Hello GUI!
- 1. Hello Label!
- 2. Hello Button!
- 3. Hello PopUp and Combo!
- 4. Hello Edit and UpDown!
- 5. Hello TextSel and Clipboard!
- 6. Hello TextEditor!
- 7. Hello ListBox!
- 8. Hello Slider and Progress!
- 9. Hello TextView!
- 10. Hello TableView!
- 11. Hello SplitView!
- 12. Hello Modal Window!
- 13. Hello Overlay Window!
- 14. Hello Gui Binding!
- 15. Hello Struct Binding!
- 16. Hello Sublayout!
- 17. Hello Subpanel!
- 18. Hello Multi-layout!
- 19. Hello Scroll-Panel!
- 20. Hello dynamic Layout!
- 21. Hello dynamic Menu!
- 22. Hello IP-Input!
- 23. Hello Font Stretch!
GuiHello is an application, which by examples, shows Gui library features for the creation of user interfaces. The source code is in folder /demo/guihello
of the SDK distribution.
1. Hello Label!
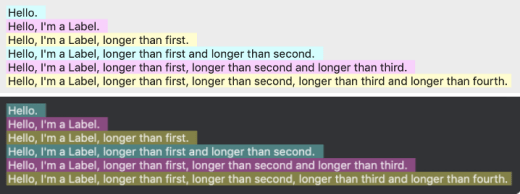
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 |
/* Labels basics */ #include "labels.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static const char_t *i_LABEL_01 = "Hello."; static const char_t *i_LABEL_02 = "Hello, I'm a Label."; static const char_t *i_LABEL_03 = "Hello, I'm a Label, longer than first."; static const char_t *i_LABEL_04 = "Hello, I'm a Label, longer than first and longer than second."; static const char_t *i_LABEL_05 = "Hello, I'm a Label, longer than first, longer than second and longer than third."; static const char_t *i_LABEL_06 = "Hello, I'm a Label, longer than first, longer than second, longer than third and longer than fourth."; static const char_t *i_LABEL_07 = "Mouse sensitive label"; /*---------------------------------------------------------------------------*/ static void i_OnLayoutWidth(Layout *layout, Event *event) { const EvButton *p = event_params(event, EvButton); real32_t width = 0; switch (p->index) { case 0: width = 0; break; case 1: width = 100; break; case 2: width = 200; break; case 3: width = 300; break; case 4: width = 400; break; cassert_default(); } layout_hsize(layout, 0, width); layout_update(layout); } /*---------------------------------------------------------------------------*/ static PopUp *i_width_popup(Layout *layout) { PopUp *popup = popup_create(); popup_add_elem(popup, "Natural", NULL); popup_add_elem(popup, "100px", NULL); popup_add_elem(popup, "200px", NULL); popup_add_elem(popup, "300px", NULL); popup_add_elem(popup, "400px", NULL); popup_OnSelect(popup, listener(layout, i_OnLayoutWidth, Layout)); return popup; } /*---------------------------------------------------------------------------*/ static Panel *i_labels(const bool_t multiline) { Panel *panel = panel_create(); Layout *layout = layout_create(1, 7); PopUp *popup = i_width_popup(layout); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); color_t c1 = gui_alt_color(color_rgb(192, 255, 255), color_rgb(48, 112, 112)); color_t c2 = gui_alt_color(color_rgb(255, 192, 255), color_rgb(128, 48, 112)); color_t c3 = gui_alt_color(color_rgb(255, 255, 192), color_rgb(112, 112, 48)); label_multiline(label1, multiline); label_multiline(label2, multiline); label_multiline(label3, multiline); label_multiline(label4, multiline); label_multiline(label5, multiline); label_multiline(label6, multiline); label_text(label1, i_LABEL_01); label_text(label2, i_LABEL_02); label_text(label3, i_LABEL_03); label_text(label4, i_LABEL_04); label_text(label5, i_LABEL_05); label_text(label6, i_LABEL_06); label_bgcolor(label1, c1); label_bgcolor(label2, c2); label_bgcolor(label3, c3); label_bgcolor(label4, c1); label_bgcolor(label5, c2); label_bgcolor(label6, c3); layout_popup(layout, popup, 0, 0); layout_label(layout, label1, 0, 1); layout_label(layout, label2, 0, 2); layout_label(layout, label3, 0, 3); layout_label(layout, label4, 0, 4); layout_label(layout, label5, 0, 5); layout_label(layout, label6, 0, 6); layout_vmargin(layout, 0, 5); panel_layout(panel, layout); return panel; } /*---------------------------------------------------------------------------*/ Panel *labels_single_line(void) { return i_labels(FALSE); } /*---------------------------------------------------------------------------*/ Panel *labels_multi_line(void) { return i_labels(TRUE); } /*---------------------------------------------------------------------------*/ Panel *labels_mouse_over(void) { Panel *panel = panel_create(); Layout *layout = layout_create(1, 5); Font *font = font_system(20, ekFNORMAL | ekFPIXELS); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); label_text(label1, i_LABEL_07); label_text(label2, i_LABEL_07); label_text(label3, i_LABEL_07); label_text(label4, i_LABEL_07); label_text(label5, i_LABEL_07); label_font(label1, font); label_font(label2, font); label_font(label3, font); label_font(label4, font); label_font(label5, font); label_color_over(label1, kCOLOR_RED); label_color_over(label2, kCOLOR_RED); label_color_over(label3, kCOLOR_RED); label_color_over(label4, kCOLOR_RED); label_color_over(label5, kCOLOR_RED); label_style_over(label1, ekFBOLD); label_style_over(label2, ekFITALIC); label_style_over(label3, ekFSTRIKEOUT); label_style_over(label4, ekFUNDERLINE); label_bgcolor_over(label5, kCOLOR_CYAN); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 0, 1); layout_label(layout, label3, 0, 2); layout_label(layout, label4, 0, 3); layout_label(layout, label5, 0, 4); panel_layout(panel, layout); font_destroy(&font); return panel; } |
2. Hello Button!
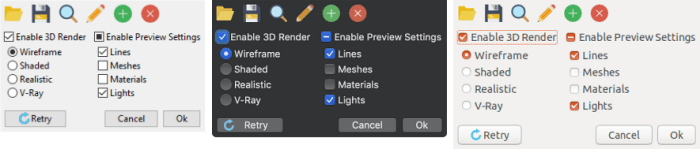
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 |
/* Buttons demo */ #include "buttons.h" #include "res_guihello.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static Layout *i_flatbuttons(void) { Layout *layout = layout_create(6, 1); Button *button1 = button_flat(); Button *button2 = button_flat(); Button *button3 = button_flat(); Button *button4 = button_flat(); Button *button5 = button_flat(); Button *button6 = button_flat(); button_text(button1, "Open File"); button_text(button2, "Save File"); button_text(button3, "Search File"); button_text(button4, "Edit File"); button_text(button5, "Add File"); button_text(button6, "Delete File"); button_image(button1, gui_image(FOLDER24_PNG)); button_image(button2, gui_image(DISK24_PNG)); button_image(button3, gui_image(SEARCH24_PNG)); button_image(button4, gui_image(EDIT24_PNG)); button_image(button5, gui_image(PLUS24_PNG)); button_image(button6, gui_image(ERROR24_PNG)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 1, 0); layout_button(layout, button3, 2, 0); layout_button(layout, button4, 3, 0); layout_button(layout, button5, 4, 0); layout_button(layout, button6, 5, 0); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_radios(void) { Layout *layout = layout_create(1, 4); Button *radio1 = button_radio(); Button *radio2 = button_radio(); Button *radio3 = button_radio(); Button *radio4 = button_radio(); button_text(radio1, "&Wireframe"); button_text(radio2, "&Shaded"); button_text(radio3, "&Realistic"); button_text(radio4, "&V-Ray"); button_state(radio1, ekGUI_ON); layout_button(layout, radio1, 0, 0); layout_button(layout, radio2, 0, 1); layout_button(layout, radio3, 0, 2); layout_button(layout, radio4, 0, 3); layout_margin(layout, 5); layout_vmargin(layout, 0, 3); layout_vmargin(layout, 1, 3); layout_vmargin(layout, 2, 3); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_checks(void) { Layout *layout = layout_create(1, 4); Button *check1 = button_check(); Button *check2 = button_check(); Button *check3 = button_check(); Button *check4 = button_check(); button_text(check1, "&Lines"); button_text(check2, "M&eshes"); button_text(check3, "M&aterials"); button_text(check4, "L&ights"); button_state(check1, ekGUI_ON); button_state(check2, ekGUI_OFF); button_state(check3, ekGUI_OFF); button_state(check4, ekGUI_ON); layout_button(layout, check1, 0, 0); layout_button(layout, check2, 0, 1); layout_button(layout, check3, 0, 2); layout_button(layout, check4, 0, 3); layout_margin(layout, 5); layout_vmargin(layout, 0, 3); layout_vmargin(layout, 1, 3); layout_vmargin(layout, 2, 3); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_pushes(Button **defbutton) { Layout *layout = layout_create(4, 1); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); button_text(button1, "Re&try"); button_text(button2, "&Cancel"); button_text(button3, "&Ok"); button_image(button1, gui_image(RETRY_PNG)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 2, 0); layout_button(layout, button3, 3, 0); layout_hmargin(layout, 2, 5); layout_hexpand(layout, 1); *defbutton = button1; return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_buttons(Button **defbutton) { Layout *layout = layout_create(1, 3); Layout *layout1 = i_flatbuttons(); Layout *layout2 = layout_create(2, 2); Layout *layout3 = i_radios(); Layout *layout4 = i_checks(); Layout *layout5 = i_pushes(defbutton); Button *check1 = button_check(); Button *check2 = button_check3(); button_text(check1, "Enable 3&D Render"); button_text(check2, "Enable &Preview Settings"); button_state(check1, ekGUI_ON); button_state(check2, ekGUI_MIXED); layout_layout(layout, layout1, 0, 0); layout_button(layout2, check1, 0, 0); layout_layout(layout2, layout3, 0, 1); layout_button(layout2, check2, 1, 0); layout_layout(layout2, layout4, 1, 1); layout_layout(layout, layout2, 0, 1); layout_layout(layout, layout5, 0, 2); layout_halign(layout, 0, 0, ekLEFT); layout_margin(layout2, 5); layout_hmargin(layout2, 0, 10); layout_margin(layout5, 5); return layout; } /*---------------------------------------------------------------------------*/ Panel *buttons_basics(Button **defbutton) { Layout *layout = i_buttons(defbutton); Panel *panel = panel_create(); panel_layout(panel, layout); return panel; } |
3. Hello PopUp and Combo!
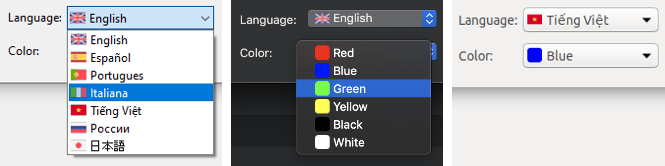
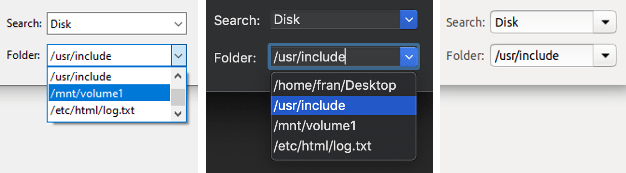
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 |
/* PopUp and Combo */ #include "popcom.h" #include "res_guihello.h" #include <gui/guiall.h> typedef struct _popupdata_t PopUpData; struct _popupdata_t { PopUp *popup; }; /*---------------------------------------------------------------------------*/ static void i_destroy_data(PopUpData **data) { heap_delete(data, PopUpData); } /*---------------------------------------------------------------------------*/ static void i_popups(Layout *layout, PopUpData *data) { Label *label1 = label_create(); Label *label2 = label_create(); PopUp *popup1 = popup_create(); PopUp *popup2 = popup_create(); label_text(label1, "Language:"); label_text(label2, "Color:"); popup_add_elem(popup1, "English", gui_image(UKING_PNG)); popup_add_elem(popup1, "Español", gui_image(SPAIN_PNG)); popup_add_elem(popup1, "Portugues", gui_image(PORTUGAL_PNG)); popup_add_elem(popup1, "Italiana", gui_image(ITALY_PNG)); popup_add_elem(popup1, "Tiếng Việt", gui_image(VIETNAM_PNG)); popup_add_elem(popup1, "России", gui_image(RUSSIA_PNG)); popup_add_elem(popup1, "日本語", gui_image(JAPAN_PNG)); popup_add_elem(popup2, "Red", gui_image(RED_PNG)); popup_add_elem(popup2, "Blue", gui_image(BLUE_PNG)); popup_add_elem(popup2, "Green", gui_image(GREEN_PNG)); popup_add_elem(popup2, "Yellow", gui_image(YELLOW_PNG)); popup_add_elem(popup2, "Black", gui_image(BLACK_PNG)); popup_add_elem(popup2, "White", gui_image(WHITE_PNG)); popup_list_height(popup1, 10); popup_list_height(popup2, 10); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 0, 1); layout_popup(layout, popup1, 1, 0); layout_popup(layout, popup2, 1, 1); data->popup = popup1; } /*---------------------------------------------------------------------------*/ static void i_combos(Layout *layout) { Label *label1 = label_create(); Label *label2 = label_create(); Combo *combo1 = combo_create(); Combo *combo2 = combo_create(); label_text(label1, "Search:"); label_text(label2, "Folder:"); combo_add_elem(combo1, "Search", NULL); combo_add_elem(combo1, "Disk", NULL); combo_add_elem(combo1, "Edit", NULL); combo_add_elem(combo2, "/home/fran/Desktop", NULL); combo_add_elem(combo2, "/usr/include", NULL); combo_add_elem(combo2, "/mnt/volume1", NULL); combo_add_elem(combo2, "/etc/html/log.txt", NULL); layout_label(layout, label1, 2, 0); layout_label(layout, label2, 2, 1); layout_combo(layout, combo1, 3, 0); layout_combo(layout, combo2, 3, 1); } /*---------------------------------------------------------------------------*/ static void i_OnAdd(PopUpData *data, Event *e) { cassert_no_null(data); unref(e); popup_add_elem(data->popup, "Español", gui_image(SPAIN_PNG)); } /*---------------------------------------------------------------------------*/ static void i_OnClear(PopUpData *data, Event *e) { cassert_no_null(data); unref(e); popup_clear(data->popup); } /*---------------------------------------------------------------------------*/ Panel *popup_combo(void) { PopUpData *data = heap_new0(PopUpData); Panel *panel = panel_create(); Layout *layout = layout_create(4, 4); Button *button1 = button_push(); Button *button2 = button_push(); i_popups(layout, data); i_combos(layout); button_text(button1, "Add elem to PopUp"); button_text(button2, "Clear PopUp"); button_OnClick(button1, listener(data, i_OnAdd, PopUpData)); button_OnClick(button2, listener(data, i_OnClear, PopUpData)); layout_button(layout, button1, 1, 2); layout_button(layout, button2, 1, 3); layout_margin(layout, 10); layout_vmargin(layout, 0, 10); layout_vmargin(layout, 1, 10); layout_vmargin(layout, 2, 5); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 10); layout_hmargin(layout, 2, 5); layout_hsize(layout, 1, 150); layout_hsize(layout, 3, 150); panel_layout(panel, layout); panel_data(panel, &data, i_destroy_data, PopUpData); return panel; } |
