OGL3D
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Header
#include <ogl3d/ogl3d.h>
Functions
void | ogl3d_start (void) |
void | ogl3d_finish (void) |
OGLCtx* | ogl3d_context (...) |
void | ogl3d_destroy (...) |
void | ogl3d_begin_draw (...) |
void | ogl3d_end_draw (...) |
void | ogl3d_set_size (...) |
const char_t* | ogl3d_err_str (...) |
Types and Constants
enum | oglapi_t |
enum | oglerr_t |
struct | OGLProps |
struct | OGLCtx |
The OGL3D library will allow us to create cross-platform OpenGL contexts, without having to worry about the particular implementation in each operating system (Figure 1). Although the OpenGL API is fully portable, the way you create graphical contexts and link them to a window is platform-dependent. In Hello 3D Graphics! you have an example application.
Important. To use OGL3D in your projects, you will have to link the library explicitly in the CMakeLists.txt
of your application.
1 2 |
nap_desktop_app(GLHello "" NRC_EMBEDDED) nap_link_opengl(GLHello) |
Important. On Linux you will need to install the Mesa development libraries.
1 |
sudo apt-get install mesa-common-dev libglu1-mesa-dev libegl1-mesa-dev |
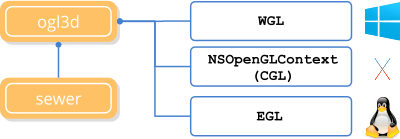
1. 3D Contexts
Use ogl3d_context to create a context.
Use ogl3d_destroy to destroy a context.
A 3D context represents a set of states and objects within which OpenGL drawing operations are performed (Figure 2). Basically a context includes:
- States: Current settings such as projection mode, transformation matrices, lighting options, etc.
- Shaders: Programs that allow you to customize the rendering within the GPU.
- Geometry and textures: Graphic resources that represent the objects to be rendered.
- Graphic buffers: Color, depth, stencil, and others that are used to store pixel information during the rendering process.
- Windows: Integration with the operating system to draw in a specific window.
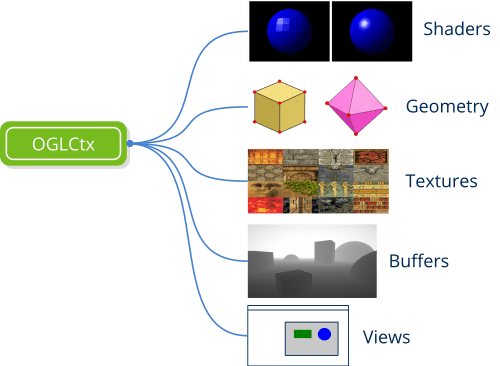
To create a context you will need the native identifier of the associated view. That is, a HWND
object on Windows, a GtkWidget
on Linux or a NSView
on macOS. In NAppGUI, this is provided by the view_native function (Listing 1).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
View *view = .... void *nview = view_native(view); OGLCtx *oglctx = NULL; OGLProps props; oglerr_t err; props.api = ekOGL_3_3; props.hdaccel = TRUE; props.color_bpp = 32; props.depth_bpp = 0; props.stencil_bpp = 0; props.aux_buffers = 0; props.transparent = FALSE; props.shared = NULL; oglctx = ogl3d_context(&props, nview, &err); if (oglctx == NULL) { bstd_printf("Error: %s\n", ogl3d_err_str(err)); } |
2. Drawing operation
- Use ogl3d_begin_draw when starting rendering.
- Use ogl3d_end_draw when finishing rendering.
The View
object will raise an OnDraw
event every time it needs to update the drawing area (Draw in views). In the handler for this event, we must include the OpenGL code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
static void i_OnDraw(App *app, Event *e) { const EvDraw *p = event_params(e, EvDraw); ogl3d_begin_draw(app->oglctx); // OpenGL Code (cross-platform) glViewport(0, 0, (GLsizei)p->width, (GLsizei)p->height); glClearColor(.8f, .8f, .8f, 1.0f); glClear(GL_COLOR_BUFFER_BIT); glMatrixMode(GL_MODELVIEW); glLoadIdentity(); glBegin(GL_TRIANGLES); glColor3f(1, 0, 0); glVertex3f(0, 1, 0); glColor3f(0, 1, 0); glVertex3f(-1, -1, 0); glColor3f(0, 0, 1); glVertex3f(1, -1, 0); glEnd(); ogl3d_end_draw(ogl->ctx); } ... view_OnDraw(view, listener(app, i_OnDraw, App)); |
3. GLEW
OpenGL allows the incorporation of optional extensions that are not included in the core of the standard. These extensions allow hardware manufacturers (such as NVIDIA, AMD, Intel, etc.) and software developers to add new functionality or improve the performance of existing features. In general, detecting whether an extension is present or not when creating a context implies a great workload for the programmer, which is why different libraries have been created for this purpose.
OGL3D includes a copy of GLEW (OpenGL Extension Wrangler Library) which facilitates this task enormously. Its initialization by calling glewInit()
is done automatically when creating the OGLCtx
object. We will only have to include this header before making any call to OpenGL (Listing 3).
1 2 3 4 5 6 7 8 9 10 11 |
// Include OpenGL and GLEW #include "nowarn.hxx" #include <ogl3d/glew.h> #include "warn.hxx" ... // OpenGL calls glViewport(0, 0, (GLsizei)width, (GLsizei)height); glClearColor(.8f, .8f, .8f, 1.0f); glClear(GL_COLOR_BUFFER_BIT); glMatrixMode(GL_MODELVIEW); ... |
To check if an extension is present, we can use glewIsSupported()
or the different macros provided by GLEW (Listing 4).
