Sewer
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Even the grandest palaces needed sewers. Tom Lehrer
Header
#include <sewer/sewer.h>
Functions
void | (*FPtr_destroy (...)) |
type* | (*FPtr_copy (...)) |
void | (*FPtr_scopy (...)) |
int | (*FPtr_compare (...)) |
int | (*FPtr_compare_ex (...)) |
void | (*FPtr_assert (...)) |
void | unref (...) |
Types and Constants
Sewer is the first library within the NAppGUI SDK (Figure 1). It declares the basic types, the Unicode support, assertions, pointers safe manipulation, elementary math functions, Standard I/O and dynamic memory allocation. It is also used as a "sink" to bury the unsightly preprocessor macros necessary to configure the compiler, CPU, platforms, etc. As dependencies only has a few headers of the C standard library:
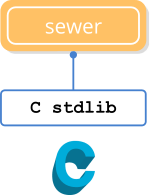
1. The C standard library
The C standard library (cstdlib) is not part of the C language, but implements functions of great utility for the developer that solve typical programming problems. Any C programmer has used it more or less and its study is usually linked to learning the language itself (Figure 2).
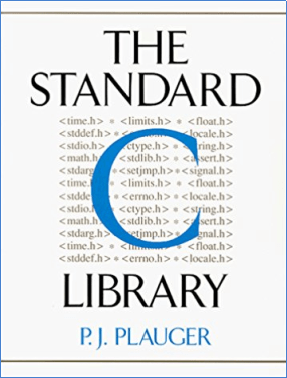
This library is located halfway between the application and system calls and provides a portable API for file access, dynamic memory, I/O, time, etc (Figure 3). It also implements mathematical functions, conversion, search, string management, etc. In one way or another, NAppGUI integrates its functionality, so it's not necessary (or advisable) to use cstdlib directly in the application layer. The reasons that have motivated this design decision can be summarized in:
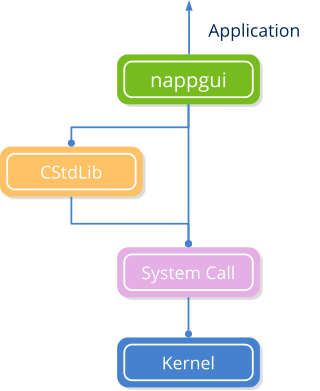
- Small differences: Unix-like systems do not support the secure cstdlib versions implemented by Microsoft (
strcpy_s()
and others). The use of classical functions (without the suffix_s
) is insecure and will trigger annoying warnings in Visual Studio. - Security: Related to the previous one, avoids buffer overflow vulnerabilities in the processing of memory blocks and strings.
- Duplicity: Much of the functionality of cstdlib is already implemented in osbs library using direct system calls (files, dynamic memory, I/O, time, etc.)
- Completeness: The cstdlib functions related to files (
fopen()
and others) do not include support for directory management. Files and directories presents a complete API based on system calls. - Performance: In certain cases, especially in mathematical functions and memory management, it may be interesting to change the implementation of cstdlib to an improved one. All applications will benefit from the change, without having to modify your code.
- Clarity: The behavior of some cstdlib functions is not entirely clear and can lead to confusion. For example,
strtoul
has a very particular functioning that we must remember every time we use it. - Style: The use of sewer functions does not break the aesthetics of an application written with NAppGUI.
- Independence: NAppGUI internally uses a very small subset of cstdlib functions. It is possible that in the future we will make our own implementations and completely disconnect the support of the standard library.
- Static link: If we statically link the standard library, sewer will contain all dependencies internally. This will avoid possible incompatibilities with the runtimes installed on each machine (the classic Windows VC++ Redistributables). With this we will be certain that our executables will work, regardless of the version of the C runtime that exists in each case. If all calls to cstdlib are inside sewer, we free higher-level libraries from their handling and possible runtime errors related to the C runtime.
1 2 3 4 5 6 7 |
1 2 3 4 5 6 7 |
real32_t a1 = 1.43f; real64_t a2 = .38; real32_t c = (real32_t)cosf((float)a1); real64_t t = (real64_t)tan((double)a2); ... real32_t c = bmath_cosf(a1); real64_t t = bmath_tand(a2); |
|
RUNTIME_C_LIBRARY "static" dumpbin /dependents dsewer.dll Image has the following dependencies: KERNEL32.dll |
|
RUNTIME_C_LIBRARY "dynamic" dumpbin /dependents dsewer.dll Image has the following dependencies: KERNEL32.dll VCRUNTIME140D.dll ucrtbased.dll |
To avoid possible bugs or incompatibilities, do not use C Standard Library functions directly in applications. Always look for an equivalent NAppGUI function.