4. Hello Edit and UpDown!
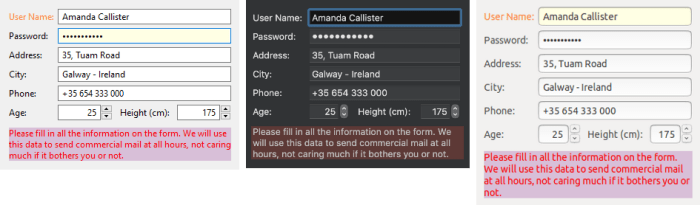
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 |
/* Form demo */ #include "form.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ typedef struct _form_data_t FormData; struct _form_data_t { Window *window; Window *modal_window; Button *validate_check; }; #define BUTTON_YES 1000 #define BUTTON_NO 1001 /*---------------------------------------------------------------------------*/ static void i_destroy_data(FormData **data) { cassert_no_null(data); cassert_no_null(*data); ptr_destopt(window_destroy, &(*data)->modal_window, Window); heap_delete(data, FormData); } /*---------------------------------------------------------------------------*/ static void i_OnFilter(void *noused, Event *e) { const EvText *params = event_params(e, EvText); EvTextFilter *result = event_result(e, EvTextFilter); uint32_t i = 0, j = 0; while (params->text[i] != '\0') { if (params->text[i] >= '0' && params->text[i] <= '9') { result->text[j] = params->text[i]; j += 1; } i += 1; } result->text[j] = '\0'; result->apply = TRUE; unref(noused); } /*---------------------------------------------------------------------------*/ static void i_OnModalButton(FormData *data, Event *e) { Button *button = event_sender(e, Button); uint32_t tag = button_get_tag(button); window_stop_modal(data->modal_window, tag); } /*---------------------------------------------------------------------------*/ static Window *i_modal_window(FormData *data, Edit *edit, const GuiControl *next, const char_t *field_text, const char_t *action_text) { Layout *layout1 = layout_create(1, 2); Layout *layout2 = layout_create(3, 1); Label *label = label_create(); Button *button1 = button_push(); Button *button2 = button_push(); Panel *panel = panel_create(); Window *window = window_create(ekWINDOW_STD | ekWINDOW_ESC); String *str = str_printf("Do you want to validate the text '%s' of the EditBox '%p'? The focus will be moved to the '%p' control using the '%s' action.", field_text, (void *)edit, (void *)next, action_text); label_multiline(label, TRUE); label_text(label, tc(str)); button_text(button1, "Yes"); button_text(button2, "No"); button_tag(button1, BUTTON_YES); button_tag(button2, BUTTON_NO); button_OnClick(button1, listener(data, i_OnModalButton, FormData)); button_OnClick(button2, listener(data, i_OnModalButton, FormData)); layout_label(layout1, label, 0, 0); layout_button(layout2, button1, 1, 0); layout_button(layout2, button2, 2, 0); layout_layout(layout1, layout2, 0, 1); layout_hsize(layout1, 0, 250); layout_vmargin(layout1, 0, 10); layout_hmargin(layout2, 1, 5); layout_margin4(layout2, 0, 10, 0, 0); layout_margin(layout1, 10); layout_hexpand(layout2, 0); window_title(window, "Data validation"); panel_layout(panel, layout1); window_panel(window, panel); window_defbutton(window, button1); str_destroy(&str); return window; } /*---------------------------------------------------------------------------*/ static const char_t *i_action_text(const gui_tab_t action) { switch (action) { case ekGUI_TAB_KEY: return "TAB_KEY"; case ekGUI_TAB_BACKKEY: return "TAB_BACKKEY"; case ekGUI_TAB_NEXT: return "TAB_NEXT"; case ekGUI_TAB_PREV: return "TAB_PREV"; case ekGUI_TAB_MOVE: return "TAB_MOVE"; case ekGUI_TAB_CLICK: return "TAB_CLICK"; } return "TAB_UNKNOWN"; } /*---------------------------------------------------------------------------*/ static V2Df i_modal_pos(Window *window, Window *parent) { V2Df pos = window_get_origin(parent); S2Df s1 = window_get_size(parent); S2Df s2 = window_get_size(window); real32_t x = pos.x + ((s1.width - s2.width) / 2); real32_t y = pos.y + ((s1.height - s2.height) / 2); return v2df(x, y); } /*---------------------------------------------------------------------------*/ static bool_t i_validate_field(FormData *data, Edit *edit, const char_t *text) { FocusInfo info; const char_t *action_text = NULL; uint32_t modal_value = UINT32_MAX; V2Df pos; cassert_no_null(data); cassert(data->modal_window == NULL); window_focus_info(data->window, &info); action_text = i_action_text(info.action); data->modal_window = i_modal_window(data, edit, info.next, text, action_text); pos = i_modal_pos(data->modal_window, data->window); window_origin(data->modal_window, pos); modal_value = window_modal(data->modal_window, data->window); window_destroy(&data->modal_window); switch (modal_value) { case ekGUI_CLOSE_BUTTON: case ekGUI_CLOSE_ESC: case BUTTON_NO: return FALSE; case BUTTON_YES: return TRUE; cassert_default(); } return TRUE; } /*---------------------------------------------------------------------------*/ static void i_OnEditChange(FormData *data, Event *e) { const EvText *p = event_params(e, EvText); Edit *edit = event_sender(e, Edit); cassert_no_null(data); if (button_get_state(data->validate_check) == ekGUI_ON) { bool_t *r = event_result(e, bool_t); *r = i_validate_field(data, edit, p->text); } } /*---------------------------------------------------------------------------*/ static void i_OnUpDown(Edit *edit, Event *e) { const EvButton *params = event_params(e, EvButton); int32_t n = str_to_i32(edit_get_text(edit), 10, NULL); char_t text[64]; n += (params->index == 0) ? 1 : -1; bstd_sprintf(text, sizeof(text), "%d", n); edit_text(edit, text); } /*---------------------------------------------------------------------------*/ static Layout *i_numbers(FormData *data, color_t colorbg) { Layout *layout = layout_create(5, 1); Label *label = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); UpDown *updown1 = updown_create(); UpDown *updown2 = updown_create(); label_text(label, "Height (cm):"); edit_text(edit1, "25"); edit_text(edit2, "175"); edit_autoselect(edit1, TRUE); edit_align(edit1, ekRIGHT); edit_align(edit2, ekRIGHT); edit_OnFilter(edit1, listener(NULL, i_OnFilter, void)); edit_OnFilter(edit2, listener(NULL, i_OnFilter, void)); edit_OnChange(edit1, listener(data, i_OnEditChange, FormData)); edit_OnChange(edit2, listener(data, i_OnEditChange, FormData)); edit_bgcolor_focus(edit1, colorbg); edit_bgcolor_focus(edit2, colorbg); updown_OnClick(updown1, listener(edit1, i_OnUpDown, Edit)); updown_OnClick(updown2, listener(edit2, i_OnUpDown, Edit)); updown_tooltip(updown1, "Increase/Decrease age"); updown_tooltip(updown2, "Increase/Decrease height"); layout_label(layout, label, 2, 0); layout_edit(layout, edit1, 0, 0); layout_edit(layout, edit2, 3, 0); layout_updown(layout, updown1, 1, 0); layout_updown(layout, updown2, 4, 0); layout_hmargin(layout, 1, 10.f); layout_hmargin(layout, 2, 10.f); layout_hexpand2(layout, 0, 3, .5f); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_edits(FormData *data) { color_t colorbg = gui_alt_color(color_rgb(255, 255, 192), color_rgb(112, 112, 48)); Layout *layout1 = layout_create(2, 6); Layout *layout2 = i_numbers(data, colorbg); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); Edit *edit3 = edit_create(); Edit *edit4 = edit_create(); Edit *edit5 = edit_create(); label_text(label1, "User Name:"); label_text(label2, "Password:"); label_text(label3, "Address:"); label_text(label4, "City:"); label_text(label5, "Phone:"); label_text(label6, "Age:"); label_color_over(label1, color_rgb(255, 128, 52)); label_color_over(label2, color_rgb(70, 129, 207)); label_color_over(label3, color_rgb(119, 188, 31)); label_style_over(label4, ekFITALIC | ekFUNDERLINE); edit_text(edit1, "Amanda Callister"); edit_text(edit2, "aQwe56nhjJk"); edit_text(edit3, "35, Tuam Road"); edit_text(edit4, "Galway - Ireland"); edit_text(edit5, "+35 654 333 000"); edit_OnChange(edit1, listener(data, i_OnEditChange, FormData)); edit_OnChange(edit2, listener(data, i_OnEditChange, FormData)); edit_OnChange(edit3, listener(data, i_OnEditChange, FormData)); edit_OnChange(edit4, listener(data, i_OnEditChange, FormData)); edit_OnChange(edit5, listener(data, i_OnEditChange, FormData)); edit_select(edit1, 2, 6); edit_passmode(edit2, TRUE); edit_bgcolor_focus(edit1, colorbg); edit_bgcolor_focus(edit2, colorbg); edit_bgcolor_focus(edit3, colorbg); edit_bgcolor_focus(edit4, colorbg); edit_bgcolor_focus(edit5, colorbg); layout_label(layout1, label1, 0, 0); layout_label(layout1, label2, 0, 1); layout_label(layout1, label3, 0, 2); layout_label(layout1, label4, 0, 3); layout_label(layout1, label5, 0, 4); layout_label(layout1, label6, 0, 5); layout_edit(layout1, edit1, 1, 0); layout_edit(layout1, edit2, 1, 1); layout_edit(layout1, edit3, 1, 2); layout_edit(layout1, edit4, 1, 3); layout_edit(layout1, edit5, 1, 4); layout_layout(layout1, layout2, 1, 5); layout_hmargin(layout1, 0, 5); layout_hexpand(layout1, 1); layout_vmargin(layout1, 0, 5); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 2, 5); layout_vmargin(layout1, 3, 5); layout_vmargin(layout1, 4, 5); return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_toolbar(FormData *data) { Layout *layout = layout_create(1, 1); Button *check = button_check(); button_text(check, "Field validations"); layout_button(layout, check, 0, 0); data->validate_check = check; return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_form(FormData *data) { Layout *layout1 = layout_create(1, 3); Layout *layout2 = i_edits(data); Layout *layout3 = i_toolbar(data); Label *label = label_create(); cassert_no_null(data); label_multiline(label, TRUE); label_text(label, "Please fill in all the information on the form. We will use this data to send commercial mail at all hours, not caring much if it bothers you or not."); label_color(label, gui_alt_color(color_rgb(255, 0, 0), color_rgb(180, 180, 180))); label_bgcolor(label, gui_alt_color(color_rgb(216, 191, 216), color_rgb(80, 40, 40))); label_bgcolor_over(label, gui_alt_color(color_rgb(255, 250, 205), color_rgb(105, 100, 55))); label_style_over(label, ekFUNDERLINE); layout_layout(layout1, layout2, 0, 0); layout_layout(layout1, layout3, 0, 1); layout_label(layout1, label, 0, 2); layout_hsize(layout1, 0, 300); layout_halign(layout1, 0, 1, ekLEFT); layout_vmargin(layout1, 0, 10); layout_vmargin(layout1, 1, 10); layout_margin(layout1, 10); layout_tabstop(layout1, 0, 1, FALSE); return layout1; } /*---------------------------------------------------------------------------*/ static FormData *i_form_data(Window *window) { FormData *data = heap_new0(FormData); data->window = window; return data; } /*---------------------------------------------------------------------------*/ Panel *form_basic(Window *window) { FormData *data = i_form_data(window); Layout *layout = i_form(data); Panel *panel = panel_create(); panel_data(panel, &data, i_destroy_data, FormData); panel_layout(panel, layout); return panel; } |
5. Hello TextSel and Clipboard!
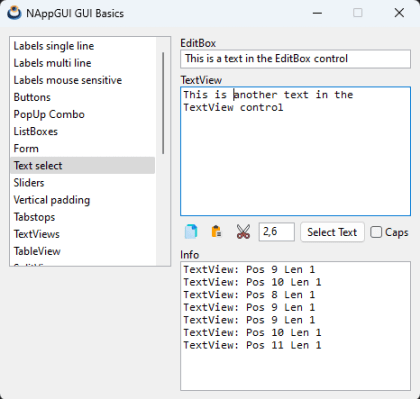
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 |
/* Text selection and clipboard demo */ #include "seltext.h" #include "res_guihello.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ typedef struct _sel_data_t SelData; struct _sel_data_t { Window *window; Edit *edit_range; Button *caps; TextView *text; TextView *info_text; }; /*---------------------------------------------------------------------------*/ static void i_destroy_data(SelData **data) { heap_delete(data, SelData); } /*---------------------------------------------------------------------------*/ static void i_OnCopy(SelData *data, Event *e) { GuiControl *control = NULL; cassert_no_null(data); unref(e); control = window_get_focus(data->window); if (guicontrol_edit(control) != NULL) edit_copy(guicontrol_edit(control)); else if (guicontrol_textview(control) != NULL) textview_copy(guicontrol_textview(control)); } /*---------------------------------------------------------------------------*/ static void i_OnPaste(SelData *data, Event *e) { GuiControl *control = NULL; cassert_no_null(data); unref(e); control = window_get_focus(data->window); if (guicontrol_edit(control) != NULL) edit_paste(guicontrol_edit(control)); else if (guicontrol_textview(control) != NULL) textview_paste(guicontrol_textview(control)); } /*---------------------------------------------------------------------------*/ static void i_OnCut(SelData *data, Event *e) { GuiControl *control = NULL; cassert_no_null(data); unref(e); control = window_get_focus(data->window); if (guicontrol_edit(control) != NULL) edit_cut(guicontrol_edit(control)); else if (guicontrol_textview(control) != NULL) textview_cut(guicontrol_textview(control)); } /*---------------------------------------------------------------------------*/ static void i_OnSelect(SelData *data, Event *e) { GuiControl *control = NULL; const char_t *range = NULL; String *left = NULL, *right = NULL; int32_t start = 0, end = 0; cassert_no_null(data); unref(e); control = window_get_focus(data->window); range = edit_get_text(data->edit_range); str_split_trim(range, ",", &left, &right); start = str_to_i32(tc(left), 10, NULL); end = str_to_i32(tc(right), 10, NULL); if (guicontrol_edit(control) != NULL) edit_select(guicontrol_edit(control), start, end); else if (guicontrol_textview(control) != NULL) textview_select(guicontrol_textview(control), start, end); str_destroy(&left); str_destroy(&right); } /*---------------------------------------------------------------------------*/ static void i_OnWrap(SelData *data, Event *e) { const EvButton *p = event_params(e, EvButton); cassert_no_null(data); textview_wrap(data->text, p->state == ekGUI_ON ? TRUE : FALSE); } /*---------------------------------------------------------------------------*/ static void i_filter_event(SelData *data, Event *e, const bool_t from_editbox) { const EvText *p = event_params(e, EvText); cassert_no_null(data); /* * Convert the inserted text in caps. * p->text the control current text (const). * r->text the new filterd text. * p->cpos current caret position. * r->apply = TRUE means the editbox text has to be updated. * p->len number of chars inserted at left of caret (to caps). * We are working with UTF8-Strings. Sequential access. */ if (button_get_state(data->caps) == ekGUI_ON && p->len > 0) { EvTextFilter *r = event_result(e, EvTextFilter); const char_t *src = p->text; char_t *dest = r->text; uint32_t cp = unicode_to_u32(src, ekUTF8); uint32_t pos = 0; while (cp != 0) { uint32_t offset = 0; if ((pos >= p->cpos - p->len && pos < p->cpos) || !from_editbox) { if (cp >= 'a' && cp <= 'z') cp -= 32; } offset = unicode_to_char(cp, dest, ekUTF8); dest += offset; src = unicode_next(src, ekUTF8); cp = unicode_to_u32(src, ekUTF8); pos += 1; } *dest = 0; r->cpos = p->cpos; r->apply = TRUE; } textview_printf(data->info_text, "%s OnFilter: Pos %d Len %d\n", from_editbox ? "Edit" : "TextView", p->cpos, p->len); textview_scroll_caret(data->info_text); } /*---------------------------------------------------------------------------*/ static void i_OnEditFilter(SelData *data, Event *e) { i_filter_event(data, e, TRUE); } /*---------------------------------------------------------------------------*/ static void i_OnEditChange(SelData *data, Event *e) { const EvText *p = event_params(e, EvText); cassert_no_null(data); textview_printf(data->info_text, "Edit OnChange: Pos: %d Len %d\n", p->cpos, p->len); textview_scroll_caret(data->info_text); } /*---------------------------------------------------------------------------*/ static void i_OnTextViewFilter(SelData *data, Event *e) { i_filter_event(data, e, FALSE); } /*---------------------------------------------------------------------------*/ static Layout *i_text_controls(SelData *data) { Layout *layout = layout_create(7, 1); Button *button1 = button_flat(); Button *button2 = button_flat(); Button *button3 = button_flat(); Button *button4 = button_push(); Button *button5 = button_check(); Button *button6 = button_check(); Edit *edit = edit_create(); button_image(button1, gui_image(COPY_PNG)); button_image(button2, gui_image(PASTE_PNG)); button_image(button3, gui_image(CUT_PNG)); edit_text(edit, "2,6"); button_OnClick(button1, listener(data, i_OnCopy, SelData)); button_OnClick(button2, listener(data, i_OnPaste, SelData)); button_OnClick(button3, listener(data, i_OnCut, SelData)); button_tooltip(button1, "Copy"); button_tooltip(button2, "Paste"); button_tooltip(button3, "Cut"); button_text(button4, "Select Text"); button_text(button5, "Caps"); button_text(button6, "Wrap"); button_OnClick(button4, listener(data, i_OnSelect, SelData)); button_OnClick(button6, listener(data, i_OnWrap, SelData)); button_state(button6, ekGUI_ON); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 1, 0); layout_button(layout, button3, 2, 0); layout_edit(layout, edit, 3, 0); layout_button(layout, button4, 4, 0); layout_button(layout, button5, 5, 0); layout_button(layout, button6, 6, 0); layout_hsize(layout, 3, 40); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_hmargin(layout, 2, 5); layout_hmargin(layout, 3, 5); layout_hmargin(layout, 4, 5); layout_hmargin(layout, 5, 5); data->edit_range = edit; data->caps = button5; return layout; } /*---------------------------------------------------------------------------*/ static void i_OnAddText(SelData *data, Event *e) { cassert_no_null(data); textview_printf(data->text, "%s", "add"); unref(e); } /*---------------------------------------------------------------------------*/ static void i_OnInsertText(SelData *data, Event *e) { cassert_no_null(data); textview_cpos_printf(data->text, "%s", "ins"); unref(e); } /*---------------------------------------------------------------------------*/ static void i_OnDeleteText(SelData *data, Event *e) { cassert_no_null(data); textview_del_select(data->text); unref(e); } /*---------------------------------------------------------------------------*/ static Layout *i_textview_controls(SelData *data) { Layout *layout = layout_create(5, 1); Label *label = label_create(); Button *button1 = button_flat(); Button *button2 = button_flat(); Button *button3 = button_flat(); label_text(label, "TextView"); button_image(button1, gui_image(EDIT16_PNG)); button_image(button2, gui_image(CURSOR16_PNG)); button_image(button3, gui_image(ERROR16_PNG)); button_OnClick(button1, listener(data, i_OnAddText, SelData)); button_OnClick(button2, listener(data, i_OnInsertText, SelData)); button_OnClick(button3, listener(data, i_OnDeleteText, SelData)); button_tooltip(button1, "Add text at the end of TextView"); button_tooltip(button2, "Insert text at cursor position in TextView"); button_tooltip(button3, "Delete the selected text, without copy into clipboard"); layout_label(layout, label, 0, 0); layout_button(layout, button1, 2, 0); layout_button(layout, button2, 3, 0); layout_button(layout, button3, 4, 0); layout_hexpand(layout, 1); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_layout(SelData *data) { Layout *layout1 = layout_create(1, 11); Layout *layout2 = i_textview_controls(data); Layout *layout3 = i_text_controls(data); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label5 = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_multiline(); Edit *edit3 = edit_multiline(); TextView *text1 = textview_create(); TextView *text2 = textview_create(); cassert_no_null(data); label_text(label1, "EditBox"); label_text(label2, "EditBox (multiline)"); label_text(label3, "EditBox (multiline with user-height)"); label_text(label5, "Info"); edit_text(edit1, "This is a text in the EditBox control"); edit_text(edit2, "This is a text in the multiline EditBox control"); edit_text(edit3, "This is a text in the multiline EditBox control"); edit_min_height(edit3, 100); edit_OnFilter(edit1, listener(data, i_OnEditFilter, SelData)); edit_OnFilter(edit2, listener(data, i_OnEditFilter, SelData)); edit_OnFilter(edit3, listener(data, i_OnEditFilter, SelData)); edit_OnChange(edit1, listener(data, i_OnEditChange, SelData)); edit_OnChange(edit2, listener(data, i_OnEditChange, SelData)); edit_OnChange(edit3, listener(data, i_OnEditChange, SelData)); textview_writef(text1, "This is another text in the TextView control, wider than the control."); textview_editable(text1, TRUE); textview_OnFilter(text1, listener(data, i_OnTextViewFilter, SelData)); layout_label(layout1, label1, 0, 0); layout_edit(layout1, edit1, 0, 1); layout_label(layout1, label2, 0, 2); layout_edit(layout1, edit2, 0, 3); layout_label(layout1, label3, 0, 4); layout_edit(layout1, edit3, 0, 5); layout_layout(layout1, layout2, 0, 6); layout_textview(layout1, text1, 0, 7); layout_layout(layout1, layout3, 0, 8); layout_label(layout1, label5, 0, 9); layout_textview(layout1, text2, 0, 10); layout_tabstop(layout1, 0, 6, FALSE); layout_tabstop(layout1, 0, 7, TRUE); layout_tabstop(layout1, 0, 8, FALSE); layout_halign(layout1, 0, 8, ekLEFT); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 3, 5); layout_vmargin(layout1, 5, 5); layout_vmargin(layout1, 7, 5); data->text = text1; data->info_text = text2; return layout1; } /*---------------------------------------------------------------------------*/ static SelData *i_seldata(Window *window) { SelData *data = heap_new0(SelData); data->window = window; return data; } /*---------------------------------------------------------------------------*/ Panel *seltext(Window *window) { SelData *data = i_seldata(window); Layout *layout = i_layout(data); Panel *panel = panel_create(); panel_data(panel, &data, i_destroy_data, SelData); panel_layout(panel, layout); return panel; } |