1 2 3 4 5 6 7 8 9 |
if (glewIsSupported("GL_ARB_vertex_program")) { // Extension available } ... if (GLEW_ARB_vertex_program) { // Extension available } |
enum oglapi_t
OpenGL version.
enum oglapi_t
{
ekOGL_1_1,
ekOGL_1_2,
ekOGL_1_2_1,
ekOGL_1_3,
ekOGL_1_4,
ekOGL_1_5,
ekOGL_2_0,
ekOGL_2_1,
ekOGL_3_0,
ekOGL_3_1,
ekOGL_3_2,
ekOGL_3_3,
ekOGL_4_0,
ekOGL_4_1,
ekOGL_4_2,
ekOGL_4_3,
ekOGL_4_4,
ekOGL_4_5,
ekOGL_4_6
};
ekOGL_1_1 | OpenGL 1.1. |
ekOGL_1_2 | OpenGL 1.2. |
ekOGL_1_2_1 | OpenGL 1.2.1. |
ekOGL_1_3 | OpenGL 1.3. |
ekOGL_1_4 | OpenGL 1.4. |
ekOGL_1_5 | OpenGL 1.5. |
ekOGL_2_0 | OpenGL 2.0. |
ekOGL_2_1 | OpenGL 2.1. |
ekOGL_3_0 | OpenGL 3.0. |
ekOGL_3_1 | OpenGL 3.1. |
ekOGL_3_2 | OpenGL 3.2. |
ekOGL_3_3 | OpenGL 3.3. |
ekOGL_4_0 | OpenGL 4.0. |
ekOGL_4_1 | OpenGL 4.1. |
ekOGL_4_2 | OpenGL 4.2. |
ekOGL_4_3 | OpenGL 4.3. |
ekOGL_4_4 | OpenGL 4.4. |
ekOGL_4_5 | OpenGL 4.5. |
ekOGL_4_6 | OpenGL 4.6. |
enum oglerr_t
Error codes in OpenGL.
enum oglerr_t
{
ekOGLAPIVERS,
ekOGLFULLSCN,
ekOGLVIEW,
ekOGLPIXFORMAT,
ekOGLCONTEXT,
ekOGLGLEW,
ekOGLOK
};
ekOGLAPIVERS | Unsupported version. |
ekOGLFULLSCN | Full screen mode not supported. |
ekOGLVIEW | Error binding context to view. |
ekOGLPIXFORMAT | Pixel format not supported. |
ekOGLCONTEXT | Error creating context. |
ekOGLGLEW | Error when initializing GLEW. |
ekOGLOK | No error. |
struct OGLProps
Properties of the OpenGL context, necessary for its creation.
struct OGLProps { oglapi_t api; bool_t hdaccel; uint32_t color_bpp; uint32_t depth_bpp; uint32_t stencil_bpp; uint32_t aux_buffers; bool_t transparent; OGLCtx* shared; };
api | Required API version. |
hdaccel | Hardware 3D acceleration required. Normally |
color_bpp | Number of bits per pixel of the color buffer. Normally 32. |
depth_bpp | Number of bits per pixel of the depth buffer. 8, 16, 24, 32. If 0, the buffer is disabled. |
stencil_bpp | Number of bits per pixel of the stencil buffer. 8, 16, 24, 32. If 0, the buffer is disabled. |
aux_buffers | Number of auxiliary color buffers. Normally 0. |
transparent | If |
shared | Context with which to share graphic objects. Normally |
struct OGLCtx
OpenGL context.
struct OGLCtx;
ogl3d_start ()
Start the OGL3D library.
void
ogl3d_start(void);
Remarks
Call this function before any other in OGL3D.
ogl3d_finish ()
Finalize the OGL3D library.
void
ogl3d_finish(void);
Remarks
Call this function before closing the program or when you no longer need to use OGL3D.
ogl3d_context ()
Creates an OpenGL context and associates it with a view.
OGLCtx* ogl3d_context(const OGLProps *props, void *view, oglerr_t *err);
props | Desired properties for the context. |
view | Native view handler. |
err | Error code. It can be |
Return
Newly created context or NULL
if there has been an error.
Remarks
See 3D Contexts.
ogl3d_destroy ()
Destroys an OpenGL context.
void ogl3d_destroy(OGLCtx **ogl);
ogl | Context to destroy. The pointer will be set to |
ogl3d_begin_draw ()
Starts a drawing operation with OpenGL.
void ogl3d_begin_draw(OGLCtx *ogl);
ogl | Context. |
Remarks
See Drawing operation.
ogl3d_end_draw ()
Completes an OpenGL drawing operation.
void ogl3d_end_draw(OGLCtx *ogl);
ogl | Context. |
Remarks
See Drawing operation.
ogl3d_set_size ()
Updates the context size in pixels. This function must be called every time the view is resized.
void ogl3d_set_size(OGLCtx *ogl, const uint32_t width, const uint32_t height);
ogl | Context. |
width | Width of the view in pixels. |
height | Height of the view in pixels. |
ogl3d_err_str ()
Returns an explanatory text, associated with an error code.
const char_t* ogl3d_err_str(const oglerr_t err);
err | Error code. |
Return
Text string with the error.