2D Oriented Boxes
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
OBB2D* | obb2d_create (...) |
OBB2D* | obb2d_from_line (...) |
OBB2D* | obb2d_from_points (...) |
OBB2D* | obb2d_copy (...) |
void | obb2d_destroy (...) |
void | obb2d_update (...) |
void | obb2d_move (...) |
void | obb2d_transform (...) |
const V2D* | obb2d_corners (...) |
V2D | obb2d_center (...) |
real | obb2d_width (...) |
real | obb2d_height (...) |
real | obb2d_angle (...) |
real | obb2d_area (...) |
Box2D | obb2d_box (...) |
Oriented Bounding Boxes are 2D boxes that can rotate about their center (Figure 1), so they will no longer be aligned with axes. Here the collision detection is somewhat complicated compared to 2D Axis-Aligned boxes, in exchange for providing a better fit against elongated objects that can rotate in the plane.
- Use obb2d_from_pointsf to create an oriented box from a set of points.
- Use obb2d_from_linef to create an oriented box from a segment.
- Use obb2d_transformf to apply a 2D transformation to the box.
- Use obb2d_boxf to get the aligned box containing the oriented box.
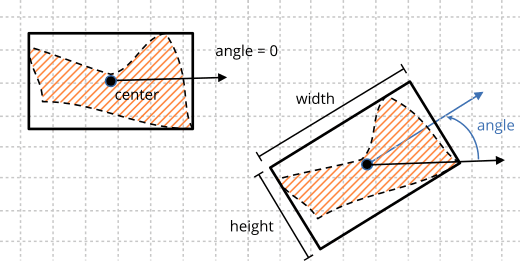
We can obtain relevant parameters of an arbitrary set of points from the covariance matrix (Formula 1), which is geometrically represented by an ellipse rotated in the plane and centered on the mean of the distribution (Figure 2). This analysis allows obb2d_from_pointsf to calculate the 2D box associated with the distribution in a quite acceptable way, without becoming the optimal solution that is much more expensive in computational terms.
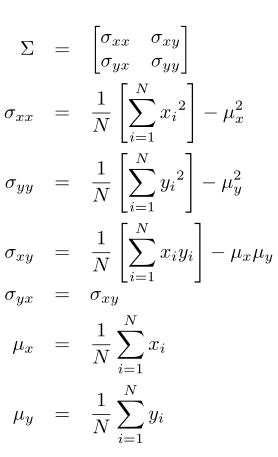
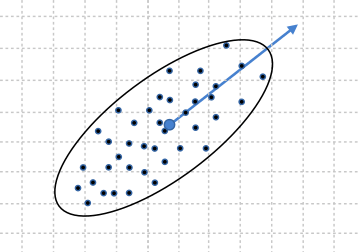
Use oriented boxes (OBB2Df
) for "elongated" point distributions. In rounded or square cases the aligned box (Box2Df
) can provide a volume with a smaller area.
obb2d_create ()
Create a new oriented box.
OBB2Df* obb2d_createf(const V2Df *center, const real32_t width, const real32_t height, const real32_t angle); OBB2Dd* obb2d_created(const V2Dd *center, const real64_t width, const real64_t height, const real64_t angle); OBB2D* OBB2D::create(const V2D *center, const real width, const real height, const real angle);
center | The central point. |
width | The width of the box. |
height | The height of the box. |
angle | The angle with respect to the X axis, in radians. |
Return
The newly created box.
Remarks
Positive angles are those that rotate from the X axis to the Y axis.
obb2d_from_line ()
Create a box from a segment.
OBB2Df* obb2d_from_linef(const V2Df *p0, const V2Df *p1, const real32_t thickness); OBB2Dd* obb2d_from_lined(const V2Dd *p0, const V2Dd *p1, const real64_t thickness); OBB2D* OBB2D::from_line(const V2D *p0, const V2D *p1, const real thickness);
p0 | The first point of the segment. |
p1 | The second point of the segment. |
thickness | The "thickness" of the segment. |
Return
The newly created box.
Remarks
The width of the box will correspond to the length of the segment. The height will be thickness
and the center will be the midpoint of the segment.
obb2d_from_points ()
Create an oriented box from a set of points.