6. Hello TextEditor!
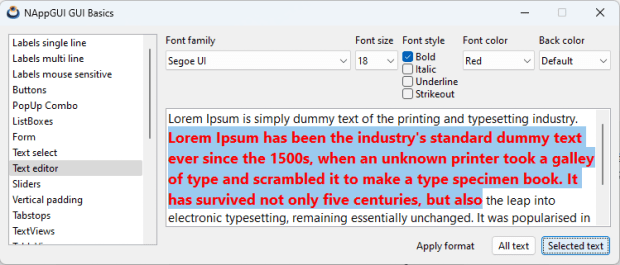
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 |
/* Text editor demo */ #include "editor.h" #include "res_guihello.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ typedef struct _edit_data_t EditData; struct _edit_data_t { ArrPt(String) *fonts; Window *window; TextView *text; PopUp *family_popup; PopUp *size_popup; PopUp *color_popup; PopUp *back_popup; Button *bold_check; Button *italic_check; Button *under_check; Button *strike_check; }; /*---------------------------------------------------------------------------*/ static color_t i_COLORS[9]; /*---------------------------------------------------------------------------*/ static void i_destroy_data(EditData **data) { cassert_no_null(data); cassert_no_null(*data); arrpt_destroy(&(*data)->fonts, str_destroy, String); heap_delete(data, EditData); } /*---------------------------------------------------------------------------*/ static void i_set_params(EditData *data) { const char_t *ffamily = NULL; const char_t *tsize = NULL; real32_t fsize = 0; uint32_t fstyle = 0; color_t color = 0; color_t back = 0; cassert_no_null(data); /* Get text attribs from GUI controls */ ffamily = popup_get_text(data->family_popup, popup_get_selected(data->family_popup)); tsize = popup_get_text(data->size_popup, popup_get_selected(data->size_popup)); fsize = str_to_r32(tsize, NULL); if (button_get_state(data->bold_check) == ekGUI_ON) fstyle |= ekFBOLD; if (button_get_state(data->italic_check) == ekGUI_ON) fstyle |= ekFITALIC; if (button_get_state(data->under_check) == ekGUI_ON) fstyle |= ekFUNDERLINE; if (button_get_state(data->strike_check) == ekGUI_ON) fstyle |= ekFSTRIKEOUT; color = i_COLORS[popup_get_selected(data->color_popup)]; back = i_COLORS[popup_get_selected(data->back_popup)]; /* Set textview params */ textview_family(data->text, ffamily); textview_fsize(data->text, fsize); textview_fstyle(data->text, fstyle); textview_color(data->text, color); textview_bgcolor(data->text, back); } /*---------------------------------------------------------------------------*/ static void i_OnSetParams(EditData *data, Event *e) { i_set_params(data); unref(e); } /*---------------------------------------------------------------------------*/ static PopUp *i_font_popup(EditData *data) { PopUp *popup = popup_create(); Font *sfont = font_system(15, 0); const char_t *fname = font_family(sfont); uint32_t sel = UINT32_MAX; uint32_t arial = UINT32_MAX; cassert_no_null(data); data->fonts = font_installed_families(); arrpt_foreach_const(font, data->fonts, String) popup_add_elem(popup, tc(font), NULL); if (str_equ(font, fname) == TRUE) sel = font_i; if (str_equ_nocase(tc(font), "Arial") == TRUE) arial = font_i; arrpt_end() if (sel != UINT32_MAX) popup_selected(popup, sel); else if (arial != UINT32_MAX) popup_selected(popup, arial); else popup_selected(popup, 0); popup_list_height(popup, 20); popup_OnSelect(popup, listener(data, i_OnSetParams, EditData)); font_destroy(&sfont); return popup; } /*---------------------------------------------------------------------------*/ static PopUp *i_font_size(EditData *data) { PopUp *popup = popup_create(); uint32_t i = 0; for (i = 10; i <= 30; ++i) { char_t buf[32]; bstd_sprintf(buf, sizeof(buf), "%d", i); popup_add_elem(popup, buf, NULL); } popup_OnSelect(popup, listener(data, i_OnSetParams, EditData)); return popup; } /*---------------------------------------------------------------------------*/ static PopUp *i_font_color(EditData *data) { PopUp *popup = popup_create(); popup_add_elem(popup, "Default", NULL); popup_add_elem(popup, "Black", NULL); popup_add_elem(popup, "White", NULL); popup_add_elem(popup, "Red", NULL); popup_add_elem(popup, "Green", NULL); popup_add_elem(popup, "Blue", NULL); popup_add_elem(popup, "Yellow", NULL); popup_add_elem(popup, "Cyan", NULL); popup_add_elem(popup, "Magenta", NULL); popup_OnSelect(popup, listener(data, i_OnSetParams, EditData)); return popup; } /*---------------------------------------------------------------------------*/ static Layout *i_font_style(EditData *data) { Layout *layout = layout_create(1, 4); Button *button1 = button_check(); Button *button2 = button_check(); Button *button3 = button_check(); Button *button4 = button_check(); cassert_no_null(data); button_text(button1, "Bold"); button_text(button2, "Italic"); button_text(button3, "Underline"); button_text(button4, "Strikeout"); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 0, 1); layout_button(layout, button3, 0, 2); layout_button(layout, button4, 0, 3); button_OnClick(button1, listener(data, i_OnSetParams, EditData)); button_OnClick(button2, listener(data, i_OnSetParams, EditData)); button_OnClick(button3, listener(data, i_OnSetParams, EditData)); button_OnClick(button4, listener(data, i_OnSetParams, EditData)); data->bold_check = button1; data->italic_check = button2; data->under_check = button3; data->strike_check = button4; return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_text_controls(EditData *data) { Layout *layout1 = layout_create(5, 2); Layout *layout2 = i_font_style(data); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); PopUp *popup1 = i_font_popup(data); PopUp *popup2 = i_font_size(data); PopUp *popup3 = i_font_color(data); PopUp *popup4 = i_font_color(data); label_text(label1, "Font family"); label_text(label2, "Font size"); label_text(label3, "Font style"); label_text(label4, "Font color"); label_text(label5, "Back color"); popup_selected(popup2, 5); layout_label(layout1, label1, 0, 0); layout_label(layout1, label2, 1, 0); layout_label(layout1, label3, 2, 0); layout_label(layout1, label4, 3, 0); layout_label(layout1, label5, 4, 0); layout_popup(layout1, popup1, 0, 1); layout_popup(layout1, popup2, 1, 1); layout_layout(layout1, layout2, 2, 1); layout_popup(layout1, popup3, 3, 1); layout_popup(layout1, popup4, 4, 1); layout_hmargin(layout1, 0, 5); layout_hmargin(layout1, 1, 5); layout_hmargin(layout1, 2, 5); layout_hmargin(layout1, 3, 5); layout_vmargin(layout1, 0, 5); layout_valign(layout1, 0, 1, ekTOP); layout_valign(layout1, 1, 1, ekTOP); layout_valign(layout1, 2, 1, ekTOP); layout_valign(layout1, 3, 1, ekTOP); layout_valign(layout1, 4, 1, ekTOP); data->family_popup = popup1; data->size_popup = popup2; data->color_popup = popup3; data->back_popup = popup4; return layout1; } /*---------------------------------------------------------------------------*/ static void i_OnInsertText(EditData *data, Event *e) { cassert_no_null(data); textview_cpos_printf(data->text, "%s", "ins"); unref(e); } /*---------------------------------------------------------------------------*/ static void i_OnAddText(EditData *data, Event *e) { cassert_no_null(data); textview_printf(data->text, "%s", "add"); unref(e); } /*---------------------------------------------------------------------------*/ static void i_apply_params(EditData *data, const bool_t apply_all) { /* Apply the format */ if (apply_all == TRUE) textview_apply_all(data->text); else textview_apply_select(data->text); } /*---------------------------------------------------------------------------*/ static void i_OnApplyAll(EditData *data, Event *e) { i_apply_params(data, TRUE); unref(e); } /*---------------------------------------------------------------------------*/ static void i_OnApplySel(EditData *data, Event *e) { i_apply_params(data, FALSE); unref(e); } /*---------------------------------------------------------------------------*/ static Layout *i_apply_buttons(EditData *data) { Layout *layout = layout_create(5, 1); Label *label = label_create(); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); label_text(label, "Apply format"); button_text(button1, "Insert text"); button_text(button2, "Add text"); button_text(button3, "All text"); button_text(button4, "Selected text"); button_OnClick(button1, listener(data, i_OnInsertText, EditData)); button_OnClick(button2, listener(data, i_OnAddText, EditData)); button_OnClick(button3, listener(data, i_OnApplyAll, EditData)); button_OnClick(button4, listener(data, i_OnApplySel, EditData)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 1, 0); layout_label(layout, label, 2, 0); layout_button(layout, button3, 3, 0); layout_button(layout, button4, 4, 0); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 10); layout_hmargin(layout, 2, 5); layout_hmargin(layout, 3, 5); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_layout(EditData *data) { Layout *layout1 = layout_create(1, 3); Layout *layout2 = i_text_controls(data); Layout *layout3 = i_apply_buttons(data); TextView *text = textview_create(); cassert_no_null(data); textview_editable(text, TRUE); textview_show_select(text, TRUE); layout_layout(layout1, layout2, 0, 0); layout_textview(layout1, text, 0, 1); layout_layout(layout1, layout3, 0, 2); layout_halign(layout1, 0, 2, ekRIGHT); layout_vmargin(layout1, 0, 10); layout_vmargin(layout1, 1, 10); layout_tabstop(layout1, 0, 1, TRUE); data->text = text; return layout1; } /*---------------------------------------------------------------------------*/ static EditData *i_edit_data(Window *window) { EditData *data = heap_new0(EditData); data->window = window; return data; } /*---------------------------------------------------------------------------*/ static void i_colors(void) { i_COLORS[0] = kCOLOR_DEFAULT; i_COLORS[1] = kCOLOR_BLACK; i_COLORS[2] = kCOLOR_WHITE; i_COLORS[3] = kCOLOR_RED; i_COLORS[4] = kCOLOR_GREEN; i_COLORS[5] = kCOLOR_BLUE; i_COLORS[6] = kCOLOR_YELLOW; i_COLORS[7] = kCOLOR_CYAN; i_COLORS[8] = kCOLOR_MAGENTA; } /*---------------------------------------------------------------------------*/ Panel *editor(Window *window) { EditData *data = i_edit_data(window); Layout *layout = i_layout(data); Panel *panel = panel_create(); i_colors(); i_set_params(data); i_apply_params(data, TRUE); panel_data(panel, &data, i_destroy_data, EditData); panel_layout(panel, layout); return panel; } |
7. Hello ListBox!
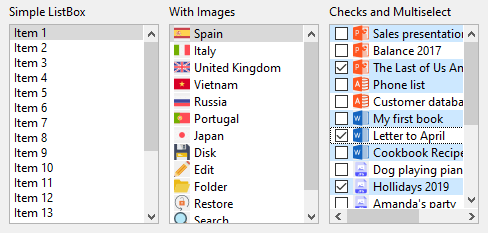
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 |
/* Listboxes */ #include "listboxes.h" #include "res_guihello.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static ListBox *i_full_listbox(void) { ListBox *listbox = listbox_create(); listbox_size(listbox, s2df(150, 200)); listbox_multisel(listbox, TRUE); listbox_checkbox(listbox, TRUE); listbox_add_elem(listbox, "Sales presentation", gui_image(POWERPOINT_PNG)); listbox_add_elem(listbox, "Balance 2017", gui_image(POWERPOINT_PNG)); listbox_add_elem(listbox, "The Last of Us Analysis", gui_image(POWERPOINT_PNG)); listbox_add_elem(listbox, "Phone list", gui_image(ACCESS_PNG)); listbox_add_elem(listbox, "Customer database", gui_image(ACCESS_PNG)); listbox_add_elem(listbox, "My first book", gui_image(WORD_PNG)); listbox_add_elem(listbox, "Letter to April", gui_image(WORD_PNG)); listbox_add_elem(listbox, "Cookbook Recipes", gui_image(WORD_PNG)); listbox_add_elem(listbox, "Dog playing piano", gui_image(JPG_PNG)); listbox_add_elem(listbox, "Hollidays 2019", gui_image(JPG_PNG)); listbox_add_elem(listbox, "Amanda's party", gui_image(JPG_PNG)); listbox_add_elem(listbox, "Flying", gui_image(JPG_PNG)); listbox_add_elem(listbox, "The C Programing Language", gui_image(PDF_PNG)); listbox_add_elem(listbox, "Graphics Programing with GDI+", gui_image(PDF_PNG)); listbox_add_elem(listbox, "Personal finances", gui_image(EXCEL_PNG)); listbox_add_elem(listbox, "Stocks 2017", gui_image(EXCEL_PNG)); listbox_add_elem(listbox, "Website Dashboard", gui_image(EXCEL_PNG)); listbox_add_elem(listbox, "Open Issues", gui_image(DOCUMENT_PNG)); listbox_add_elem(listbox, "TODO List", gui_image(DOCUMENT_PNG)); listbox_select(listbox, 0, TRUE); return listbox; } /*---------------------------------------------------------------------------*/ static ListBox *i_image_listbox(void) { ListBox *listbox = listbox_create(); listbox_size(listbox, s2df(150, 200)); listbox_add_elem(listbox, "Spain", gui_image(SPAIN_PNG)); listbox_add_elem(listbox, "Italy", gui_image(ITALY_PNG)); listbox_add_elem(listbox, "United Kingdom", gui_image(UKING_PNG)); listbox_add_elem(listbox, "Vietnam", gui_image(VIETNAM_PNG)); listbox_add_elem(listbox, "Russia", gui_image(RUSSIA_PNG)); listbox_add_elem(listbox, "Portugal", gui_image(PORTUGAL_PNG)); listbox_add_elem(listbox, "Japan", gui_image(JAPAN_PNG)); listbox_add_elem(listbox, "Disk", gui_image(DISK16_PNG)); listbox_add_elem(listbox, "Edit", gui_image(EDIT16_PNG)); listbox_add_elem(listbox, "Folder", gui_image(FOLDER16_PNG)); listbox_add_elem(listbox, "Restore", gui_image(RESTORE16_PNG)); listbox_add_elem(listbox, "Search", gui_image(SEARCH16_PNG)); listbox_add_elem(listbox, "Error", gui_image(ERROR16_PNG)); listbox_select(listbox, 0, TRUE); return listbox; } /*---------------------------------------------------------------------------*/ static ListBox *i_simple_listbox(void) { ListBox *listbox = listbox_create(); listbox_size(listbox, s2df(150, 200)); listbox_add_elem(listbox, "Item 1", NULL); listbox_add_elem(listbox, "Item 2", NULL); listbox_add_elem(listbox, "Item 3", NULL); listbox_add_elem(listbox, "Item 4", NULL); listbox_color(listbox, 0, gui_alt_color(color_rgb(128, 0, 0), kCOLOR_RED)); listbox_color(listbox, 1, gui_alt_color(color_rgb(0, 128, 0), kCOLOR_GREEN)); listbox_color(listbox, 2, gui_alt_color(color_rgb(0, 0, 128), kCOLOR_BLUE)); listbox_select(listbox, 0, TRUE); return listbox; } /*---------------------------------------------------------------------------*/ Panel *listboxes(void) { Panel *panel = panel_create(); Layout *layout = layout_create(3, 2); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); ListBox *listbox1 = i_simple_listbox(); ListBox *listbox2 = i_image_listbox(); ListBox *listbox3 = i_full_listbox(); label_text(label1, "Simple ListBox"); label_text(label2, "With Images"); label_text(label3, "Checks and Multiselect"); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 1, 0); layout_label(layout, label3, 2, 0); layout_listbox(layout, listbox1, 0, 1); layout_listbox(layout, listbox2, 1, 1); layout_listbox(layout, listbox3, 2, 1); layout_hmargin(layout, 0, 10); layout_hmargin(layout, 1, 10); layout_vmargin(layout, 0, 5); panel_layout(panel, layout); return panel; } |
8. Hello Slider and Progress!
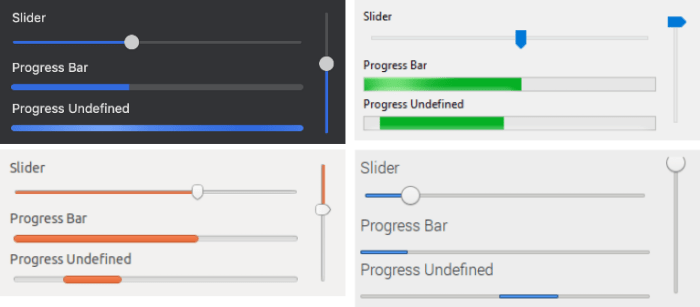
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
/* Sliders */ #include "sliders.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static void i_OnSlider(Progress *prog, Event *event) { const EvSlider *params = event_params(event, EvSlider); progress_value(prog, params->pos); } /*---------------------------------------------------------------------------*/ Panel *sliders(void) { Layout *layout1 = layout_create(2, 1); Layout *layout2 = layout_create(1, 8); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Slider *slider1 = slider_create(); Slider *slider2 = slider_create(); Slider *slider3 = slider_vertical(); Progress *prog1 = progress_create(); Progress *prog2 = progress_create(); Panel *panel = panel_create(); label_text(label1, "Slider"); label_text(label2, "Slider (discrete 6 steps)"); label_text(label3, "Progress Bar"); label_text(label4, "Progress Undefined"); slider_steps(slider2, 6); slider_tooltip(slider1, "Horizontal Slider"); slider_tooltip(slider2, "Horizontal Discrete Slider"); slider_tooltip(slider3, "Vertical Slider"); slider_OnMoved(slider1, listener(prog1, i_OnSlider, Progress)); progress_undefined(prog2, TRUE); layout_label(layout2, label1, 0, 0); layout_label(layout2, label2, 0, 2); layout_label(layout2, label3, 0, 4); layout_label(layout2, label4, 0, 6); layout_slider(layout2, slider1, 0, 1); layout_slider(layout2, slider2, 0, 3); layout_slider(layout1, slider3, 1, 0); layout_progress(layout2, prog1, 0, 5); layout_progress(layout2, prog2, 0, 7); layout_hsize(layout2, 0, 300); layout_layout(layout1, layout2, 0, 0); layout_vmargin(layout2, 0, 5); layout_vmargin(layout2, 1, 5); layout_vmargin(layout2, 2, 5); layout_vmargin(layout2, 3, 5); layout_vmargin(layout2, 4, 5); layout_vmargin(layout2, 5, 5); layout_vmargin(layout2, 6, 5); layout_hmargin(layout1, 0, 10); panel_layout(panel, layout1); return panel; } |
9. Hello TextView!
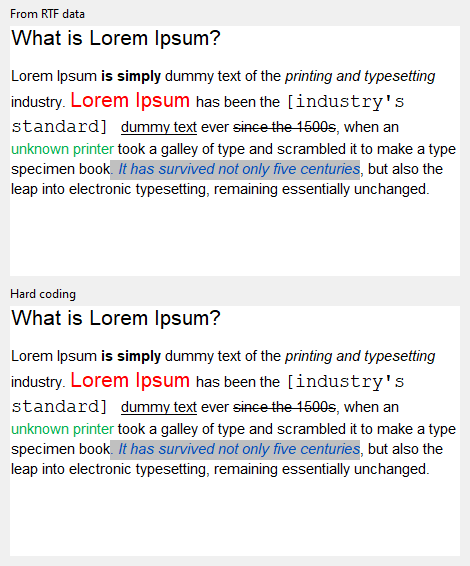
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 |
/* Use of textviews */ #include "textviews.h" #include "res_guihello.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static void i_set_rtf(TextView *text) { ResPack *pack = res_guihello_respack(""); uint32_t size = 0; const byte_t *data = respack_file(pack, TEXTVIEW_RTF, &size); Stream *stm = stm_from_block(data, size); textview_rtf(text, stm); stm_close(&stm); respack_destroy(&pack); } /*---------------------------------------------------------------------------*/ static void i_set_hard_coding(TextView *text) { textview_units(text, ekFPOINTS); textview_lspacing(text, 1.15f); textview_afspace(text, 10); textview_family(text, "Arial"); textview_fsize(text, 16); textview_writef(text, "What is Lorem Ipsum?\n"); textview_fsize(text, 11); textview_writef(text, "Lorem Ipsum "); textview_fstyle(text, ekFBOLD); textview_writef(text, "is simply"); textview_fstyle(text, ekFNORMAL); textview_writef(text, " dummy text of the "); textview_fstyle(text, ekFITALIC); textview_writef(text, "printing and typesetting "); textview_fstyle(text, ekFNORMAL); textview_writef(text, "industry. "); textview_fsize(text, 16); textview_color(text, color_rgb(255, 0, 0)); textview_writef(text, "Lorem Ipsum "); textview_color(text, kCOLOR_DEFAULT); textview_fsize(text, 11); textview_writef(text, "has been the "); textview_family(text, "Courier New"); textview_fsize(text, 14); textview_writef(text, "[industry's standard] "); textview_family(text, "Arial"); textview_fsize(text, 11); textview_fstyle(text, ekFUNDERLINE); textview_writef(text, "dummy text"); textview_fstyle(text, ekFNORMAL); textview_writef(text, " ever "); textview_fstyle(text, ekFSTRIKEOUT); textview_writef(text, "since the 1500s"); textview_fstyle(text, ekFNORMAL); textview_writef(text, ", when an "); textview_color(text, color_rgb(0, 176, 80)); textview_writef(text, "unknown printer "); textview_color(text, kCOLOR_DEFAULT); textview_writef(text, "took a galley of type and scrambled it to make a type specimen book"); textview_fstyle(text, ekFITALIC); textview_color(text, color_rgb(0, 77, 187)); textview_bgcolor(text, color_rgb(192, 192, 192)); textview_writef(text, ". It has survived not only five centuries"); textview_fstyle(text, ekFNORMAL); textview_color(text, kCOLOR_DEFAULT); textview_bgcolor(text, kCOLOR_DEFAULT); textview_writef(text, ", but also the leap into electronic typesetting, remaining essentially unchanged."); } /*---------------------------------------------------------------------------*/ Panel *textviews(void) { Layout *layout = layout_create(1, 4); Label *label1 = label_create(); Label *label2 = label_create(); TextView *text1 = textview_create(); TextView *text2 = textview_create(); Panel *panel = panel_create(); label_text(label1, "From RTF data"); label_text(label2, "Hard coding"); textview_size(text1, s2df(450, 250)); textview_size(text2, s2df(450, 250)); i_set_rtf(text1); i_set_hard_coding(text2); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 0, 2); layout_textview(layout, text1, 0, 1); layout_textview(layout, text2, 0, 3); layout_vmargin(layout, 0, 5); layout_vmargin(layout, 1, 10); layout_vmargin(layout, 2, 5); panel_layout(panel, layout); return panel; } |
