Draw2D
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
void | draw2d_start (void) |
void | draw2d_finish (void) |
void | draw2d_preferred_monospace (...) |
Types and Constants
enum | pixformat_t |
enum | codec_t |
enum | fstyle_t |
enum | linecap_t |
enum | linejoin_t |
enum | fillwrap_t |
enum | drawop_t |
enum | align_t |
enum | ellipsis_t |
struct | color_t |
struct | DCtx |
struct | Draw |
struct | Palette |
struct | Pixbuf |
struct | Image |
struct | Font |
The Draw2D library integrates all the functionality necessary to create two dimensions vector graphics. It depends directly on Geom2D (Figure 1) and, as we will see later, drawing does not imply having a graphical user interface in the program. It is possible to generate images using an internal memory buffer, without displaying the result in a window.
- 2D Contexts.
- Drawing primitives.
- Colors and Palettes.
- Pixel Buffer and Images.
- Fonts.
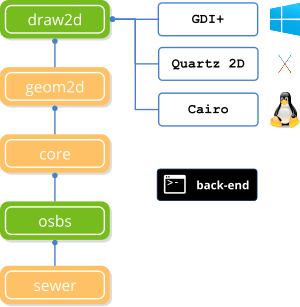
This library connects directly to the native technologies of each operating system: GDI+ on Windows systems, Quartz2D on macOS and Cairo on Linux. In essence, draw2d offers a common and light interface so that the code is portable, delegating the final work in each of them. With this we guarantee three things:
- Efficiency: These APIs have been tested for years and are maintained by system manufacturers.
- Presence: They are integrated as stardard in all computers, so it is not necessary to install additional software.
- Performance: The programs are smaller since they do not require linking with special routines for handling graphics, typography or images.
enum pixformat_t
Pixel format in an image. Number of bits per pixel and color model.
enum pixformat_t
{
ekINDEX1,
ekINDEX2,
ekINDEX4,
ekINDEX8,
ekGRAY8,
ekRGB24,
ekRGBA32,
ekFIMAGE
};
ekINDEX1 | 1 bit per pixel. 2 colors, indexed. |
ekINDEX2 | 2 bits per pixel. 4 colors, indexed. |
ekINDEX4 | 4 bits per pixel. 16 colors, indexed. |
ekINDEX8 | 8 bits per pixel. 256 colors, indexed. |
ekGRAY8 | 8 bits per pixel in grayscale. 256 shades of gray. |
ekRGB24 | 24 bits per RGB pixel. 8 bits per channel (red, green, blue). The lowest order byte corresponds to the red one and the highest one to the blue one. |
ekRGBA32 | 32 bits per pixel RGBA. 8 bits per channel (red, green, blue, alpha). The lowest order byte corresponds to the red one and the highest one to alpha (transparency). |
ekFIMAGE | Represents the original format of the image. Only valid at image_pixels. |
enum codec_t
Image encoding and compression format.
enum codec_t
{
ekJPG,
ekPNG,
ekBMP,
ekGIF
};
ekJPG | Joint Photographic Experts Group. |
ekPNG | Portable Network Graphics. |
ekBMP | BitMaP. |
ekGIF | Graphics Interchange Format. |
enum fstyle_t
Style in typographic fonts. Multiple values can be combined with the OR operator ('|')
.
enum fstyle_t
{
ekFNORMAL,
ekFBOLD,
ekFITALIC,
ekFSTRIKEOUT,
ekFUNDERLINE,
ekFSUBSCRIPT,
ekFSUPSCRIPT,
ekFPIXELS,
ekFPOINTS,
ekFCELL
};
ekFNORMAL | Normal font, no style. Also called Regular. |
ekFBOLD | Bold font. |
ekFITALIC | Italic font. |
ekFSTRIKEOUT |
|
ekFUNDERLINE | Underlined font. |
ekFSUBSCRIPT | Subscript. See textview_fstyle. |
ekFSUPSCRIPT | Superscript. See textview_fstyle |
ekFPIXELS | Font sizes will be indicated in pixels. |
ekFPOINTS | Font sizes will be indicated in points. Size in points. |
ekFCELL | Font sizes will refer to cell height and not character height. |
enum linecap_t
Line end style.