int8_t
8-bit signed integer. It can represent a value between INT8_MIN and INT8_MAX.
int16_t
16-bit signed integer. It can represent a value between INT16_MIN and INT16_MAX.
int32_t
32-bit signed integer. It can represent a value between INT32_MIN and INT32_MAX.
int64_t
64-bit signed integer. It can represent a value between INT64_MIN and INT64_MAX.
uint8_t
8-bit unsigned integer. It can represent a value between 0 and UINT8_MAX.
uint16_t
16-bit unsigned integer. It can represent a value between 0 and UINT16_MAX.
uint32_t
32-bit unsigned integer. It can represent a value between 0 and UINT32_MAX.
uint64_t
64-bit unsigned integer. It can represent a value between 0 and UINT64_MAX.
char_t
8-bit character type (Unicode). A single character may need 1, 2, 3 or 4 elements (bytes), depending on UTF encodings.
byte_t
8-bit type to store generic memory blocks.
bool_t
8-bit boolean. Only two values are allowed TRUE (1) and FALSE (0).
real
32 or 64-bit floating point number.
real32_t
32-bit floating point number. The C float
type.
real64_t
64-bit floating point number. The C double
type.
TRUE
const bool_t TRUE = 1;
True.
FALSE
const bool_t FALSE = 0;
False.
NULL
const void* NULL = 0;
Null pointer.
INT8_MIN
const int8_t INT8_MIN = 0x80;
-128.
INT8_MAX
const int8_t INT8_MAX = 0x7F;
127.
INT16_MIN
const int16_t INT16_MIN = 0x8000;
-32.768.
INT16_MAX
const int16_t INT16_MAX = 0x7FFF;
32.767.
INT32_MIN
const int32_t INT32_MIN = 0x80000000;
-2.147.483.648.
INT32_MAX
const int32_t INT32_MAX = 0x7FFFFFFF;
2.147.483.647.
INT64_MIN
const int64_t INT64_MIN = 0x8000000000000000;
-9.223.372.036.854.775.808.
INT64_MAX
const int64_t INT64_MAX = 0x7FFFFFFFFFFFFFFF;
9.223.372.036.854.775.807.
UINT8_MAX
const uint8_t UINT8_MAX = 0xFF;
255.
UINT16_MAX
const uint16_t UINT16_MAX = 0xFFFF;
65.535.
UINT32_MAX
const uint32_t UINT32_MAX = 0xFFFFFFFF;
4.294.967.295.
UINT64_MAX
const uint64_t UINT64_MAX = 0xFFFFFFFFFFFFFFFF;
18.446.744.073.709.551.615.
enum unicode_t
Represents the UTF encodings.
enum unicode_t
{
ekUTF8,
ekUTF16,
ekUTF32
};
ekUTF8 | UTF8 encoding. |
ekUTF16 | UTF16 encoding. |
ekUTF32 | UTF32 encoding. |
struct REnv
Random numbers environment.
struct REnv;
FPtr_destroy
Destructor function prototype.
void (*FPtr_destroy)(type **item);
item | Double pointer to the object to destroy. It must be assigned to |
FPtr_copy
Copy constructor function prototype.
type* (*FPtr_copy)(const type *item);
item | Pointer to the object to be copied. |
Return
The new object that is an exact copy of the input.
FPtr_scopy
Unallocated memory copy constructor prototype.
void (*FPtr_scopy)(type *dest, const type *src);
dest | Destination object (copy). |
src | Pointer to the object to be copied (source). |
Remarks
In this copy operation, the memory required by the object has already been allocated. We must create dynamic memory for the fields of the object that require it, but not for the object itself. Usually used to copy arrays of objects (not pointers to objects).
FPtr_compare
Comparison function prototype.
int (*FPtr_compare)(const type *item1, const type *item2);
item1 | First item to compare. |
item2 | Second item to compare. |
Return
Comparison result.
FPtr_compare_ex
Similar to FPtr_compare
, but receive an additional parameter that may influence the comparison.
int (*FPtr_compare_ex)(const type *item1, const type *item2, const dtype *data);
item1 | First item to compare. |
item2 | Second item to compare. |
data | Additional parameter. |
Return
Comparison result.
FPtr_assert
Callback function prototype called when an assert
occurs.
void (*FPtr_assert)(type *item, const uint32_t group, const char_t *caption, const char_t *detail, const char_t *file, const uint32_t line);
item | User data passed as the first parameter. |
group | 0 = Fatal error, 1 = Execution can continue. |
caption | Title. |
detail | Detailed message. |
file | Source file where the assert occurred. |
line | Line inside the source file. |
unref ()
Mark the parameter as non-referenced, disabling the compiler's warnings.
void
unref(param);
param | Parameter. |