10. Hello TableView!
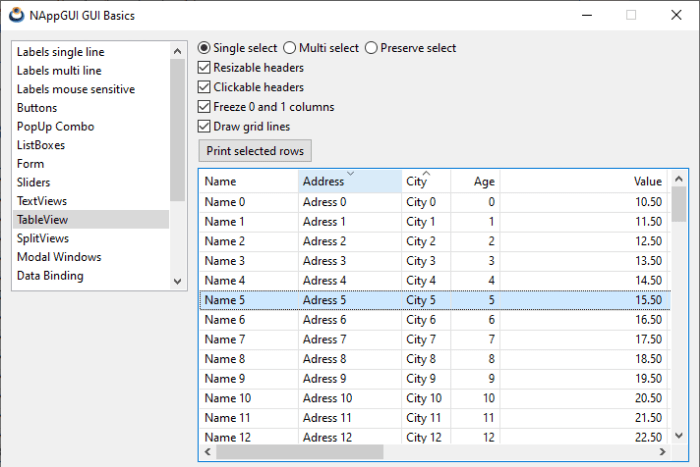
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 |
/* Use of tables */ #include "table.h" #include <gui/guiall.h> typedef struct _appdata_t AppData; struct _appdata_t { TableView *table; TextView *text; char_t temp_string[256]; }; /*---------------------------------------------------------------------------*/ static void i_destroy_appdata(AppData **data) { heap_delete(data, AppData); } /*---------------------------------------------------------------------------*/ /* AppData must contain the real data access(array, stream, etc) */ static void i_OnTableData(AppData *data, Event *e) { uint32_t etype = event_type(e); switch (etype) { case ekGUI_EVENT_TBL_NROWS: { uint32_t *n = event_result(e, uint32_t); *n = 100; break; } case ekGUI_EVENT_TBL_CELL: { const EvTbPos *pos = event_params(e, EvTbPos); EvTbCell *cell = event_result(e, EvTbCell); switch (pos->col) { case 0: cell->align = ekLEFT; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Name %d", pos->row); break; case 1: cell->align = ekLEFT; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Adress %d", pos->row); break; case 2: cell->align = ekLEFT; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "City %d", pos->row); break; case 3: cell->align = ekRIGHT; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "%d", pos->row); break; case 4: cell->align = ekRIGHT; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "%.2f", 10.5f + pos->row); break; case 5: cell->align = ekCENTER; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Extra Data 1 %d", pos->row); break; case 6: cell->align = ekCENTER; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Extra Data 2 %d", pos->row); break; case 7: cell->align = ekCENTER; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Extra Data 3 %d", pos->row); break; case 8: cell->align = ekCENTER; bstd_sprintf(data->temp_string, sizeof(data->temp_string), "Extra Data 4 %d", pos->row); break; cassert_default(); } cell->text = data->temp_string; break; } } } /*---------------------------------------------------------------------------*/ static void i_OnHeaderClick(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); textview_printf(data->text, "Click on Header: %d\n", p->index); } /*---------------------------------------------------------------------------*/ static void i_OnMultisel(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); if (p->index == 0) tableview_multisel(data->table, FALSE, FALSE); else if (p->index == 1) tableview_multisel(data->table, TRUE, FALSE); else if (p->index == 2) tableview_multisel(data->table, TRUE, TRUE); } /*---------------------------------------------------------------------------*/ static void i_OnResizeCheck(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); bool_t resizable = p->state == ekGUI_ON ? TRUE : FALSE; tableview_header_resizable(data->table, resizable); } /*---------------------------------------------------------------------------*/ static void i_OnHeaderCheck(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); bool_t clickable = p->state == ekGUI_ON ? TRUE : FALSE; tableview_header_clickable(data->table, clickable); } /*---------------------------------------------------------------------------*/ static void i_OnFreezeCheck(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); uint32_t col_freeze = p->state == ekGUI_ON ? 1 : UINT32_MAX; tableview_column_freeze(data->table, col_freeze); } /*---------------------------------------------------------------------------*/ static void i_OnGridCheck(AppData *data, Event *e) { const EvButton *p = event_params(e, EvButton); bool_t grid = p->state == ekGUI_ON ? TRUE : FALSE; tableview_grid(data->table, grid, grid); } /*---------------------------------------------------------------------------*/ static void i_OnPrintsel(AppData *data, Event *e) { const ArrSt(uint32_t) *sel = tableview_selected(data->table); uint32_t n = arrst_size(sel, uint32_t); textview_writef(data->text, "Selected rows: "); arrst_foreach_const(row, sel, uint32_t) textview_printf(data->text, "%d", *row); if (row_i < n - 1) textview_writef(data->text, ", "); arrst_end() textview_writef(data->text, "\n"); unref(e); } /*---------------------------------------------------------------------------*/ static Layout *i_table_control_layout(AppData *data) { Layout *layout1 = layout_create(3, 1); Layout *layout2 = layout_create(1, 6); Button *button1 = button_radio(); Button *button2 = button_radio(); Button *button3 = button_radio(); Button *button4 = button_check(); Button *button5 = button_check(); Button *button6 = button_check(); Button *button7 = button_check(); Button *button8 = button_push(); button_text(button1, "Single select"); button_text(button2, "Multi select"); button_text(button3, "Preserve select"); button_text(button4, "Resizable headers"); button_text(button5, "Clickable headers"); button_text(button6, "Freeze 0 and 1 columns"); button_text(button7, "Draw grid lines"); button_text(button8, "Print selected rows"); button_state(button1, ekGUI_ON); button_state(button4, ekGUI_ON); button_state(button5, ekGUI_ON); button_state(button6, ekGUI_ON); button_state(button7, ekGUI_ON); layout_button(layout1, button1, 0, 0); layout_button(layout1, button2, 1, 0); layout_button(layout1, button3, 2, 0); layout_layout(layout2, layout1, 0, 0); layout_button(layout2, button4, 0, 1); layout_button(layout2, button5, 0, 2); layout_button(layout2, button6, 0, 3); layout_button(layout2, button7, 0, 4); layout_button(layout2, button8, 0, 5); layout_hmargin(layout1, 0, 5.f); layout_hmargin(layout1, 1, 5.f); layout_vmargin(layout2, 0, 5.f); layout_vmargin(layout2, 1, 5.f); layout_vmargin(layout2, 2, 5.f); layout_vmargin(layout2, 3, 5.f); layout_vmargin(layout2, 4, 5.f); layout_halign(layout2, 0, 0, ekLEFT); layout_halign(layout2, 0, 5, ekLEFT); button_OnClick(button1, listener(data, i_OnMultisel, AppData)); button_OnClick(button2, listener(data, i_OnMultisel, AppData)); button_OnClick(button3, listener(data, i_OnMultisel, AppData)); button_OnClick(button4, listener(data, i_OnResizeCheck, AppData)); button_OnClick(button5, listener(data, i_OnHeaderCheck, AppData)); button_OnClick(button6, listener(data, i_OnFreezeCheck, AppData)); button_OnClick(button7, listener(data, i_OnGridCheck, AppData)); button_OnClick(button8, listener(data, i_OnPrintsel, AppData)); return layout2; } /*---------------------------------------------------------------------------*/ Panel *table_view(void) { Panel *panel = panel_create(); AppData *data = heap_new0(AppData); TableView *table = tableview_create(); TextView *text = textview_create(); Layout *layout1 = layout_create(1, 3); Layout *layout2 = i_table_control_layout(data); data->table = table; data->text = text; tableview_size(table, s2df(500, 300)); tableview_OnData(table, listener(data, i_OnTableData, AppData)); tableview_OnHeaderClick(table, listener(data, i_OnHeaderClick, AppData)); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_new_column_text(table); tableview_header_clickable(table, TRUE); tableview_header_resizable(table, TRUE); tableview_header_indicator(table, 1, ekINDDOWN_ARROW); tableview_header_indicator(table, 2, ekINDUP_ARROW); tableview_header_title(table, 0, "Name"); tableview_header_title(table, 1, "Address"); tableview_header_title(table, 2, "City"); tableview_header_title(table, 3, "Age"); tableview_header_title(table, 4, "Value"); tableview_header_title(table, 5, "Extra\nData 1"); tableview_header_title(table, 6, "Extra\nData 2"); tableview_header_title(table, 7, "Extra\nData 3"); tableview_header_title(table, 8, "Extra\nData 4"); tableview_column_width(table, 0, 100); tableview_column_width(table, 1, 105); tableview_column_width(table, 2, 50); tableview_column_width(table, 3, 50); tableview_column_width(table, 4, 170); tableview_column_width(table, 5, 200); tableview_column_width(table, 6, 200); tableview_column_width(table, 7, 200); tableview_column_width(table, 8, 200); tableview_column_limits(table, 2, 50, 100); tableview_column_freeze(table, 1); tableview_header_align(table, 0, ekLEFT); tableview_header_align(table, 1, ekLEFT); tableview_header_align(table, 2, ekLEFT); tableview_header_align(table, 3, ekRIGHT); tableview_header_align(table, 4, ekRIGHT); tableview_header_align(table, 5, ekCENTER); tableview_header_align(table, 6, ekCENTER); tableview_header_align(table, 7, ekCENTER); tableview_header_align(table, 8, ekCENTER); tableview_multisel(table, FALSE, FALSE); tableview_header_visible(table, TRUE); tableview_grid(table, TRUE, TRUE); tableview_update(table); { uint32_t row = 20; tableview_select(table, &row, 1); tableview_focus_row(table, row, ekBOTTOM); } layout_layout(layout1, layout2, 0, 0); layout_tableview(layout1, table, 0, 1); layout_textview(layout1, text, 0, 2); layout_vmargin(layout1, 0, 5.f); layout_vmargin(layout1, 1, 5.f); panel_data(panel, &data, i_destroy_appdata, AppData); panel_layout(panel, layout1); return panel; } |
11. Hello SplitView!
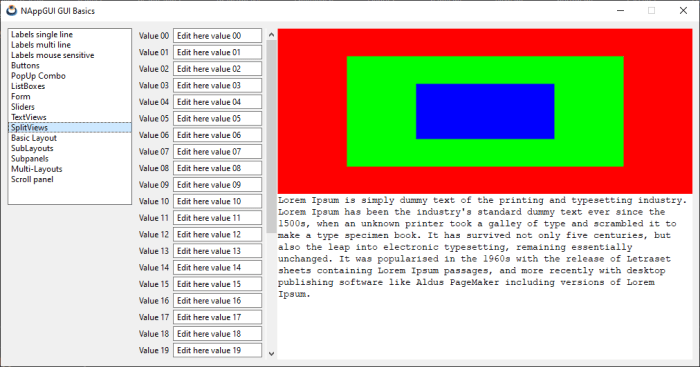
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
/* Use of splitviews */ #include "splits.h" #include <gui/guiall.h> static const char_t *i_LOREM = "Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum."; /*---------------------------------------------------------------------------*/ static void i_OnDraw(View *view, Event *e) { const EvDraw *p = event_params(e, EvDraw); real32_t p0 = p->width / 6; real32_t p1 = p->height / 6; real32_t p2 = p->width / 3; real32_t p3 = p->height / 3; unref(view); draw_fill_color(p->ctx, kCOLOR_RED); draw_rect(p->ctx, ekFILL, 0, 0, p->width, p->height); draw_fill_color(p->ctx, kCOLOR_GREEN); draw_rect(p->ctx, ekFILL, p0, p1, p->width - 2 * p0, p->height - 2 * p1); draw_fill_color(p->ctx, kCOLOR_BLUE); draw_rect(p->ctx, ekFILL, p2, p3, p->width - 2 * p2, p->height - 2 * p3); } /*---------------------------------------------------------------------------*/ static Panel *i_left_panel(void) { uint32_t i, n = 32; Panel *panel = panel_scroll(FALSE, TRUE); Layout *layout = layout_create(2, n); real32_t rmargin = panel_scroll_width(panel); for (i = 0; i < n; ++i) { char_t text[64]; Label *label = label_create(); Edit *edit = edit_create(); bstd_sprintf(text, sizeof(text), "Value %02d", i); label_text(label, text); bstd_sprintf(text, sizeof(text), "Edit here value %02d", i); edit_text(edit, text); layout_label(layout, label, 0, i); layout_edit(layout, edit, 1, i); } for (i = 0; i < n - 1; ++i) layout_vmargin(layout, i, 3); layout_hmargin(layout, 0, 5); layout_margin4(layout, 0, rmargin, 0, 0); layout_hexpand(layout, 1); panel_layout(panel, layout); return panel; } /*---------------------------------------------------------------------------*/ Panel *split_panel(void) { Panel *panel1 = panel_create(); Panel *panel2 = i_left_panel(); Layout *layout = layout_create(1, 1); SplitView *split1 = splitview_vertical(); SplitView *split2 = splitview_horizontal(); TextView *text = textview_create(); View *view = view_create(); textview_writef(text, i_LOREM); view_size(view, s2df(400, 200)); textview_size(text, s2df(400, 200)); view_OnDraw(view, listener(view, i_OnDraw, View)); splitview_view(split2, view, FALSE); splitview_textview(split2, text, FALSE); splitview_panel(split1, panel2); splitview_splitview(split1, split2); layout_splitview(layout, split1, 0, 0); panel_layout(panel1, layout); return panel1; } |
12. Hello Modal Window!
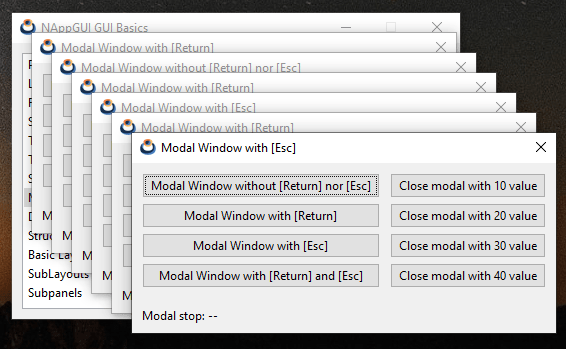
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 |
/* Listboxes */ #include "modalwin.h" #include <gui/guiall.h> typedef struct _modal_data_t ModalData; struct _modal_data_t { uint32_t type; Label *label; Window *parent; }; /*---------------------------------------------------------------------------*/ static const char_t *i_MODAL0 = "Modal Window without [Return] nor [Esc]"; static const char_t *i_MODAL1 = "Modal Window with [Return]"; static const char_t *i_MODAL2 = "Modal Window with [Esc]"; static const char_t *i_MODAL3 = "Modal Window with [Return] and [Esc]"; /*---------------------------------------------------------------------------*/ static Layout *i_modal_layout(ModalData *data); /*---------------------------------------------------------------------------*/ static ModalData* i_modal_data(Window* parent) { ModalData *data = heap_new0(ModalData); data->parent = parent; data->type = UINT32_MAX; return data; } /*---------------------------------------------------------------------------*/ static void i_destroy_modal_data(ModalData** data) { heap_delete(data, ModalData); } /*---------------------------------------------------------------------------*/ static void i_OnCloseModal(Window* window, Event* e) { Button *button = event_sender(e, Button); window_stop_modal(window, button_get_tag(button)); } /*---------------------------------------------------------------------------*/ static Layout* i_close_layout(Window *window) { Layout *layout = layout_create(1, 4); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); button_text(button1, "Close modal with 10 value"); button_text(button2, "Close modal with 20 value"); button_text(button3, "Close modal with 30 value"); button_text(button4, "Close modal with 40 value"); button_tag(button1, 10); button_tag(button2, 20); button_tag(button3, 30); button_tag(button4, 40); button_OnClick(button1, listener(window, i_OnCloseModal, Window)); button_OnClick(button2, listener(window, i_OnCloseModal, Window)); button_OnClick(button3, listener(window, i_OnCloseModal, Window)); button_OnClick(button4, listener(window, i_OnCloseModal, Window)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 0, 1); layout_button(layout, button3, 0, 2); layout_button(layout, button4, 0, 3); layout_vmargin(layout, 0, 5); layout_vmargin(layout, 1, 5); layout_vmargin(layout, 2, 5); return layout; } /*---------------------------------------------------------------------------*/ static uint32_t i_window_flags(const uint32_t type) { uint32_t flags = ekWINDOW_TITLE | ekWINDOW_CLOSE; switch(type) { case 0: return flags; case 1: return flags | ekWINDOW_RETURN; case 2: return flags | ekWINDOW_ESC; case 3: return flags | ekWINDOW_RETURN | ekWINDOW_ESC; cassert_default(); } return 0; } /*---------------------------------------------------------------------------*/ static const char_t *i_window_title(const uint32_t type) { switch(type) { case 0: return i_MODAL0; case 1: return i_MODAL1; case 2: return i_MODAL2; case 3: return i_MODAL3; cassert_default(); } return 0; } /*---------------------------------------------------------------------------*/ static void i_modal_window(ModalData *data) { uint32_t flags = i_window_flags(data->type); Window *window = window_create(flags); ModalData *ndata = i_modal_data(window); Panel *panel = panel_create(); Layout *layout1 = layout_create(2, 1); Layout *layout2 = i_modal_layout(ndata); Layout *layout3 = i_close_layout(window); uint32_t retval = UINT32_MAX; V2Df pos = window_get_origin(data->parent); char_t text[128]; layout_layout(layout1, layout2, 0, 0); layout_layout(layout1, layout3, 1, 0); layout_hmargin(layout1, 0, 10); layout_valign(layout1, 1, 0, ekTOP); layout_margin(layout1, 10); panel_data(panel, &ndata, i_destroy_modal_data, ModalData); panel_layout(panel, layout1); window_panel(window, panel); window_title(window, i_window_title(data->type)); window_origin(window, v2df(pos.x + 20, pos.y + 20)); retval = window_modal(window, data->parent); if (retval == (uint32_t)ekGUI_CLOSE_ESC) bstd_sprintf(text, sizeof(text), "Modal stop: [Esc] (%d)", retval); else if (retval == (uint32_t)ekGUI_CLOSE_INTRO) bstd_sprintf(text, sizeof(text), "Modal stop: [Return] (%d)", retval); else if (retval == (uint32_t)ekGUI_CLOSE_BUTTON) bstd_sprintf(text, sizeof(text), "Modal stop: [X] (%d)", retval); else bstd_sprintf(text, sizeof(text), "Modal stop: %d", retval); label_text(data->label, text); window_destroy(&window); } /*---------------------------------------------------------------------------*/ static void i_OnClickModal(ModalData* data, Event* e) { Button *button = event_sender(e, Button); data->type = button_get_tag(button); i_modal_window(data); } /*---------------------------------------------------------------------------*/ static Layout *i_modal_layout(ModalData *data) { Layout *layout = layout_create(1, 5); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); Label *label = label_create(); cassert(data->label == NULL); data->label = label; button_text(button1, i_MODAL0); button_text(button2, i_MODAL1); button_text(button3, i_MODAL2); button_text(button4, i_MODAL3); label_text(label, "Modal stop: --"); button_tag(button1, 0); button_tag(button2, 1); button_tag(button3, 2); button_tag(button4, 3); button_OnClick(button1, listener(data, i_OnClickModal, ModalData)); button_OnClick(button2, listener(data, i_OnClickModal, ModalData)); button_OnClick(button3, listener(data, i_OnClickModal, ModalData)); button_OnClick(button4, listener(data, i_OnClickModal, ModalData)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 0, 1); layout_button(layout, button3, 0, 2); layout_button(layout, button4, 0, 3); layout_label(layout, label, 0, 4); layout_halign(layout, 0, 4, ekJUSTIFY); layout_vmargin(layout, 0, 5); layout_vmargin(layout, 1, 5); layout_vmargin(layout, 2, 5); layout_vmargin(layout, 3, 20); return layout; } /*---------------------------------------------------------------------------*/ Panel *modal_windows(Window *parent) { Panel *panel = panel_create(); ModalData *data = i_modal_data(parent); Layout *layout = i_modal_layout(data); panel_layout(panel, layout); panel_data(panel, &data, i_destroy_modal_data, ModalData); return panel; } |
