void);
Remarks
Normally it is not necessary to call this method. It is called automatically from osapp
.
gui_OnThemeChanged ()
Set a handler to detect the change of the visual theme of the windows environment.
void gui_OnThemeChanged(Listener *listener);
listener | The event handler. |
gui_update_transitions ()
Update the automatic animations of the interface.
void gui_update_transitions(const real64_t prtime, const real64_t crtime);
prtime | Time of the previous instant. |
crtime | Time of the current instant. |
Remarks
Normally it is not necessary to call this method. It is called automatically from osapp
.
gui_OnNotification ()
Sets up a handler to receive notifications from gui
.
void gui_OnNotification(Listener *listener);
listener | The event handler. |
Remarks
See gui_notif_t.
gui_OnIdle ()
Sets a handler to raise an event when the application is in "standby mode".
void gui_OnIdle(Listener *listener);
listener | The event handler. |
Remarks
The event will be raised once when the application's message loop is idle, that is, not processing other pending events. See GUI Events.
gui_info_window ()
Displays an information window about the execution of the program. It is aimed at displaying anomaly messages or debugging messages. Do not use for user information.
uint32_t gui_info_window(const bool_t fatal, const char_t *msg, const char_t *caption, const char_t *detail, const char_t *file, const uint32_t line, const ArrPt(String) *buttons, const uint32_t defindex);
1 2 3 4 5 6 7 8 |
uint32_t sel = 0; ArrPt(String) *buttons = arrpt_create(String); arrpt_append(buttons, str_c("Ok"), String); arrpt_append(buttons, str_c("Bye"), String); arrpt_append(buttons, str_c("Forget"), String); arrpt_append(buttons, str_c("Ask again"), String); sel = gui_info_window(TRUE, "Here an info window", "Something happens", "You do something wrong\nin the file passed as 'stream' parameter.", "your_file.txt", 43, buttons, 1); arrpt_destroy(&buttons, str_destroy, String); |
fatal |
|
msg | Summary message. |
caption | Title, |
detail | Detailed message. Supports '\n'. |
file | File related to the message. |
line | Line related to the message. |
buttons | Buttons. |
defindex | Default button. |
Return
Button number pressed.
evbind_object ()
Gets the object linked to a layout within a callback
function.
type* evbind_object(Event *e, type);
e | The event. |
type | The object type. |
Return
The object.
Remarks
See Notifications and calculated fields.
evbind_modify ()
Checks, inside a callback
function, if the object's field has been modified.
bool_t evbind_modify(Event *e, type, mtype, mname);
e | The event. |
type | The object type. |
mtype | The type of the field to check. |
mname | The name of the field to check. |
Return
TRUE
if the field has been modified.
Remarks
See Notifications and calculated fields.