OBB2Df* obb2d_from_pointsf(const V2Df *p, const uint32_t n); OBB2Dd* obb2d_from_pointsd(const V2Dd *p, const uint32_t n); OBB2D* OBB2D::from_points(const V2D *p, const uint32_t n);
p | Points array. |
n | Number of points. |
Return
The newly created box.
Remarks
A good fit will be produced in "elongated" point distributions by calculating the covariance matrix and projecting points onto the director vector of that distribution. However, it does not provide the minimum volume box.
obb2d_copy ()
Create a copy of the box.
OBB2Df* obb2d_copyf(const OBB2Df obb); OBB2Dd* obb2d_copyd(const OBB2Dd obb); OBB2D* OBB2D::copy(const OBB2D obb);
obb | Original box. |
Return
The copy.
obb2d_destroy ()
Destroy the box.
void obb2d_destroyf(OBB2Df **obb); void obb2d_destroyd(OBB2Dd **obb); void OBB2D::destroy(OBB2D **obb);
obb | The box. Will be set to |
obb2d_update ()
Update the box parameters.
void obb2d_updatef(OBB2Df *obb, const V2Df *center, const real32_t width, const real32_t height, const real32_t angle); void obb2d_updated(OBB2Dd *obb, const V2Dd *center, const real64_t width, const real64_t height, const real64_t angle); void OBB2D::update(OBB2D *obb, const V2D *center, const real width, const real height, const real angle);
obb | The box to update. |
center | The central point. |
width | The width. |
height | The height. |
angle | The angle. |
Remarks
See obb2d_createf.
obb2d_move ()
Move the box on the plane.
void obb2d_movef(OBB2Df *obb, const real32_t offset_x, const real32_t offset_y); void obb2d_moved(OBB2Dd *obb, const real64_t offset_x, const real64_t offset_y); void OBB2D::move(OBB2D *obb, const real offset_x, const real offset_y);
obb | The box. |
offset_x | X displacement. |
offset_y | Y displacement. |
obb2d_transform ()
Apply a transformation to the box.
void obb2d_transformf(OBB2Df *obb, const T2Df *t2d); void obb2d_transformd(OBB2Dd *obb, const T2Dd *t2d); void OBB2D::transform(OBB2D *obb, const T2D *t2d);
obb | The box. |
t2d | Affine transformation. |
obb2d_corners ()
Gets the vertices bounding the box.
const V2Df* obb2d_cornersf(const OBB2Df *obb); const V2Dd* obb2d_cornersd(const OBB2Dd *obb); const V2D* OBB2D::corners(const OBB2D *obb);
obb | The box. |
Return
Pointer to an array of 4 vertices.
Remarks
Do not modify the returned array. Copy if necessary.
obb2d_center ()
Gets the center point of the box.
V2Df obb2d_centerf(const OBB2Df *obb); V2Dd obb2d_centerd(const OBB2Dd *obb); V2D OBB2D::center(const OBB2D *obb);
obb | The box. |
Return
Center.
obb2d_width ()
Get the width of the box.
real32_t obb2d_widthf(const OBB2Df *obb); real64_t obb2d_widthd(const OBB2Dd *obb); real OBB2D::width(const OBB2D *obb);
obb | The box. |
Return
The width.
obb2d_height ()
Get the height of the box.
real32_t obb2d_heightf(const OBB2Df *obb); real64_t obb2d_heightd(const OBB2Dd *obb); real OBB2D::height(const OBB2D *obb);
obb | The box. |
Return
The height.
obb2d_angle ()
Get the angle of the box.
real32_t obb2d_anglef(const OBB2Df *obb); real64_t obb2d_angled(const OBB2Dd *obb); real OBB2D::angle(const OBB2D *obb);
obb | The box. |
Return
The angle in radians with respect to the X axis.
obb2d_area ()
Gets the box area.
real32_t obb2d_areaf(const OBB2Df *obb); real64_t obb2d_aread(const OBB2Dd *obb); real OBB2D::area(const OBB2D *obb);
obb | The box. |
Return
The area (width * height
).
obb2d_box ()
Get the box limits.
Box2Df obb2d_boxf(const OBB2Df *obb); Box2Dd obb2d_boxd(const OBB2Dd *obb); Box2D OBB2D::box(const OBB2D *obb);
obb | The box. |
Return
Box aligned with the axes, defined by the minimum and maximum vectors.