13. Hello Overlay Window!
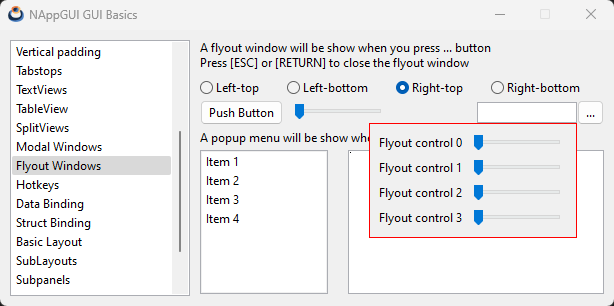
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 |
/* Flyout window */ #include "flyout.h" #include <gui/guiall.h> typedef struct _flyout_t FlyOut; struct _flyout_t { Window *parent; Window *flywin; Menu *menu; TextView *text; GuiControl *edit; uint32_t align; }; /*---------------------------------------------------------------------------*/ static void i_destroy_flyout(FlyOut **flyout) { cassert_no_null(flyout); cassert_no_null(*flyout); window_destroy(&(*flyout)->flywin); if ((*flyout)->menu != NULL) menu_destroy(&(*flyout)->menu); heap_delete(flyout, FlyOut); } /*---------------------------------------------------------------------------*/ static Window *i_create_flywin(void) { uint32_t nrows = 4; Layout *layout = layout_create(2, nrows); Panel *panel = panel_create(); Window *window = window_create(ekWINDOW_RETURN | ekWINDOW_ESC); uint32_t i; for (i = 0; i < nrows; ++i) { char_t text[64]; Label *label = label_create(); Slider *slider = slider_create(); bstd_sprintf(text, sizeof(text), "Flyout control %d", i); label_text(label, text); layout_label(layout, label, 0, i); layout_slider(layout, slider, 1, i); if (i < nrows - 1) layout_vmargin(layout, i, 5); } layout_hmargin(layout, 0, 5); layout_margin(layout, 10); layout_skcolor(layout, kCOLOR_RED); panel_layout(panel, layout); window_panel(window, panel); return window; } /*---------------------------------------------------------------------------*/ static void i_OnIdleLaunch(FlyOut *flyout, Event *e) { /* Edit control bounds in window coordinates */ R2Df frame = window_control_frame(flyout->parent, flyout->edit); /* Top-Left edit control in screen coordinates */ V2Df pos = window_client_to_screen(flyout->parent, frame.pos); /* Flyout window size */ S2Df size = window_get_size(flyout->flywin); switch (flyout->align) { case 0: pos.y += frame.size.height; break; case 1: pos.y -= size.height; break; case 2: pos.x -= size.width - frame.size.width; pos.y += frame.size.height; break; case 3: pos.x -= size.width - frame.size.width; pos.y -= size.height; break; } /* Position in screen coordinates */ window_origin(flyout->flywin, pos); window_overlay(flyout->flywin, flyout->parent); unref(e); } /*---------------------------------------------------------------------------*/ static void i_OnClick(FlyOut *flyout, Event *e) { gui_OnIdle(listener(flyout, i_OnIdleLaunch, FlyOut)); unref(e); } /*---------------------------------------------------------------------------*/ static Layout *i_controls_layout(FlyOut *flyout) { Layout *layout = layout_create(5, 1); Button *button1 = button_push(); Button *button2 = button_push(); Slider *slider = slider_create(); Edit *edit = edit_create(); button_text(button1, "Push Button"); button_text(button2, "..."); button_OnClick(button2, listener(flyout, i_OnClick, FlyOut)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 4, 0); layout_slider(layout, slider, 1, 0); layout_edit(layout, edit, 3, 0); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_hexpand(layout, 2); flyout->edit = guicontrol(edit); return layout; } /*---------------------------------------------------------------------------*/ static void i_OnAlign(FlyOut *flyout, Event *e) { const EvButton *p = event_params(e, EvButton); cassert_no_null(flyout); flyout->align = p->index; } /*---------------------------------------------------------------------------*/ static Layout *i_align_layout(FlyOut *flyout) { Layout *layout = layout_create(4, 1); Button *button1 = button_radio(); Button *button2 = button_radio(); Button *button3 = button_radio(); Button *button4 = button_radio(); button_text(button1, "Left-top"); button_text(button2, "Left-bottom"); button_text(button3, "Right-top"); button_text(button4, "Right-bottom"); button_OnClick(button1, listener(flyout, i_OnAlign, FlyOut)); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 1, 0); layout_button(layout, button3, 2, 0); layout_button(layout, button4, 3, 0); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_hmargin(layout, 2, 5); button_state(button1, ekGUI_ON); return layout; } /*---------------------------------------------------------------------------*/ static void i_OnMenu(FlyOut *flyout, Event *e) { const EvMenu *p = event_params(e, EvMenu); textview_writef(flyout->text, p->text); textview_writef(flyout->text, "\n"); } /*---------------------------------------------------------------------------*/ static void i_OnDown(FlyOut *flyout, Event *e) { const EvMouse *p = event_params(e, EvMouse); if (p->button == ekGUI_MOUSE_RIGHT && p->tag != UINT32_MAX) { uint32_t i = 0; if (flyout->menu != NULL) menu_destroy(&flyout->menu); flyout->menu = menu_create(); for (i = 0; i < 4; ++i) { char_t text[64]; MenuItem *item = menuitem_create(); bstd_sprintf(text, sizeof(text), "Item %d Option %d", p->tag + 1, i + 1); menuitem_text(item, text); menuitem_OnClick(item, listener(flyout, i_OnMenu, FlyOut)); menu_add_item(flyout->menu, item); } { V2Df pos = gui_mouse_pos(); menu_launch(flyout->menu, flyout->parent, pos); } } } /*---------------------------------------------------------------------------*/ static Layout *i_listbox_layout(FlyOut *flyout) { Layout *layout = layout_create(2, 1); ListBox *list = listbox_create(); TextView *text = textview_create(); listbox_add_elem(list, "Item 1", NULL); listbox_add_elem(list, "Item 2", NULL); listbox_add_elem(list, "Item 3", NULL); listbox_add_elem(list, "Item 4", NULL); listbox_OnDown(list, listener(flyout, i_OnDown, FlyOut)); layout_listbox(layout, list, 0, 0); layout_textview(layout, text, 1, 0); layout_hmargin(layout, 0, 20); flyout->text = text; return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_layout(FlyOut *flyout) { Layout *layout1 = layout_create(1, 5); Layout *layout2 = i_align_layout(flyout); Layout *layout3 = i_controls_layout(flyout); Layout *layout4 = i_listbox_layout(flyout); Label *label1 = label_create(); Label *label2 = label_create(); label_multiline(label1, TRUE); label_text(label1, "A flyout window will be show when you press ... button\nPress [ESC] or [RETURN] to close the flyout window"); label_text(label2, "A popup menu will be show when right click in ListBox"); layout_label(layout1, label1, 0, 0); layout_layout(layout1, layout2, 0, 1); layout_layout(layout1, layout3, 0, 2); layout_label(layout1, label2, 0, 3); layout_layout(layout1, layout4, 0, 4); layout_vmargin(layout1, 0, 10); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 2, 5); layout_vmargin(layout1, 3, 5); return layout1; } /*---------------------------------------------------------------------------*/ static FlyOut *i_flyout(Window *window) { FlyOut *flyout = heap_new(FlyOut); flyout->parent = window; flyout->flywin = i_create_flywin(); flyout->menu = NULL; flyout->align = 0; return flyout; } /*---------------------------------------------------------------------------*/ Panel *flyout_window(Window *window) { FlyOut *flyout = i_flyout(window); Layout *layout = i_layout(flyout); Panel *panel = panel_create(); panel_data(panel, &flyout, i_destroy_flyout, FlyOut); panel_layout(panel, layout); return panel; } |
14. Hello Gui Binding!
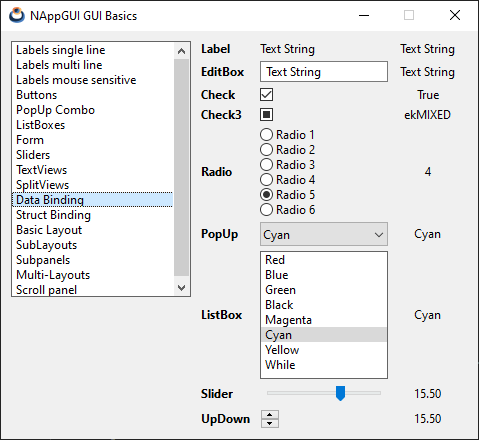
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 |
/* GUI data binding */ #include "guibind.h" #include <gui/guiall.h> typedef struct _basictypes_t BasicTypes; typedef enum _myenum_t { ekRED, ekBLUE, ekGREEN, ekBLACK, ekMAGENTA, ekCYAN, ekYELLOW, ekWHITE } myenum_t; struct _basictypes_t { bool_t bool_val; uint16_t uint16_val; real32_t real32_val; myenum_t enum_val; gui_state_t enum3_val; String *str_val; }; #define i_NUM_CONTROLS 9 /*---------------------------------------------------------------------------*/ static void i_data_bind(void) { dbind_enum(gui_state_t, ekGUI_OFF, ""); dbind_enum(gui_state_t, ekGUI_ON, ""); dbind_enum(gui_state_t, ekGUI_MIXED, ""); dbind_enum(myenum_t, ekRED, "Red"); dbind_enum(myenum_t, ekBLUE, "Blue"); dbind_enum(myenum_t, ekGREEN, "Green"); dbind_enum(myenum_t, ekBLACK, "Black"); dbind_enum(myenum_t, ekMAGENTA, "Magenta"); dbind_enum(myenum_t, ekCYAN, "Cyan"); dbind_enum(myenum_t, ekYELLOW, "Yellow"); dbind_enum(myenum_t, ekWHITE, "While"); dbind(BasicTypes, bool_t, bool_val); dbind(BasicTypes, uint16_t, uint16_val); dbind(BasicTypes, real32_t, real32_val); dbind(BasicTypes, gui_state_t, enum3_val); dbind(BasicTypes, myenum_t, enum_val); dbind(BasicTypes, String *, str_val); dbind_range(BasicTypes, real32_t, real32_val, -50, 50); dbind_increment(BasicTypes, real32_t, real32_val, 5); } /*---------------------------------------------------------------------------*/ static void i_destroy_data(BasicTypes **data) { str_destroy(&(*data)->str_val); heap_delete(data, BasicTypes); dbind_unreg(BasicTypes); dbind_unreg(myenum_t); } /*---------------------------------------------------------------------------*/ static Layout *i_radio_layout(void) { uint32_t i = 0, n = 6; Layout *layout = layout_create(1, n); for (i = 0; i < n; ++i) { Button *radio = button_radio(); char_t str[64]; bstd_sprintf(str, sizeof(str), "Radio %d", i + 1); button_text(radio, str); layout_button(layout, radio, 0, i); } return layout; } /*---------------------------------------------------------------------------*/ static void i_title_labels(Layout *layout) { Font *font = font_system(font_regular_size(), ekFBOLD); const char_t *strs[] = {"Label", "EditBox", "Check", "Check3", "Radio", "PopUp", "ListBox", "Slider", "UpDown"}; uint32_t i = 0; for (i = 0; i < i_NUM_CONTROLS; ++i) { Label *label = label_create(); label_text(label, strs[i]); label_font(label, font); layout_label(layout, label, 0, i); } layout_hmargin(layout, 0, 10); font_destroy(&font); } /*---------------------------------------------------------------------------*/ static void i_value_labels(Layout *layout) { uint32_t i = 0; for (i = 0; i < i_NUM_CONTROLS; ++i) { Label *label = label_create(); label_align(label, ekCENTER); layout_label(layout, label, 2, i); layout_halign(layout, 2, i, ekJUSTIFY); } layout_hsize(layout, 2, 80); layout_hmargin(layout, 0, 10); for (i = 0; i < i_NUM_CONTROLS - 1; ++i) layout_vmargin(layout, i, 5); cell_dbind(layout_cell(layout, 2, 0), BasicTypes, String *, str_val); cell_dbind(layout_cell(layout, 2, 1), BasicTypes, String *, str_val); cell_dbind(layout_cell(layout, 2, 2), BasicTypes, bool_t, bool_val); cell_dbind(layout_cell(layout, 2, 3), BasicTypes, gui_state_t, enum3_val); cell_dbind(layout_cell(layout, 2, 4), BasicTypes, uint16_t, uint16_val); cell_dbind(layout_cell(layout, 2, 5), BasicTypes, myenum_t, enum_val); cell_dbind(layout_cell(layout, 2, 6), BasicTypes, myenum_t, enum_val); cell_dbind(layout_cell(layout, 2, 7), BasicTypes, real32_t, real32_val); cell_dbind(layout_cell(layout, 2, 8), BasicTypes, real32_t, real32_val); } /*---------------------------------------------------------------------------*/ static Layout *i_layout(void) { Layout *layout = layout_create(3, 9); Label *label = label_create(); Edit *edit = edit_create(); Button *check = button_check(); Button *check3 = button_check3(); Layout *radios = i_radio_layout(); PopUp *popup = popup_create(); ListBox *listbox = listbox_create(); Slider *slider = slider_create(); UpDown *updown = updown_create(); layout_label(layout, label, 1, 0); layout_edit(layout, edit, 1, 1); layout_button(layout, check, 1, 2); layout_button(layout, check3, 1, 3); layout_layout(layout, radios, 1, 4); layout_popup(layout, popup, 1, 5); layout_listbox(layout, listbox, 1, 6); layout_slider(layout, slider, 1, 7); layout_updown(layout, updown, 1, 8); layout_halign(layout, 1, 0, ekJUSTIFY); layout_halign(layout, 1, 8, ekLEFT); cell_dbind(layout_cell(layout, 1, 0), BasicTypes, String *, str_val); cell_dbind(layout_cell(layout, 1, 1), BasicTypes, String *, str_val); cell_dbind(layout_cell(layout, 1, 2), BasicTypes, bool_t, bool_val); cell_dbind(layout_cell(layout, 1, 3), BasicTypes, gui_state_t, enum3_val); cell_dbind(layout_cell(layout, 1, 4), BasicTypes, uint16_t, uint16_val); cell_dbind(layout_cell(layout, 1, 5), BasicTypes, myenum_t, enum_val); cell_dbind(layout_cell(layout, 1, 6), BasicTypes, myenum_t, enum_val); cell_dbind(layout_cell(layout, 1, 7), BasicTypes, real32_t, real32_val); cell_dbind(layout_cell(layout, 1, 8), BasicTypes, real32_t, real32_val); i_title_labels(layout); i_value_labels(layout); return layout; } /*---------------------------------------------------------------------------*/ Panel *guibind(void) { Layout *layout = NULL; Panel *panel = NULL; BasicTypes *data = heap_new(BasicTypes); i_data_bind(); layout = i_layout(); panel = panel_create(); panel_layout(panel, layout); data->bool_val = TRUE; data->uint16_val = 4; data->real32_val = 15.5f; data->enum3_val = ekGUI_MIXED; data->enum_val = ekCYAN; data->str_val = str_c("Text String"); layout_dbind(layout, NULL, BasicTypes); layout_dbind_obj(layout, data, BasicTypes); panel_data(panel, &data, i_destroy_data, BasicTypes); return panel; } |
15. Hello Struct Binding!
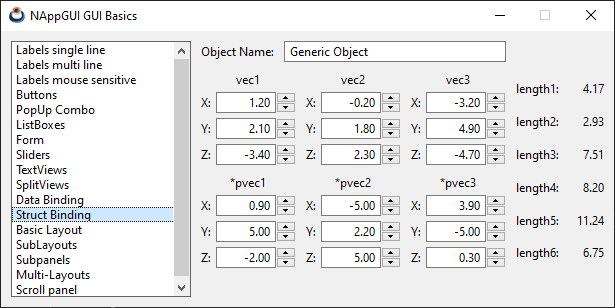
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 |
/* GUI data binding */ #include "layoutbind.h" #include <gui/guiall.h> typedef struct _vector_t Vector; typedef struct _structtypes_t StructTypes; struct _vector_t { real32_t x; real32_t y; real32_t z; }; struct _structtypes_t { String *name; Vector vec1; Vector vec2; Vector vec3; Vector *pvec1; Vector *pvec2; Vector *pvec3; real32_t length1; real32_t length2; real32_t length3; real32_t length4; real32_t length5; real32_t length6; }; /*---------------------------------------------------------------------------*/ static void i_data_bind(void) { dbind(Vector, real32_t, x); dbind(Vector, real32_t, y); dbind(Vector, real32_t, z); dbind(StructTypes, String *, name); dbind(StructTypes, Vector, vec1); dbind(StructTypes, Vector, vec2); dbind(StructTypes, Vector, vec3); dbind(StructTypes, Vector *, pvec1); dbind(StructTypes, Vector *, pvec2); dbind(StructTypes, Vector *, pvec3); dbind(StructTypes, real32_t, length1); dbind(StructTypes, real32_t, length2); dbind(StructTypes, real32_t, length3); dbind(StructTypes, real32_t, length4); dbind(StructTypes, real32_t, length5); dbind(StructTypes, real32_t, length6); dbind_range(Vector, real32_t, x, -5, 5); dbind_range(Vector, real32_t, y, -5, 5); dbind_range(Vector, real32_t, z, -5, 5); dbind_increment(Vector, real32_t, x, .1f); dbind_increment(Vector, real32_t, y, .1f); dbind_increment(Vector, real32_t, z, .1f); } /*---------------------------------------------------------------------------*/ static void i_destroy_data(StructTypes **data) { str_destroy(&(*data)->name); heap_delete(&(*data)->pvec1, Vector); heap_delete(&(*data)->pvec2, Vector); heap_delete(&(*data)->pvec3, Vector); heap_delete(data, StructTypes); dbind_unreg(StructTypes); dbind_unreg(Vector); } /*---------------------------------------------------------------------------*/ static Vector i_vec_init(const real32_t x, const real32_t y, const real32_t z) { Vector v; v.x = x; v.y = y; v.z = z; return v; } /*---------------------------------------------------------------------------*/ static real32_t i_vec_length(const Vector *vec) { real32_t n = vec->x * vec->x + vec->y * vec->y + vec->z * vec->z; return bmath_sqrtf(n); } /*---------------------------------------------------------------------------*/ static void i_OnDataChange(void *non_used, Event *e) { StructTypes *data = evbind_object(e, StructTypes); Layout *layout = event_sender(e, Layout); unref(non_used); if (evbind_modify(e, StructTypes, Vector, vec1) == TRUE) { data->length1 = i_vec_length(&data->vec1); layout_dbind_update(layout, StructTypes, real32_t, length1); } else if (evbind_modify(e, StructTypes, Vector, vec2) == TRUE) { data->length2 = i_vec_length(&data->vec2); layout_dbind_update(layout, StructTypes, real32_t, length2); } else if (evbind_modify(e, StructTypes, Vector, vec3) == TRUE) { data->length3 = i_vec_length(&data->vec3); layout_dbind_update(layout, StructTypes, real32_t, length3); } else if (evbind_modify(e, StructTypes, Vector *, pvec1) == TRUE) { data->length4 = i_vec_length(data->pvec1); layout_dbind_update(layout, StructTypes, real32_t, length4); } else if (evbind_modify(e, StructTypes, Vector *, pvec2) == TRUE) { data->length5 = i_vec_length(data->pvec2); layout_dbind_update(layout, StructTypes, real32_t, length5); } else if (evbind_modify(e, StructTypes, Vector *, pvec3) == TRUE) { data->length6 = i_vec_length(data->pvec3); layout_dbind_update(layout, StructTypes, real32_t, length6); } } /*---------------------------------------------------------------------------*/ static Layout *i_vector_layout(void) { Layout *layout = layout_create(3, 3); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); Edit *edit3 = edit_create(); UpDown *updown1 = updown_create(); UpDown *updown2 = updown_create(); UpDown *updown3 = updown_create(); label_text(label1, "X:"); label_text(label2, "Y:"); label_text(label3, "Z:"); edit_align(edit1, ekRIGHT); edit_align(edit2, ekRIGHT); edit_align(edit3, ekRIGHT); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 0, 1); layout_label(layout, label3, 0, 2); layout_edit(layout, edit1, 1, 0); layout_edit(layout, edit2, 1, 1); layout_edit(layout, edit3, 1, 2); layout_updown(layout, updown1, 2, 0); layout_updown(layout, updown2, 2, 1); layout_updown(layout, updown3, 2, 2); layout_hmargin(layout, 0, 5); layout_vmargin(layout, 0, 5); layout_vmargin(layout, 1, 5); layout_hsize(layout, 1, 60); cell_dbind(layout_cell(layout, 1, 0), Vector, real32_t, x); cell_dbind(layout_cell(layout, 1, 1), Vector, real32_t, y); cell_dbind(layout_cell(layout, 1, 2), Vector, real32_t, z); cell_dbind(layout_cell(layout, 2, 0), Vector, real32_t, x); cell_dbind(layout_cell(layout, 2, 1), Vector, real32_t, y); cell_dbind(layout_cell(layout, 2, 2), Vector, real32_t, z); layout_dbind(layout, NULL, Vector); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_name_layout(void) { Layout *layout = layout_create(2, 1); Label *label = label_create(); Edit *edit = edit_create(); label_text(label, "Object Name:"); layout_hexpand(layout, 1); layout_label(layout, label, 0, 0); layout_edit(layout, edit, 1, 0); layout_hmargin(layout, 0, 10); cell_dbind(layout_cell(layout, 1, 0), StructTypes, String *, name); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_vectors_layout(void) { Layout *layout1 = layout_create(3, 4); Layout *layout2 = i_vector_layout(); Layout *layout3 = i_vector_layout(); Layout *layout4 = i_vector_layout(); Layout *layout5 = i_vector_layout(); Layout *layout6 = i_vector_layout(); Layout *layout7 = i_vector_layout(); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); label_text(label1, "vec1"); label_text(label2, "vec2"); label_text(label3, "vec3"); label_text(label4, "*pvec1"); label_text(label5, "*pvec2"); label_text(label6, "*pvec3"); layout_label(layout1, label1, 0, 0); layout_label(layout1, label2, 1, 0); layout_label(layout1, label3, 2, 0); layout_label(layout1, label4, 0, 2); layout_label(layout1, label5, 1, 2); layout_label(layout1, label6, 2, 2); layout_layout(layout1, layout2, 0, 1); layout_layout(layout1, layout3, 1, 1); layout_layout(layout1, layout4, 2, 1); layout_layout(layout1, layout5, 0, 3); layout_layout(layout1, layout6, 1, 3); layout_layout(layout1, layout7, 2, 3); layout_halign(layout1, 0, 0, ekCENTER); layout_halign(layout1, 1, 0, ekCENTER); layout_halign(layout1, 2, 0, ekCENTER); layout_halign(layout1, 0, 2, ekCENTER); layout_halign(layout1, 1, 2, ekCENTER); layout_halign(layout1, 2, 2, ekCENTER); layout_hmargin(layout1, 0, 10); layout_hmargin(layout1, 1, 10); layout_vmargin(layout1, 0, 5); layout_vmargin(layout1, 1, 10); layout_vmargin(layout1, 2, 5); cell_dbind(layout_cell(layout1, 0, 1), StructTypes, Vector, vec1); cell_dbind(layout_cell(layout1, 1, 1), StructTypes, Vector, vec2); cell_dbind(layout_cell(layout1, 2, 1), StructTypes, Vector, vec3); cell_dbind(layout_cell(layout1, 0, 3), StructTypes, Vector *, pvec1); cell_dbind(layout_cell(layout1, 1, 3), StructTypes, Vector *, pvec2); cell_dbind(layout_cell(layout1, 2, 3), StructTypes, Vector *, pvec3); return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_lengths_layout(void) { Layout *layout = layout_create(2, 6); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); Label *label7 = label_create(); Label *label8 = label_create(); Label *label9 = label_create(); Label *label10 = label_create(); Label *label11 = label_create(); Label *label12 = label_create(); label_text(label1, "length1:"); label_text(label2, "length2:"); label_text(label3, "length3:"); label_text(label4, "length4:"); label_text(label5, "length5:"); label_text(label6, "length6:"); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 0, 1); layout_label(layout, label3, 0, 2); layout_label(layout, label4, 0, 3); layout_label(layout, label5, 0, 4); layout_label(layout, label6, 0, 5); layout_label(layout, label7, 1, 0); layout_label(layout, label8, 1, 1); layout_label(layout, label9, 1, 2); layout_label(layout, label10, 1, 3); layout_label(layout, label11, 1, 4); layout_label(layout, label12, 1, 5); label_align(label7, ekRIGHT); label_align(label8, ekRIGHT); label_align(label9, ekRIGHT); label_align(label10, ekRIGHT); label_align(label11, ekRIGHT); label_align(label12, ekRIGHT); layout_hsize(layout, 1, 40); layout_hmargin(layout, 0, 5); layout_halign(layout, 1, 0, ekJUSTIFY); layout_halign(layout, 1, 1, ekJUSTIFY); layout_halign(layout, 1, 2, ekJUSTIFY); layout_halign(layout, 1, 3, ekJUSTIFY); layout_halign(layout, 1, 4, ekJUSTIFY); layout_halign(layout, 1, 5, ekJUSTIFY); cell_dbind(layout_cell(layout, 1, 0), StructTypes, real32_t, length1); cell_dbind(layout_cell(layout, 1, 1), StructTypes, real32_t, length2); cell_dbind(layout_cell(layout, 1, 2), StructTypes, real32_t, length3); cell_dbind(layout_cell(layout, 1, 3), StructTypes, real32_t, length4); cell_dbind(layout_cell(layout, 1, 4), StructTypes, real32_t, length5); cell_dbind(layout_cell(layout, 1, 5), StructTypes, real32_t, length6); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_layout(void) { Layout *layout1 = layout_create(2, 2); Layout *layout2 = i_name_layout(); Layout *layout3 = i_vectors_layout(); Layout *layout4 = i_lengths_layout(); layout_layout(layout1, layout2, 0, 0); layout_layout(layout1, layout3, 0, 1); layout_layout(layout1, layout4, 1, 1); layout_hmargin(layout1, 0, 10); layout_vmargin(layout1, 0, 10); return layout1; } /*---------------------------------------------------------------------------*/ Panel *layoutbind(void) { Layout *layout = NULL; Panel *panel = NULL; StructTypes *data = heap_new(StructTypes); i_data_bind(); layout = i_layout(); panel = panel_create(); panel_layout(panel, layout); data->name = str_c("Generic Object"); data->pvec1 = heap_new(Vector); data->pvec2 = heap_new(Vector); data->pvec3 = heap_new(Vector); data->vec1 = i_vec_init(1.2f, 2.1f, -3.4f); data->vec2 = i_vec_init(-0.2f, 1.8f, 2.3f); data->vec3 = i_vec_init(-3.2f, 4.9f, -4.7f); *data->pvec1 = i_vec_init(0.9f, 7.9f, -2.0f); *data->pvec2 = i_vec_init(-6.9f, 2.2f, 8.6f); *data->pvec3 = i_vec_init(3.9f, -5.5f, 0.3f); data->length1 = i_vec_length(&data->vec1); data->length2 = i_vec_length(&data->vec2); data->length3 = i_vec_length(&data->vec3); data->length4 = i_vec_length(data->pvec1); data->length5 = i_vec_length(data->pvec2); data->length6 = i_vec_length(data->pvec3); layout_dbind(layout, listener(NULL, i_OnDataChange, void), StructTypes); layout_dbind_obj(layout, data, StructTypes); panel_data(panel, &data, i_destroy_data, StructTypes); return panel; } |
