Geom2D Entities Drawing
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
void | draw_v2d (...) |
void | draw_seg2d (...) |
void | draw_r2d (...) |
void | draw_cir2d (...) |
void | draw_box2d (...) |
void | draw_obb2d (...) |
void | draw_tri2d (...) |
void | draw_pol2d (...) |
In the previous section we have seen the basic primitives for drawing in 2D. However, Draw2D has specialized functions for Geom2D objects. These new functions would be totally dispensable, since you could get the same result using draw_rect
, draw_polygon
, etc. They are included as a mere shortcut, in addition to offering a version of them based on Math templates, very useful when developing generic algorithms in C++. The line and fill properties will be those that are in effect at any given time within the context, due to: draw_line_color
, draw_line_width
, draw_fill_color
, etc..
- Use draw_v2df to draw a point.
- Use draw_seg2df to draw a segment.
- Use draw_r2df to draw a rectangle.
- Use draw_cir2df to draw a circle.
- Use draw_box2df to draw an aligned box.
- Use draw_obb2df to draw an oriented box.
- Use draw_tri2df to draw a triangle.
- Use draw_pol2df to draw a polygon.
You can find a complete example of the use of 2D entities in Col2DHello (Figure 1). In addition to drawing, this application shows other concepts related to graphics and geometric calculation such as:
- Create 2D objects on demand.
- Click+Drag interactivity.
- Collision detection.
- Calculation of areas.
- Triangulation of polygons and decomposition into convex components.
- Calculation of the optimal circle that surrounds a set of points.
- Calculation of the oriented box (
OBB2Df
) that best represents a set of points. - Calculation of the Convex Hull.
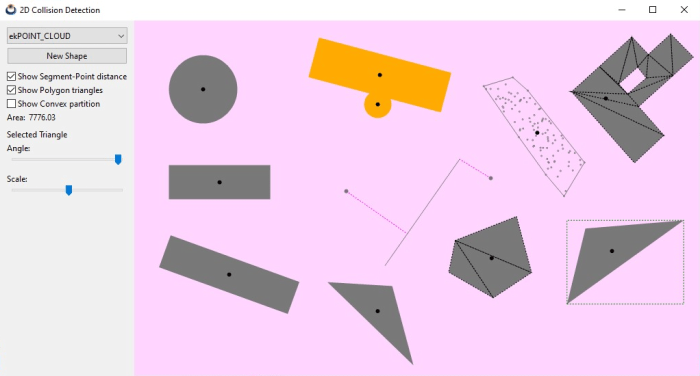
draw_v2d ()
Draw a 2D point.
void draw_v2df(DCtx *ctx, const drawop_t op, const V2Df *v2d, const real32_t radius); void draw_v2dd(DCtx *ctx, const drawop_t op, const V2Dd *v2d, const real64_t radius); void Draw::v2d(DCtx *ctx, const drawop_t op, const V2D *v2d, const real radius);
ctx | Drawing context. |
op | Drawing operation. |
v2d | Point. |
radius | Radius. |
draw_seg2d ()
Draw a 2D segment.
void draw_seg2df(DCtx *ctx, const Seg2Df *seg); void draw_seg2dd(DCtx *ctx, const Seg2Dd *seg); void Draw::seg2d(DCtx *ctx, const Seg2D *seg);
ctx | Drawing context. |
seg | Segment. |
draw_r2d ()
Draw a 2D rectangle.
void draw_r2df(DCtx *ctx, const drawop_t op, const R2Df *rect); void draw_r2dd(DCtx *ctx, const drawop_t op, const R2Dd *rect); void Draw::r2d(DCtx *ctx, const drawop_t op, const R2D *rect);
ctx | Drawing context. |
op | Drawing operation. |
rect | Rectangle. |
draw_cir2d ()
Draw a 2D circle.
void draw_cir2df(DCtx *ctx, const drawop_t op, const Cir2Df *cir); void draw_cir2dd(DCtx *ctx, const drawop_t op, const Cir2Dd *cir); void Draw::cir2d(DCtx *ctx, const drawop_t op, const Cir2D *cir);
ctx | Drawing context. |
op | Drawing operation. |
cir | Circle. |
draw_box2d ()
Draw a 2D box.
void draw_box2df(DCtx *ctx, const drawop_t op, const Box2Df *box); void draw_box2dd(DCtx *ctx, const drawop_t op, const Box2Dd *box); void Draw::box2d(DCtx *ctx, const drawop_t op, const Box2D *box);
ctx | Drawing context. |
op | Drawing operation. |
box | Aligned box. |
draw_obb2d ()
Draw an oriented 2D box.
void draw_obb2df(DCtx *ctx, const drawop_t op, const OBB2Df *obb); void draw_obb2dd(DCtx *ctx, const drawop_t op, const OBB2Dd *obb); void Draw::obb2d(DCtx *ctx, const drawop_t op, const OBB2D *obb);
ctx | Drawing context. |
op | Drawing operation. |
obb | Oriented box. |
draw_tri2d ()
Draw a 2D triangle.
void draw_tri2df(DCtx *ctx, const drawop_t op, const Tri2Df *tri); void draw_tri2dd(DCtx *ctx, const drawop_t op, const Tri2Dd *tri); void Draw::tri2d(DCtx *ctx, const drawop_t op, const Tri2D *tri);
ctx | Drawing context. |
op | Drawing operation. |
tri | Triangle. |
draw_pol2d ()
Draw a 2D polygon.
void draw_pol2df(DCtx *ctx, const drawop_t op, const Pol2Df *pol); void draw_pol2dd(DCtx *ctx, const drawop_t op, const Pol2Dd *pol); void Draw::pol2d(DCtx *ctx, const drawop_t op, const Pol2D *pol);
ctx | Drawing context. |
op | Drawing operation. |
pol | Polygon. |