enum linecap_t
{
ekLCFLAT,
ekLCSQUARE,
ekLCROUND
};
ekLCFLAT | Flat termination at the last point of the line. |
ekLCSQUARE | Termination in a box, whose center is the last point of the line. |
ekLCROUND | Termination in a circle, whose center is the last point of the line. |
enum linejoin_t
Line junction style.
enum linejoin_t
{
ekLJMITER,
ekLJROUND,
ekLJBEVEL
};
ekLJMITER | Union at an angle. In very closed angles it is trimmed. |
ekLJROUND | Rounded union. |
ekLJBEVEL | Beveled union. |
enum fillwrap_t
Behavior of the fill pattern in the limits.
enum fillwrap_t
{
ekFCLAMP,
ekFTILE,
ekFFLIP
};
ekFCLAMP | The last limit value is used to fill the outside area. |
ekFTILE | Pattern is repeated. |
ekFFLIP | The pattern is repeated, reversing the order. |
enum drawop_t
Operation to be performed on graphic primitives.
enum drawop_t
{
ekSTROKE,
ekFILL,
ekSKFILL,
ekFILLSK
};
ekSTROKE | Draw the outline of the figure with the default line style. |
ekFILL | Fill the figure area with the default color or pattern. |
ekSKFILL | First draw the outline and then fill in. |
ekFILLSK | First fill in and then draw the outline. |
enum align_t
Alignment values.
enum align_t
{
ekLEFT,
ekTOP,
ekCENTER,
ekRIGHT,
ekBOTTOM,
ekJUSTIFY
};
ekLEFT | Alignment to the left margin. |
ekTOP | Alignment to the upper margin. |
ekCENTER | Centered alignment. |
ekRIGHT | Alignment to the right margin. |
ekBOTTOM | Alignment to the lower margin. |
ekJUSTIFY | Justification or expansion of content. |
enum ellipsis_t
Position of the ellipsis (...) when clipping a text.
enum ellipsis_t
{
ekELLIPNONE,
ekELLIPBEGIN,
ekELLIPMIDDLE,
ekELLIPEND,
ekELLIPMLINE
};
ekELLIPNONE | Without ellipsis. |
ekELLIPBEGIN | Ellipsis at the beginning of the text. |
ekELLIPMIDDLE | Ellipsis in the center of the text. |
ekELLIPEND | Ellipsis at the end of the text. |
ekELLIPMLINE | Multi-line text (without ellipsis). |
struct color_t
32-bit integer representing an RGBA color. The lowest order byte corresponds to the red channel (Red) and the highest order to the Alpha channel (transparency). Colors.
struct color_t;
struct DCtx
2D drawing context, recipient for drawing commands. It is also known as canvas or surface. 2D Contexts.
struct DCtx;
struct Draw
Drawing geometric entities.
struct Drawf; struct Drawd; template<typename real> struct Draw;
struct Palette
Color palette, usually related to indexed Pixbuf. Palettes.
struct Palette;
struct Pixbuf
In-memory buffer with pixel information. Pixel Buffer.
struct Pixbuf;
struct Image
Represents a bitmap image, composed of pixels. Images.
struct Image;
struct Font
Represents a typographic family, size and style with which the texts will be drawn. Fonts.
struct Font;
draw2d_start ()
Start the draw2d library, reserving space for global internal structures. Internally call core_start. In desktop applications, osmain call this function when starting the program.
void
draw2d_start(void);
draw2d_finish ()
Ends the draw2d library, freeing up the space of the global internal structures. Internally call core_finish. In desktop applications, osmain call this function when exiting the program.
void
draw2d_finish(void);
draw2d_preferred_monospace ()
Sets the default monospace font for the font_monospace function.
void draw2d_preferred_monospace(const char_t *family);
family | Monospaced font family. |
Remarks
If this function is not called or the font does not exist, a default one will be set. See Monospace font.