16. Hello Sublayout!
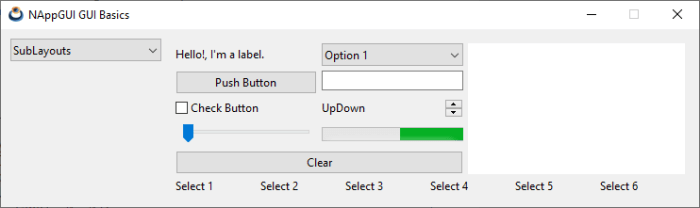
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 |
/* Sublayouts */ #include "sublayout.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static Layout *i_updown_layout(void) { Layout *layout = layout_create(2, 1); Label *label = label_create(); UpDown *updown = updown_create(); label_text(label, "UpDown"); layout_label(layout, label, 0, 0); layout_updown(layout, updown, 1, 0); layout_hexpand(layout, 0); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_left_grid_layout(void) { Layout *layout1 = layout_create(2, 4); Layout *layout2 = i_updown_layout(); Label *label = label_create(); Button *button1 = button_push(); Button *button2 = button_check(); Slider *slider = slider_create(); PopUp *popup = popup_create(); Edit *edit = edit_create(); Progress *progress = progress_create(); label_text(label, "Hello!, I'm a label."); button_text(button1, "Push Button"); button_text(button2, "Check Button"); popup_add_elem(popup, "Option 1", NULL); popup_add_elem(popup, "Option 2", NULL); popup_add_elem(popup, "Option 3", NULL); popup_add_elem(popup, "Option 4", NULL); progress_undefined(progress, TRUE); layout_label(layout1, label, 0, 0); layout_button(layout1, button1, 0, 1); layout_button(layout1, button2, 0, 2); layout_slider(layout1, slider, 0, 3); layout_popup(layout1, popup, 1, 0); layout_edit(layout1, edit, 1, 1); layout_layout(layout1, layout2, 1, 2); layout_progress(layout1, progress, 1, 3); layout_hsize(layout1, 0, 150); layout_hsize(layout1, 1, 150); layout_hmargin(layout1, 0, 5); layout_vmargin(layout1, 0, 5); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 2, 5); return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_left_layout(void) { Layout *layout1 = layout_create(1, 2); Layout *layout2 = i_left_grid_layout(); Button *button = button_push(); button_text(button, "Clear"); layout_layout(layout1, layout2, 0, 0); layout_button(layout1, button, 0, 1); layout_vmargin(layout1, 0, 5); return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_top_layout(void) { Layout *layout1 = layout_create(2, 1); Layout *layout2 = i_left_layout(); TextView *view = textview_create(); layout_layout(layout1, layout2, 0, 0); layout_textview(layout1, view, 1, 0); layout_hsize(layout1, 1, 230); layout_hmargin(layout1, 0, 5); return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_bottom_layout(void) { Layout *layout = layout_create(6, 1); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); label_text(label1, "Select 1"); label_text(label2, "Select 2"); label_text(label3, "Select 3"); label_text(label4, "Select 4"); label_text(label5, "Select 5"); label_text(label6, "Select 6"); label_style_over(label1, ekFUNDERLINE); label_style_over(label2, ekFUNDERLINE); label_style_over(label3, ekFUNDERLINE); label_style_over(label4, ekFUNDERLINE); label_style_over(label5, ekFUNDERLINE); label_style_over(label6, ekFUNDERLINE); layout_label(layout, label1, 0, 0); layout_label(layout, label2, 1, 0); layout_label(layout, label3, 2, 0); layout_label(layout, label4, 3, 0); layout_label(layout, label5, 4, 0); layout_label(layout, label6, 5, 0); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_main_layout(void) { Layout *layout1 = layout_create(1, 2); Layout *layout2 = i_top_layout(); Layout *layout3 = i_bottom_layout(); layout_layout(layout1, layout2, 0, 0); layout_layout(layout1, layout3, 0, 1); layout_margin(layout1, 5); layout_vmargin(layout1, 0, 5); return layout1; } /*---------------------------------------------------------------------------*/ Panel *sublayouts(void) { Panel *panel = panel_create(); Layout *layout = i_main_layout(); panel_layout(panel, layout); return panel; } |
17. Hello Subpanel!
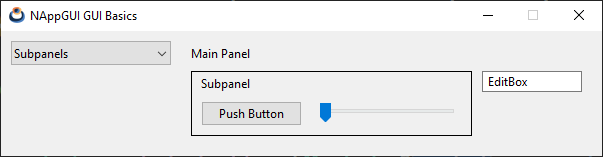
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* Use of subpanels */ #include "subpanel.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ Panel *subpanels(void) { Panel *panel1 = panel_create(); Panel *panel2 = panel_create(); Layout *layout1 = layout_create(2, 2); Layout *layout2 = layout_create(2, 2); Label *label1 = label_create(); Label *label2 = label_create(); Button *button = button_push(); Slider *slider = slider_create(); Edit *edit = edit_create(); label_text(label1, "Main Panel"); label_text(label2, "Subpanel"); button_text(button, "Push Button"); edit_text(edit, "EditBox"); layout_label(layout2, label2, 0, 0); layout_button(layout2, button, 0, 1); layout_slider(layout2, slider, 1, 1); layout_hsize(layout2, 1, 150); layout_hmargin(layout2, 0, 10); layout_vmargin(layout2, 0, 10); layout_margin4(layout2, 5, 10, 10, 10); layout_skcolor(layout2, gui_line_color()); panel_layout(panel2, layout2); layout_label(layout1, label1, 0, 0); layout_edit(layout1, edit, 1, 1); layout_panel(layout1, panel2, 0, 1); layout_hsize(layout1, 1, 100); layout_hmargin(layout1, 0, 10); layout_vmargin(layout1, 0, 10); layout_margin4(layout1, 5, 10, 10, 10); panel_layout(panel1, layout1); return panel1; } |
18. Hello Multi-layout!
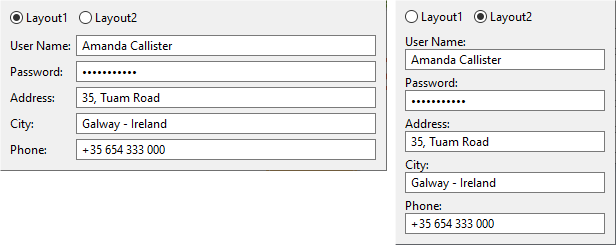
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
/* Panels with multiple layouts */ #include "multilayout.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static Panel *i_multilayout_panel(void) { Panel *panel = panel_create(); Layout *layout1 = layout_create(2, 5); Layout *layout2 = layout_create(1, 10); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); Edit *edit3 = edit_create(); Edit *edit4 = edit_create(); Edit *edit5 = edit_create(); label_text(label1, "User Name:"); label_text(label2, "Password:"); label_text(label3, "Address:"); label_text(label4, "City:"); label_text(label5, "Phone:"); edit_text(edit1, "Amanda Callister"); edit_text(edit2, "aQwe56nhjJk"); edit_text(edit3, "35, Tuam Road"); edit_text(edit4, "Galway - Ireland"); edit_text(edit5, "+35 654 333 000"); edit_passmode(edit2, TRUE); layout_label(layout1, label1, 0, 0); layout_label(layout1, label2, 0, 1); layout_label(layout1, label3, 0, 2); layout_label(layout1, label4, 0, 3); layout_label(layout1, label5, 0, 4); layout_edit(layout1, edit1, 1, 0); layout_edit(layout1, edit2, 1, 1); layout_edit(layout1, edit3, 1, 2); layout_edit(layout1, edit4, 1, 3); layout_edit(layout1, edit5, 1, 4); layout_hsize(layout1, 1, 300); layout_hmargin(layout1, 0, 5); layout_vmargin(layout1, 0, 5); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 2, 5); layout_vmargin(layout1, 3, 5); layout_label(layout2, label1, 0, 0); layout_label(layout2, label2, 0, 2); layout_label(layout2, label3, 0, 4); layout_label(layout2, label4, 0, 6); layout_label(layout2, label5, 0, 8); layout_edit(layout2, edit1, 0, 1); layout_edit(layout2, edit2, 0, 3); layout_edit(layout2, edit3, 0, 5); layout_edit(layout2, edit4, 0, 7); layout_edit(layout2, edit5, 0, 9); layout_hsize(layout2, 0, 200); layout_vmargin(layout2, 1, 5); layout_vmargin(layout2, 3, 5); layout_vmargin(layout2, 5, 5); layout_vmargin(layout2, 7, 5); panel_layout(panel, layout1); panel_layout(panel, layout2); return panel; } /*---------------------------------------------------------------------------*/ static void i_OnLayout(Panel *panel, Event *e) { const EvButton *params = event_params(e, EvButton); panel_visible_layout(panel, params->index); panel_update(panel); } /*---------------------------------------------------------------------------*/ Panel *multilayouts(void) { Panel *panel1 = panel_create(); Panel *panel2 = i_multilayout_panel(); Button *button1 = button_radio(); Button *button2 = button_radio(); Layout *layout1 = layout_create(1, 2); Layout *layout2 = layout_create(2, 1); button_text(button1, "Layout1"); button_text(button2, "Layout2"); button_state(button1, ekGUI_ON); button_OnClick(button1, listener(panel2, i_OnLayout, Panel)); layout_button(layout2, button1, 0, 0); layout_button(layout2, button2, 1, 0); layout_layout(layout1, layout2, 0, 0); layout_panel(layout1, panel2, 0, 1); layout_vmargin(layout1, 0, 10); layout_hmargin(layout2, 0, 10); layout_halign(layout1, 0, 0, ekLEFT); panel_layout(panel1, layout1); return panel1; } |
19. Hello Scroll-Panel!
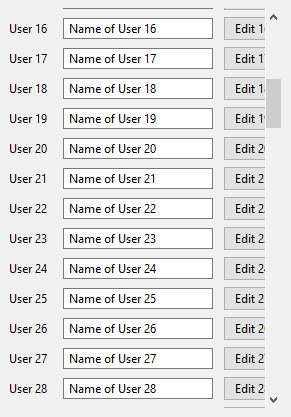
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* Panel with scroll */ #include "scrollpanel.h" #include <gui/guiall.h> static const uint32_t i_ROWS = 100; /*---------------------------------------------------------------------------*/ Panel *scrollpanel(void) { Panel *panel = panel_scroll(FALSE, TRUE); Layout *layout = layout_create(3, i_ROWS); real32_t margin = panel_scroll_width(panel); uint32_t i = 0; panel_size(panel, s2df(-1, 400)); for (i = 0; i < i_ROWS; ++i) { char_t text[128]; Label *label = label_create(); Edit *edit = edit_create(); Button *button = button_push(); bstd_sprintf(text, sizeof(text), "User %d", i + 1); label_text(label, text); bstd_sprintf(text, sizeof(text), "Name of User %d", i + 1); edit_text(edit, text); bstd_sprintf(text, sizeof(text), "Edit %d", i + 1); button_text(button, text); layout_label(layout, label, 0, i); layout_edit(layout, edit, 1, i); layout_button(layout, button, 2, i); } for (i = 0; i < i_ROWS - 1; ++i) layout_vmargin(layout, i, 5); layout_hmargin(layout, 0, 10); layout_hmargin(layout, 1, 10); layout_hsize(layout, 1, 150); layout_margin4(layout, 0, margin, 0, 0); panel_layout(panel, layout); return panel; } |
20. Hello dynamic Layout!
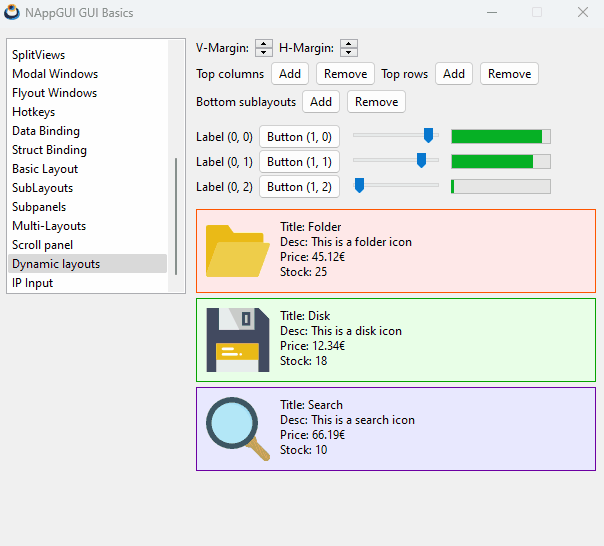
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 |
/* Dynamic layouts */ #include "dynlay.h" #include "res_guihello.h" #include <gui/guiall.h> typedef struct dyn_data_t DynData; struct dyn_data_t { Layout *top_layout; Layout *bottom_layout; uint32_t hmargin; uint32_t vmargin; color_t bgcolor[3]; color_t skcolor[3]; }; /*---------------------------------------------------------------------------*/ static void i_destroy_dyndata(DynData **data) { heap_delete(data, DynData); } /*---------------------------------------------------------------------------*/ static void i_top_layout_margins(DynData *data) { uint32_t i, ncols, nrows; cassert_no_null(data); ncols = layout_ncols(data->top_layout); nrows = layout_nrows(data->top_layout); cassert(ncols >= 2); /* At lest one column and expand column */ cassert(nrows >= 1); /* At lest one row */ if (ncols > 2) { for (i = 0; i < ncols - 2; ++i) layout_hmargin(data->top_layout, i, (real32_t)data->hmargin); } if (nrows > 1) { for (i = 0; i < nrows - 1; ++i) layout_vmargin(data->top_layout, i, (real32_t)data->vmargin); } } /*---------------------------------------------------------------------------*/ static void i_bottom_layout_margins(DynData *data) { uint32_t i, nrows; cassert_no_null(data); nrows = layout_nrows(data->bottom_layout); cassert(nrows >= 1); /* At lest one row */ if (nrows > 1) { for (i = 0; i < nrows - 1; ++i) layout_vmargin(data->bottom_layout, i, 5); } } /*---------------------------------------------------------------------------*/ static void i_OnVMargin(DynData *data, Event *e) { const EvButton *p = event_params(e, EvButton); cassert_no_null(data); if (p->index == 1 && data->vmargin > 0) data->vmargin -= 1; else if (p->index == 0) data->vmargin += 1; i_top_layout_margins(data); layout_update(data->top_layout); } /*---------------------------------------------------------------------------*/ static void i_OnHMargin(DynData *data, Event *e) { const EvButton *p = event_params(e, EvButton); cassert_no_null(data); if (p->index == 1 && data->hmargin > 0) data->hmargin -= 1; else if (p->index == 0) data->hmargin += 1; i_top_layout_margins(data); layout_update(data->top_layout); } /*---------------------------------------------------------------------------*/ static Layout *i_control_layout_1(DynData *data) { Layout *layout = layout_create(5, 1); Label *label1 = label_create(); Label *label2 = label_create(); UpDown *updown1 = updown_create(); UpDown *updown2 = updown_create(); label_text(label1, "V-Margin:"); label_text(label2, "H-Margin:"); updown_OnClick(updown1, listener(data, i_OnVMargin, DynData)); updown_OnClick(updown2, listener(data, i_OnHMargin, DynData)); layout_label(layout, label1, 0, 0); layout_updown(layout, updown1, 1, 0); layout_label(layout, label2, 2, 0); layout_updown(layout, updown2, 3, 0); /* * Static margin between columns */ layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_hmargin(layout, 2, 5); /* * By default, updown and button cells are JUSTIFICABLE * We force keep the buttons into their original size */ layout_halign(layout, 1, 0, ekLEFT); layout_halign(layout, 3, 0, ekLEFT); /* * The horizontal expansion is delegated to a fifth empty cell. * This prevents excess pixels from being distributed across all columns, * keeping the left four columns together. */ layout_hexpand(layout, 4); return layout; } /*---------------------------------------------------------------------------*/ static void i_OnSlider(DynData *data, Event *e) { Slider *slider = event_sender(e, Slider); GuiControl *control = guicontrol(slider); uint32_t tag = guicontrol_get_tag(control); uint32_t col = tag & 0x0000FFFF; uint32_t row = ((tag & 0xFFFF0000) >> 16); uint32_t ncols = layout_ncols(data->top_layout); /* Exists a column at the right of slider */ if (ncols > col + 1) { const EvSlider *p = event_params(e, EvSlider); /* The element at the right of slider is a progress always */ Progress *progress = layout_get_progress(data->top_layout, col + 1, row); /* We syncro the progress with its neighbor slider */ progress_value(progress, p->pos); } } /*---------------------------------------------------------------------------*/ static void i_fill_cell(Layout *layout, const uint32_t col, const uint32_t row, DynData *data) { /* We are sure not to overwrite a cell */ cassert(cell_empty(layout_cell(layout, col, row)) == TRUE); switch (col % 5) { case 0: { Label *label = label_create(); String *text = str_printf("Label (%d, %d)", col, row); label_text(label, tc(text)); layout_label(layout, label, col, row); str_destroy(&text); break; } case 1: { Button *button = button_push(); String *text = str_printf("Button (%d, %d)", col, row); button_text(button, tc(text)); layout_button(layout, button, col, row); str_destroy(&text); break; } case 2: { Slider *slider = slider_create(); GuiControl *control = guicontrol(slider); real32_t pos = bmath_randf(0, 1); uint32_t tag = (row << 16) | col; slider_value(slider, pos); slider_OnMoved(slider, listener(data, i_OnSlider, DynData)); guicontrol_tag(control, tag); layout_slider(layout, slider, col, row); break; } case 3: { /* Progress is in syncro with its left slider */ Progress *progress = progress_create(); Slider *slider = layout_get_slider(layout, col - 1, row); real32_t pos = slider_get_value(slider); progress_value(progress, pos); layout_progress(layout, progress, col, row); break; } case 4: { PopUp *popup = popup_create(); uint32_t i; for (i = 0; i < 5; ++i) { String *text = str_printf("Item (%d, %d) - %d", col, row, i); popup_add_elem(popup, tc(text), NULL); str_destroy(&text); } layout_popup(layout, popup, col, row); break; } } } /*---------------------------------------------------------------------------*/ static void i_fill_row(Layout *layout, const uint32_t row, DynData *data) { uint32_t i, cols = layout_ncols(layout); cassert(cols > 1); for (i = 0; i < cols - 1; ++i) i_fill_cell(layout, i, row, data); } /*---------------------------------------------------------------------------*/ static void i_fill_col(Layout *layout, const uint32_t col, DynData *data) { uint32_t i, rows = layout_nrows(layout); cassert(rows > 1); for (i = 0; i < rows; ++i) i_fill_cell(layout, col, i, data); } /*---------------------------------------------------------------------------*/ static const Image *i_image(const uint32_t row) { switch (row % 6) { case 0: return gui_image(FOLDER64_PNG); case 1: return gui_image(DISK64_PNG); case 2: return gui_image(SEARCH64_PNG); case 3: return gui_image(EDIT64_PNG); case 4: return gui_image(PLUS64_PNG); case 5: return gui_image(ERROR64_PNG); } return NULL; } /*---------------------------------------------------------------------------*/ static const char_t *i_title_text(const uint32_t row) { switch (row % 6) { case 0: return "Title: Folder"; case 1: return "Title: Disk"; case 2: return "Title: Search"; case 3: return "Title: Edit"; case 4: return "Title: Plus"; case 5: return "Title: Error"; } return NULL; } /*---------------------------------------------------------------------------*/ static const char_t *i_desc_text(const uint32_t row) { switch (row % 6) { case 0: return "Desc: This is a folder icon"; case 1: return "Desc: This is a disk icon"; case 2: return "Desc: This is a search icon"; case 3: return "Desc: This is a edit icon"; case 4: return "Desc: This is a plus icon"; case 5: return "Desc: This is a error icon"; } return NULL; } /*---------------------------------------------------------------------------*/ static const char_t *i_price_text(const uint32_t row) { switch (row % 6) { case 0: return "Price: 45.12€"; case 1: return "Price: 12.34€"; case 2: return "Price: 66.19€"; case 3: return "Price: 22.65€"; case 4: return "Price: 99.99€"; case 5: return "Price: 32.56€"; } return NULL; } /*---------------------------------------------------------------------------*/ static const char_t *i_stock_text(const uint32_t row) { switch (row % 6) { case 0: return "Stock: 25"; case 1: return "Stock: 18"; case 2: return "Stock: 10"; case 3: return "Stock: 22"; case 4: return "Stock: 7"; case 5: return "Stock: 0"; } return NULL; } /*---------------------------------------------------------------------------*/ static void i_fill_sublayout(Layout *layout, const uint32_t row, DynData *data) { /* * layout1 is a new row-sublayout added to bottom_layout * composed by tree cells: * Image cell * Data cell: vertical sublayout with 4 labels in a stack * Expand cell: Only for expand the excess of pixels of wider sublayout (top_layout) */ Layout *layout1 = layout_create(3, 1); Layout *layout2 = layout_create(1, 4); ImageView *view = imageview_create(); const Image *image = i_image(row); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); /* We are sure not to overwrite a cell */ cassert(cell_empty(layout_cell(layout, 0, row)) == TRUE); imageview_image(view, image); layout_imageview(layout1, view, 0, 0); label_text(label1, i_title_text(row)); label_text(label2, i_desc_text(row)); label_text(label3, i_price_text(row)); label_text(label4, i_stock_text(row)); layout_label(layout2, label1, 0, 0); layout_label(layout2, label2, 0, 1); layout_label(layout2, label3, 0, 2); layout_label(layout2, label4, 0, 3); layout_layout(layout1, layout2, 1, 0); layout_valign(layout1, 0, 0, ekTOP); layout_valign(layout1, 1, 0, ekTOP); layout_hmargin(layout1, 0, 10); layout_hexpand(layout1, 2); layout_margin(layout1, 10); layout_bgcolor(layout1, data->bgcolor[row % 3]); layout_skcolor(layout1, data->skcolor[row % 3]); layout_layout(layout, layout1, 0, row); } /*---------------------------------------------------------------------------*/ static void i_OnTopAddCol(DynData *data, Event *e) { uint32_t ncols = 0; cassert_no_null(data); ncols = layout_ncols(data->top_layout); cassert(ncols > 1); unref(e); /* Insert new column in penultimate position. The last is the empty-resizable column */ /* Because empty cells with 0-margin are added, the visual appearance does not change after insert */ layout_insert_col(data->top_layout, ncols - 1); /* Add the new widget to recent-created cells */ i_fill_col(data->top_layout, ncols - 1, data); /* Update the margins, because the new column has 0-margin */ i_top_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->top_layout); } /*---------------------------------------------------------------------------*/ static void i_OnTopDelCol(DynData *data, Event *e) { uint32_t ncols = 0; cassert_no_null(data); ncols = layout_ncols(data->top_layout); unref(e); if (ncols > 3) { layout_remove_col(data->top_layout, ncols - 2); /* Update the margins, because the new column has 0-margin */ i_top_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->top_layout); } } /*---------------------------------------------------------------------------*/ static void i_OnTopAddRow(DynData *data, Event *e) { uint32_t nrows = 0; cassert_no_null(data); nrows = layout_nrows(data->top_layout); cassert(nrows >= 1); unref(e); /* Insert new row in last position */ /* Because empty cells with 0-margin are added, the visual appearance does not change after insert */ layout_insert_row(data->top_layout, nrows); /* Add the new widget to recent-created cells */ i_fill_row(data->top_layout, nrows, data); /* Update the margins, because the new row has 0-margin */ i_top_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->top_layout); } /*---------------------------------------------------------------------------*/ static void i_OnTopDelRow(DynData *data, Event *e) { uint32_t nrows = 0; cassert_no_null(data); nrows = layout_nrows(data->top_layout); unref(e); if (nrows > 2) { layout_remove_row(data->top_layout, nrows - 1); /* Update the margins, because the new column has 0-margin */ i_top_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->top_layout); } } /*---------------------------------------------------------------------------*/ static void i_OnTopAddLayout(DynData *data, Event *e) { uint32_t nrows = 0; cassert_no_null(data); nrows = layout_nrows(data->bottom_layout); cassert(nrows >= 1); unref(e); /* Insert new row in last position */ layout_insert_row(data->bottom_layout, nrows); /* Add a new sublayout to last cell */ i_fill_sublayout(data->bottom_layout, nrows, data); /* Update the margins, because the new row has 0-margin */ i_bottom_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->bottom_layout); } /*---------------------------------------------------------------------------*/ static void i_OnTopDelLayout(DynData *data, Event *e) { uint32_t nrows = 0; cassert_no_null(data); nrows = layout_nrows(data->bottom_layout); unref(e); if (nrows > 1) { /* Remove the row in last position */ layout_remove_row(data->bottom_layout, nrows - 1); /* Update the margins, because the new row has 0-margin */ i_bottom_layout_margins(data); /* Recompute the layout appearance and update widgets */ layout_update(data->bottom_layout); } } /*---------------------------------------------------------------------------*/ static Layout *i_control_layout_2(DynData *data) { Layout *layout = layout_create(7, 1); Label *label1 = label_create(); Label *label2 = label_create(); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); label_text(label1, "Top columns"); label_text(label2, "Top rows"); button_text(button1, "Add"); button_text(button2, "Remove"); button_text(button3, "Add"); button_text(button4, "Remove"); button_OnClick(button1, listener(data, i_OnTopAddCol, DynData)); button_OnClick(button2, listener(data, i_OnTopDelCol, DynData)); button_OnClick(button3, listener(data, i_OnTopAddRow, DynData)); button_OnClick(button4, listener(data, i_OnTopDelRow, DynData)); layout_label(layout, label1, 0, 0); layout_button(layout, button1, 1, 0); layout_button(layout, button2, 2, 0); layout_label(layout, label2, 3, 0); layout_button(layout, button3, 4, 0); layout_button(layout, button4, 5, 0); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_hmargin(layout, 2, 5); layout_hmargin(layout, 3, 5); layout_hmargin(layout, 4, 5); layout_halign(layout, 1, 0, ekLEFT); layout_halign(layout, 2, 0, ekLEFT); layout_halign(layout, 4, 0, ekLEFT); layout_halign(layout, 5, 0, ekLEFT); layout_hexpand(layout, 6); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_control_layout_3(DynData *data) { Layout *layout = layout_create(4, 1); Label *label = label_create(); Button *button1 = button_push(); Button *button2 = button_push(); label_text(label, "Bottom sublayouts"); button_text(button1, "Add"); button_text(button2, "Remove"); button_OnClick(button1, listener(data, i_OnTopAddLayout, DynData)); button_OnClick(button2, listener(data, i_OnTopDelLayout, DynData)); layout_label(layout, label, 0, 0); layout_button(layout, button1, 1, 0); layout_button(layout, button2, 2, 0); layout_hmargin(layout, 0, 5); layout_hmargin(layout, 1, 5); layout_halign(layout, 1, 0, ekLEFT); layout_halign(layout, 2, 0, ekLEFT); layout_hexpand(layout, 3); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_control_layout(DynData *data) { Layout *layout = layout_create(1, 3); Layout *layout1 = i_control_layout_1(data); Layout *layout2 = i_control_layout_2(data); Layout *layout3 = i_control_layout_3(data); layout_layout(layout, layout1, 0, 0); layout_layout(layout, layout2, 0, 1); layout_layout(layout, layout3, 0, 2); layout_vmargin(layout, 0, 3); layout_vmargin(layout, 1, 3); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_top_layout(DynData *data) { Layout *layout = layout_create(5, 3); i_fill_row(layout, 0, data); i_fill_row(layout, 1, data); i_fill_row(layout, 2, data); layout_hexpand(layout, 4); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_bottom_layout(DynData *data) { Layout *layout = layout_create(1, 3); i_fill_sublayout(layout, 0, data); i_fill_sublayout(layout, 1, data); i_fill_sublayout(layout, 2, data); return layout; } /*---------------------------------------------------------------------------*/ static DynData *i_panel_data(void) { DynData *data = heap_new0(DynData); data->hmargin = 5; data->vmargin = 0; /* Alternative colors for Light and Dark themes */ data->bgcolor[0] = gui_alt_color(color_rgb(255, 232, 232), color_rgb(128, 0, 0)); data->bgcolor[1] = gui_alt_color(color_rgb(232, 255, 232), color_rgb(0, 128, 0)); data->bgcolor[2] = gui_alt_color(color_rgb(232, 232, 255), color_rgb(0, 0, 128)); data->skcolor[0] = gui_alt_color(color_rgb(255, 85, 0), color_rgb(255,0, 0)); data->skcolor[1] = gui_alt_color(color_rgb(5, 163, 0), color_rgb(0, 255, 0)); data->skcolor[2] = gui_alt_color(color_rgb(109, 0, 163), color_rgb(0, 0, 255)); return data; } /*---------------------------------------------------------------------------*/ /* * Dynamic layouts example * * The main layout is a stack with three rows: * Control Layout: Buttons to add/remove dynamic layouts cells * Top Layout: Grid layout with simple widgets where we can add/remove columns/rows dynamically * Bottom Layout: A stack where we can add/remove complex sublayouts dynamically * * Main layout lives in a scroll panel with fixed size. * When main layout grows, scrollbars will be activated to browse all the content. * */ /*---------------------------------------------------------------------------*/ Panel *dynlay_panel(void) { DynData *data = i_panel_data(); Panel *panel = panel_scroll(TRUE, TRUE); Layout *layout = layout_create(1, 4); Layout *control_layout = i_control_layout(data); Layout *top_layout = i_top_layout(data); Layout *bottom_layout = i_bottom_layout(data); data->top_layout = top_layout; data->bottom_layout = bottom_layout; /* Main layout composition */ layout_layout(layout, control_layout, 0, 0); layout_layout(layout, top_layout, 0, 1); layout_layout(layout, bottom_layout, 0, 2); /* Grid layout dynamic margins */ i_top_layout_margins(data); /* Articles layout margins */ i_bottom_layout_margins(data); /* Static vertical separation between three layouts */ layout_vmargin(layout, 0, 10); layout_vmargin(layout, 1, 10); /* * The main layout vertical expansion is delegated to a fourth empty cell. * This prevents excess pixels from being distributed across all rows, * keeping the top three rows together. */ layout_vexpand(layout, 3); /* * Main container fixed size * Scrollbars will be activated when layouts grow */ panel_size(panel, s2df(400, 500)); /* Panel-Layout binding */ panel_layout(panel, layout); /* DynData is a dynamic structure that will be destroyed the Panel destroys */ panel_data(panel, &data, i_destroy_dyndata, DynData); return panel; } |
21. Hello dynamic Menu!
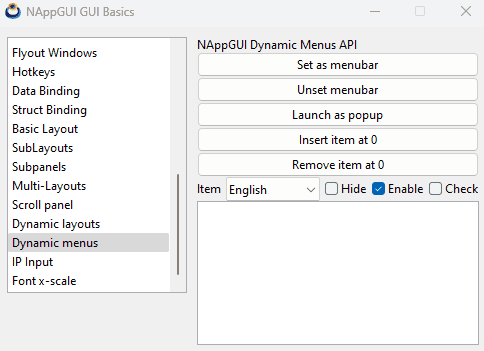
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 |
/* Dynamic menus */ #include "dynmenu.h" #include "res_guihello.h" #include <gui/guiall.h> #include <osapp/osapp.h> typedef struct _dynmenu_t DynMenu; struct _dynmenu_t { Menu *menu; Window *window; PopUp *popup; TextView *text; Button *hide_check; Button *enable_check; Button *state_check; uint32_t itemid; }; /*---------------------------------------------------------------------------*/ static void i_destroy_dynmenu(DynMenu **dmenu) { cassert_no_null(dmenu); menu_destroy(&(*dmenu)->menu); heap_delete(dmenu, DynMenu); } /*---------------------------------------------------------------------------*/ static void i_OnMenuClick(DynMenu *dmenu, Event *e) { MenuItem *item = event_sender(e, MenuItem); const char_t *text = menuitem_get_text(item); cassert_no_null(dmenu); textview_printf(dmenu->text, "Click: %s\n", text); } /*---------------------------------------------------------------------------*/ static void i_select_item(DynMenu *dmenu, const uint32_t index) { MenuItem *item = NULL; bool_t visible = TRUE; bool_t enabled = TRUE; cassert_no_null(dmenu); item = menu_get_item(dmenu->menu, index); visible = menuitem_get_visible(item); enabled = menuitem_get_enabled(item); button_state(dmenu->hide_check, visible ? ekGUI_OFF : ekGUI_ON); button_state(dmenu->enable_check, enabled ? ekGUI_ON : ekGUI_OFF); button_state(dmenu->state_check, menuitem_get_state(item)); } /*---------------------------------------------------------------------------*/ static void i_index_popup(DynMenu *dmenu) { uint32_t n; cassert_no_null(dmenu); n = menu_count(dmenu->menu); popup_clear(dmenu->popup); if (n > 0) { uint32_t i; for (i = 0; i < n; ++i) { const MenuItem *item = menu_get_citem(dmenu->menu, i); popup_add_elem(dmenu->popup, menuitem_get_text(item), NULL); } i_select_item(dmenu, popup_get_selected(dmenu->popup)); } } /*---------------------------------------------------------------------------*/ static MenuItem *i_menuitem(DynMenu *dmenu, const char_t *text, const Image *image) { MenuItem *item = menuitem_create(); menuitem_text(item, text); menuitem_image(item, image); menuitem_OnClick(item, listener(dmenu, i_OnMenuClick, DynMenu)); return item; } /*---------------------------------------------------------------------------*/ static Menu *i_menu(DynMenu *dmenu) { Menu *menu = menu_create(); Menu *submenu = menu_create(); MenuItem *item1 = i_menuitem(dmenu, "English", gui_image(UKING_PNG)); MenuItem *item2 = i_menuitem(dmenu, "Español", gui_image(SPAIN_PNG)); MenuItem *item3 = i_menuitem(dmenu, "Portugues", gui_image(PORTUGAL_PNG)); MenuItem *item4 = i_menuitem(dmenu, "Italiana", gui_image(ITALY_PNG)); MenuItem *item5 = i_menuitem(dmenu, "Tiếng Việt", gui_image(VIETNAM_PNG)); MenuItem *item6 = i_menuitem(dmenu, "России", gui_image(RUSSIA_PNG)); MenuItem *item7 = i_menuitem(dmenu, "日本語", gui_image(JAPAN_PNG)); MenuItem *item8 = i_menuitem(dmenu, "Red", gui_image(RED_PNG)); MenuItem *item9 = i_menuitem(dmenu, "Blue", gui_image(BLUE_PNG)); MenuItem *item10 = i_menuitem(dmenu, "Green", gui_image(GREEN_PNG)); MenuItem *item11 = i_menuitem(dmenu, "Yellow", gui_image(YELLOW_PNG)); MenuItem *item12 = i_menuitem(dmenu, "Black", gui_image(BLACK_PNG)); MenuItem *item13 = i_menuitem(dmenu, "White", gui_image(WHITE_PNG)); MenuItem *item14 = menuitem_create(); menu_add_item(menu, item1); menu_add_item(menu, item2); menu_add_item(menu, item3); menu_add_item(menu, item4); menu_add_item(menu, item5); menu_add_item(menu, item6); menu_add_item(menu, item7); menu_add_item(submenu, item8); menu_add_item(submenu, item9); menu_add_item(submenu, item10); menu_add_item(submenu, item11); menu_add_item(submenu, menuitem_separator()); menu_add_item(submenu, item12); menu_add_item(submenu, item13); menuitem_text(item14, "Colors"); menuitem_submenu(item14, &submenu); menu_add_item(menu, menuitem_separator()); menu_add_item(menu, item14); return menu; } /*---------------------------------------------------------------------------*/ static DynMenu *i_dynmenu(Window *window) { DynMenu *dmenu = heap_new0(DynMenu); dmenu->menu = i_menu(dmenu); dmenu->window = window; return dmenu; } /*---------------------------------------------------------------------------*/ static void i_OnSelectItem(DynMenu *dmenu, Event *e) { const EvButton *p = event_params(e, EvButton); i_select_item(dmenu, p->index); } /*---------------------------------------------------------------------------*/ static void i_OnHideClick(DynMenu *dmenu, Event *e) { cassert_no_null(dmenu); if (menu_count(dmenu->menu) > 0) { const EvButton *p = event_params(e, EvButton); uint32_t index = popup_get_selected(dmenu->popup); MenuItem *item = menu_get_item(dmenu->menu, index); bool_t visible = p->state == ekGUI_OFF; menuitem_visible(item, visible); window_update(dmenu->window); } } /*---------------------------------------------------------------------------*/ static void i_OnEnableClick(DynMenu *dmenu, Event *e) { cassert_no_null(dmenu); if (menu_count(dmenu->menu) > 0) { const EvButton *p = event_params(e, EvButton); uint32_t index = popup_get_selected(dmenu->popup); MenuItem *item = menu_get_item(dmenu->menu, index); bool_t enabled = p->state == ekGUI_ON; menuitem_enabled(item, enabled); } } /*---------------------------------------------------------------------------*/ static void i_OnStateClick(DynMenu *dmenu, Event *e) { cassert_no_null(dmenu); if (menu_count(dmenu->menu) > 0) { const EvButton *p = event_params(e, EvButton); uint32_t index = popup_get_selected(dmenu->popup); MenuItem *item = menu_get_item(dmenu->menu, index); menuitem_state(item, p->state); } } /*---------------------------------------------------------------------------*/ static void i_OnSetMenubar(DynMenu *dmenu, Event *e) { cassert_no_null(dmenu); unref(e); if (menu_is_menubar(dmenu->menu) == FALSE) { /* In apple menubar, the first menu item is attached automatically to app name menu entry. */ #if defined(__APPLE__) MenuItem *item00 = menuitem_create(); Menu *apple_app_menu = menu_create(); MenuItem *item01 = menuitem_create(); MenuItem *item02 = menuitem_create(); menuitem_text(item00, ""); menuitem_text(item01, "About..."); menuitem_text(item02, "Settings..."); menu_add_item(apple_app_menu, item01); menu_add_item(apple_app_menu, item02); menuitem_submenu(item00, &apple_app_menu); menu_ins_item(dmenu->menu, 0, item00); i_index_popup(dmenu); #endif osapp_menubar(dmenu->menu, dmenu->window); } } /*---------------------------------------------------------------------------*/ static void i_unset_menubar(DynMenu *dmenu) { cassert_no_null(dmenu); if (menu_is_menubar(dmenu->menu) == TRUE) { /* Remove the Apple 'appName' first menu entry */ #if defined(__APPLE__) menu_del_item(dmenu->menu, 0); i_index_popup(dmenu); #endif osapp_menubar(NULL, dmenu->window); } } /*---------------------------------------------------------------------------*/ static void i_OnUnsetMenubar(DynMenu *dmenu, Event *e) { unref(e); i_unset_menubar(dmenu); } /*---------------------------------------------------------------------------*/ static void i_OnPopUpMenu(DynMenu *dmenu, Event *e) { V2Df pos; cassert_no_null(dmenu); unref(e); /* First, unlink the menu if has a menubar role */ i_unset_menubar(dmenu); /* Screen position of top-left textview corner */ { R2Df frame = window_control_frame(dmenu->window, guicontrol(dmenu->text)); pos = window_client_to_screen(dmenu->window, frame.pos); } /* Launch as popup */ menu_launch(dmenu->menu, dmenu->window, pos); } /*---------------------------------------------------------------------------*/ static void i_OnInsertItem(DynMenu *dmenu, Event *e) { MenuItem *item = menuitem_create(); char_t text[64]; uint32_t pos = 0; cassert_no_null(dmenu); unref(e); bstd_sprintf(text, sizeof(text), "NewItem%d", dmenu->itemid++); menuitem_text(item, text); menuitem_OnClick(item, listener(dmenu, i_OnMenuClick, DynMenu)); #if defined(__APPLE__) /* In macOS, we insert the new item AFTER the 'appName' menu item */ if (menu_is_menubar(dmenu->menu) == TRUE) pos = 1; #endif menu_ins_item(dmenu->menu, pos, item); i_index_popup(dmenu); window_update(dmenu->window); } /*---------------------------------------------------------------------------*/ static void i_OnRemoveItem(DynMenu *dmenu, Event *e) { uint32_t pos = 0; cassert_no_null(dmenu); unref(e); #if defined(__APPLE__) /* In macOS, we remove the item AFTER the 'appName' menu item */ if (menu_is_menubar(dmenu->menu) == TRUE) pos = 1; #endif if (menu_count(dmenu->menu) > pos) { menu_del_item(dmenu->menu, pos); i_index_popup(dmenu); window_update(dmenu->window); } } /*---------------------------------------------------------------------------*/ Panel *dynmenu_panel(Window *window) { DynMenu *dmenu = i_dynmenu(window); Layout *layout1 = layout_create(1, 8); Layout *layout2 = layout_create(5, 1); Label *label1 = label_create(); Label *label2 = label_create(); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); Button *button5 = button_push(); Button *button6 = button_check(); Button *button7 = button_check(); Button *button8 = button_check(); PopUp *popup = popup_create(); TextView *text = textview_create(); Panel *panel = panel_create(); dmenu->text = text; label_text(label1, "NAppGUI Dynamic Menus API"); label_text(label2, "Item"); button_text(button1, "Set as menubar"); button_text(button2, "Unset menubar"); button_text(button3, "Launch as popup"); button_text(button4, "Insert item at 0"); button_text(button5, "Remove item at 0"); button_text(button6, "Hide"); button_text(button7, "Enable"); button_text(button8, "Check"); button_OnClick(button1, listener(dmenu, i_OnSetMenubar, DynMenu)); button_OnClick(button2, listener(dmenu, i_OnUnsetMenubar, DynMenu)); button_OnClick(button3, listener(dmenu, i_OnPopUpMenu, DynMenu)); button_OnClick(button4, listener(dmenu, i_OnInsertItem, DynMenu)); button_OnClick(button5, listener(dmenu, i_OnRemoveItem, DynMenu)); button_OnClick(button6, listener(dmenu, i_OnHideClick, DynMenu)); button_OnClick(button7, listener(dmenu, i_OnEnableClick, DynMenu)); button_OnClick(button8, listener(dmenu, i_OnStateClick, DynMenu)); popup_OnSelect(popup, listener(dmenu, i_OnSelectItem, DynMenu)); layout_label(layout1, label1, 0, 0); layout_button(layout1, button1, 0, 1); layout_button(layout1, button2, 0, 2); layout_button(layout1, button3, 0, 3); layout_button(layout1, button4, 0, 4); layout_button(layout1, button5, 0, 5); layout_layout(layout1, layout2, 0, 6); layout_textview(layout1, text, 0, 7); layout_label(layout2, label2, 0, 0); layout_popup(layout2, popup, 1, 0); layout_button(layout2, button6, 2, 0); layout_button(layout2, button7, 3, 0); layout_button(layout2, button8, 4, 0); layout_halign(layout1, 0, 6, ekLEFT); layout_hmargin(layout2, 0, 5); layout_hmargin(layout2, 1, 5); layout_hmargin(layout2, 2, 5); layout_hmargin(layout2, 3, 5); panel_layout(panel, layout1); dmenu->popup = popup; dmenu->hide_check = button6; dmenu->enable_check = button7; dmenu->state_check = button8; i_index_popup(dmenu); panel_data(panel, &dmenu, i_destroy_dynmenu, DynMenu); return panel; } |
22. Hello IP-Input!
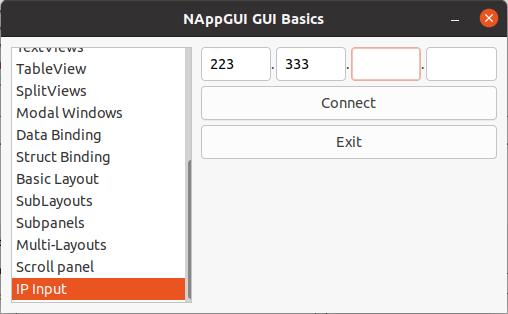
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
/* IP input */ #include "ipinput.h" #include <gui/guiall.h> /*---------------------------------------------------------------------------*/ static void i_OnEditFilter(Window *window, Event* e) { const EvText *p = event_params(e, EvText); EvTextFilter *filter = event_result(e, EvTextFilter); uint32_t i, j = 0, n = str_len_c(p->text); /* We only accept numbers in IP controls */ for(i = 0; i < n; ++i) { if (p->text[i] >= '0' && p->text[i] <= '9') filter->text[j++] = p->text[i]; } if (j > 3) j = 3; filter->text[j] = '\0'; filter->apply = TRUE; /* We wrote the third character --> Jump to next control */ if (j == 3) window_next_tabstop(window); } /*---------------------------------------------------------------------------*/ Panel *ip_input(Window *window) { Panel *panel = panel_create(); Layout *layout1 = layout_create(7, 1); Layout *layout2 = layout_create(1, 3); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); Edit *edit3 = edit_create(); Edit *edit4 = edit_create(); Button *button1 = button_push(); Button *button2 = button_push(); label_text(label1, "."); label_text(label2, "."); label_text(label3, "."); button_text(button1, "Connect"); button_text(button2, "Exit"); edit_OnFilter(edit1, listener(window, i_OnEditFilter, Window)); edit_OnFilter(edit2, listener(window, i_OnEditFilter, Window)); edit_OnFilter(edit3, listener(window, i_OnEditFilter, Window)); edit_OnFilter(edit4, listener(window, i_OnEditFilter, Window)); layout_label(layout1, label1, 1, 0); layout_label(layout1, label2, 3, 0); layout_label(layout1, label3, 5, 0); layout_edit(layout1, edit1, 0, 0); layout_edit(layout1, edit2, 2, 0); layout_edit(layout1, edit3, 4, 0); layout_edit(layout1, edit4, 6, 0); layout_layout(layout2, layout1, 0, 0); layout_button(layout2, button1, 0, 1); layout_button(layout2, button2, 0, 2); layout_vmargin(layout2, 0, 5.f); layout_vmargin(layout2, 1, 5.f); layout_hsize(layout2, 0, 200.f); panel_layout(panel, layout2); return panel; } |
23. Hello Font Stretch!
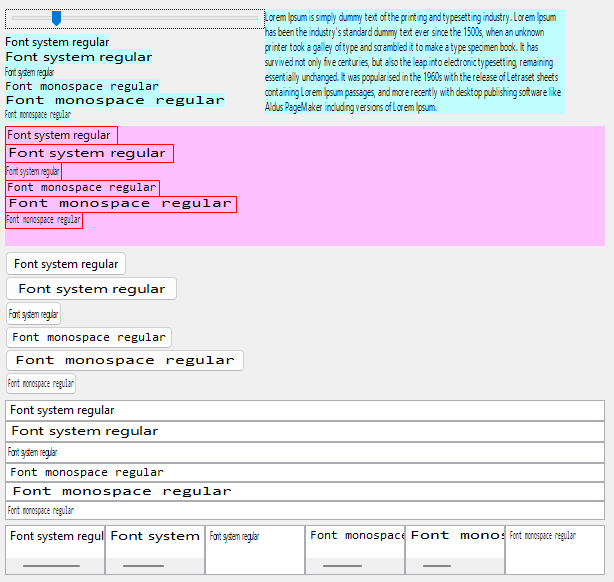
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 |
/* Font x-scale */ #include "fontx.h" #include <gui/guiall.h> typedef struct _fontx_t FontX; struct _fontx_t { Font *font_1_0; Font *font_1_5; Font *font_0_5; Font *fontm_1_0; Font *fontm_1_5; Font *fontm_0_5; Label *mline; Layout *layout; color_t c1; color_t c2; }; const char_t *i_TEXT1 = "Font system regular"; const char_t *i_TEXT2 = "Font monospace regular"; const char_t *i_TEXT3 = "Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum."; /*---------------------------------------------------------------------------*/ static void i_destroy_fontx(FontX **fontx) { cassert_no_null(fontx); cassert_no_null(*fontx); font_destroy(&(*fontx)->font_1_0); font_destroy(&(*fontx)->font_1_5); font_destroy(&(*fontx)->font_0_5); font_destroy(&(*fontx)->fontm_1_0); font_destroy(&(*fontx)->fontm_1_5); font_destroy(&(*fontx)->fontm_0_5); heap_delete(fontx, FontX); } /*---------------------------------------------------------------------------*/ static void i_OnMoved(FontX *fontx, Event *e) { const EvSlider *p = event_params(e, EvSlider); Font *font = font_system(font_regular_size(), 0); Font *font_sx = font_with_xscale(font, p->pos + .5f); label_font(fontx->mline, font_sx); layout_update(fontx->layout); font_destroy(&font_sx); font_destroy(&font); } /*---------------------------------------------------------------------------*/ static Layout *i_label_layout(FontX *fontx) { Layout *layout1 = layout_create(2, 1); Layout *layout2 = layout_create(1, 7); Slider *slider = slider_create(); Label *label1 = label_create(); Label *label2 = label_create(); Label *label3 = label_create(); Label *label4 = label_create(); Label *label5 = label_create(); Label *label6 = label_create(); Label *label7 = label_create(); cassert_no_null(fontx); slider_value(slider, .5f); slider_OnMoved(slider, listener(fontx, i_OnMoved, FontX)); label_multiline(label7, TRUE); label_text(label1, i_TEXT1); label_text(label2, i_TEXT1); label_text(label3, i_TEXT1); label_text(label4, i_TEXT2); label_text(label5, i_TEXT2); label_text(label6, i_TEXT2); label_text(label7, i_TEXT3); label_font(label1, fontx->font_1_0); label_font(label2, fontx->font_1_5); label_font(label3, fontx->font_0_5); label_font(label4, fontx->fontm_1_0); label_font(label5, fontx->fontm_1_5); label_font(label6, fontx->fontm_0_5); label_font(label7, fontx->font_1_0); label_bgcolor(label1, fontx->c1); label_bgcolor(label2, fontx->c1); label_bgcolor(label3, fontx->c1); label_bgcolor(label4, fontx->c1); label_bgcolor(label5, fontx->c1); label_bgcolor(label6, fontx->c1); label_bgcolor(label7, fontx->c1); layout_slider(layout2, slider, 0, 0); layout_label(layout2, label1, 0, 1); layout_label(layout2, label2, 0, 2); layout_label(layout2, label3, 0, 3); layout_label(layout2, label4, 0, 4); layout_label(layout2, label5, 0, 5); layout_label(layout2, label6, 0, 6); layout_vmargin(layout2, 0, 5); layout_valign(layout1, 0, 0, ekTOP); layout_valign(layout1, 1, 0, ekTOP); layout_hsize(layout1, 1, 300); layout_layout(layout1, layout2, 0, 0); layout_label(layout1, label7, 1, 0); fontx->mline = label7; fontx->layout = layout1; return layout1; } /*---------------------------------------------------------------------------*/ static Layout *i_button_layout(FontX *fontx) { Layout *layout = layout_create(1, 6); Button *button1 = button_push(); Button *button2 = button_push(); Button *button3 = button_push(); Button *button4 = button_push(); Button *button5 = button_push(); Button *button6 = button_push(); cassert_no_null(fontx); button_text(button1, i_TEXT1); button_text(button2, i_TEXT1); button_text(button3, i_TEXT1); button_text(button4, i_TEXT2); button_text(button5, i_TEXT2); button_text(button6, i_TEXT2); button_font(button1, fontx->font_1_0); button_font(button2, fontx->font_1_5); button_font(button3, fontx->font_0_5); button_font(button4, fontx->fontm_1_0); button_font(button5, fontx->fontm_1_5); button_font(button6, fontx->fontm_0_5); layout_button(layout, button1, 0, 0); layout_button(layout, button2, 0, 1); layout_button(layout, button3, 0, 2); layout_button(layout, button4, 0, 3); layout_button(layout, button5, 0, 4); layout_button(layout, button6, 0, 5); layout_halign(layout, 0, 0, ekLEFT); layout_halign(layout, 0, 1, ekLEFT); layout_halign(layout, 0, 2, ekLEFT); layout_halign(layout, 0, 3, ekLEFT); layout_halign(layout, 0, 4, ekLEFT); layout_halign(layout, 0, 5, ekLEFT); return layout; } /*---------------------------------------------------------------------------*/ static Layout *i_edit_layout(FontX *fontx) { Layout *layout = layout_create(1, 6); Edit *edit1 = edit_create(); Edit *edit2 = edit_create(); Edit *edit3 = edit_create(); Edit *edit4 = edit_create(); Edit *edit5 = edit_create(); Edit *edit6 = edit_create(); cassert_no_null(fontx); edit_text(edit1, i_TEXT1); edit_text(edit2, i_TEXT1); edit_text(edit3, i_TEXT1); edit_text(edit4, i_TEXT2); edit_text(edit5, i_TEXT2); edit_text(edit6, i_TEXT2); edit_font(edit1, fontx->font_1_0); edit_font(edit2, fontx->font_1_5); edit_font(edit3, fontx->font_0_5); edit_font(edit4, fontx->fontm_1_0); edit_font(edit5, fontx->fontm_1_5); edit_font(edit6, fontx->fontm_0_5); layout_edit(layout, edit1, 0, 0); layout_edit(layout, edit2, 0, 1); layout_edit(layout, edit3, 0, 2); layout_edit(layout, edit4, 0, 3); layout_edit(layout, edit5, 0, 4); layout_edit(layout, edit6, 0, 5); return layout; } /*---------------------------------------------------------------------------*/ static ListBox *i_listbox(const Font *font, const char_t *text) { ListBox *listbox = listbox_create(); listbox_font(listbox, font); listbox_add_elem(listbox, text, NULL); listbox_size(listbox, s2df(100, 50)); return listbox; } /*---------------------------------------------------------------------------*/ static Layout *i_list_layout(FontX *fontx) { Layout *layout = layout_create(6, 1); ListBox *list1 = i_listbox(fontx->font_1_0, i_TEXT1); ListBox *list2 = i_listbox(fontx->font_1_5, i_TEXT1); ListBox *list3 = i_listbox(fontx->font_0_5, i_TEXT1); ListBox *list4 = i_listbox(fontx->fontm_1_0, i_TEXT2); ListBox *list5 = i_listbox(fontx->fontm_1_5, i_TEXT2); ListBox *list6 = i_listbox(fontx->fontm_0_5, i_TEXT2); layout_listbox(layout, list1, 0, 0); layout_listbox(layout, list2, 1, 0); layout_listbox(layout, list3, 2, 0); layout_listbox(layout, list4, 3, 0); layout_listbox(layout, list5, 4, 0); layout_listbox(layout, list6, 5, 0); return layout; } /*---------------------------------------------------------------------------*/ static void i_OnDraw(FontX *fontx, Event *e) { const EvDraw *p = event_params(e, EvDraw); real32_t w1, w2, w3, w4, w5, w6; real32_t h1, h2, h3, h4, h5, h6; cassert_no_null(fontx); draw_clear(p->ctx, fontx->c2); draw_text_color(p->ctx, gui_label_color()); draw_font(p->ctx, fontx->font_1_0); draw_text_extents(p->ctx, i_TEXT1, -1, &w1, &h1); draw_text(p->ctx, i_TEXT1, 0, 0); draw_font(p->ctx, fontx->font_1_5); draw_text_extents(p->ctx, i_TEXT1, -1, &w2, &h2); draw_text(p->ctx, i_TEXT1, 0, h1); draw_font(p->ctx, fontx->font_0_5); draw_text_extents(p->ctx, i_TEXT1, -1, &w3, &h3); draw_text(p->ctx, i_TEXT1, 0, h1 + h2); draw_font(p->ctx, fontx->fontm_1_0); draw_text_extents(p->ctx, i_TEXT2, -1, &w4, &h4); draw_text(p->ctx, i_TEXT2, 0, h1 + h2 + h3); draw_font(p->ctx, fontx->fontm_1_5); draw_text_extents(p->ctx, i_TEXT2, -1, &w5, &h5); draw_text(p->ctx, i_TEXT2, 0, h1 + h2 + h3 + h4); draw_font(p->ctx, fontx->fontm_0_5); draw_text_extents(p->ctx, i_TEXT2, -1, &w6, &h6); draw_text(p->ctx, i_TEXT2, 0, h1 + h2 + h3 + h4 + h5); draw_line_color(p->ctx, kCOLOR_RED); draw_rect(p->ctx, ekSTROKE, 0, 0, w1, h1); draw_rect(p->ctx, ekSTROKE, 0, h1, w2, h2); draw_rect(p->ctx, ekSTROKE, 0, h1 + h2, w3, h3); draw_rect(p->ctx, ekSTROKE, 0, h1 + h2 + h3, w4, h4); draw_rect(p->ctx, ekSTROKE, 0, h1 + h2 + h3 + h4, w5, h5); draw_rect(p->ctx, ekSTROKE, 0, h1 + h2 + h3 + h4 + h5, w6, h6); } /*---------------------------------------------------------------------------*/ static Layout *i_layout(FontX *fontx) { Layout *layout1 = layout_create(1, 5); Layout *layout2 = i_label_layout(fontx); Layout *layout3 = i_button_layout(fontx); Layout *layout4 = i_edit_layout(fontx); Layout *layout5 = i_list_layout(fontx); View *view = view_create(); view_OnDraw(view, listener(fontx, i_OnDraw, FontX)); view_size(view, s2df(200, 120)); layout_layout(layout1, layout2, 0, 0); layout_view(layout1, view, 0, 1); layout_layout(layout1, layout3, 0, 2); layout_layout(layout1, layout4, 0, 3); layout_layout(layout1, layout5, 0, 4); layout_vmargin(layout1, 0, 5); layout_vmargin(layout1, 1, 5); layout_vmargin(layout1, 2, 5); layout_vmargin(layout1, 3, 5); return layout1; } /*---------------------------------------------------------------------------*/ static FontX *i_fontx(void) { FontX *fontx = heap_new(FontX); fontx->font_1_0 = font_system(font_regular_size(), 0); fontx->font_1_5 = font_with_xscale(fontx->font_1_0, 1.5f); fontx->font_0_5 = font_with_xscale(fontx->font_1_0, 0.5f); fontx->fontm_1_0 = font_monospace(font_regular_size(), 0); fontx->fontm_1_5 = font_with_xscale(fontx->fontm_1_0, 1.5f); fontx->fontm_0_5 = font_with_xscale(fontx->fontm_1_0, 0.5f); fontx->c1 = gui_alt_color(color_rgb(192, 255, 255), color_rgb(48, 112, 112)); fontx->c2 = gui_alt_color(color_rgb(255, 192, 255), color_rgb(128, 48, 112)); return fontx; } /*---------------------------------------------------------------------------*/ Panel *font_x_scale(void) { FontX *fontx = i_fontx(); Layout *layout = i_layout(fontx); Panel *panel = panel_create(); panel_data(panel, &fontx, i_destroy_fontx, FontX); panel_layout(panel, layout); return panel; } |