2D Collisions
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Header
#include <geom2d/col2d.h>
Functions
bool_t | col2d_point_point (...) |
bool_t | col2d_segment_point (...) |
bool_t | col2d_segment_segment (...) |
bool_t | col2d_circle_point (...) |
bool_t | col2d_circle_segment (...) |
bool_t | col2d_circle_circle (...) |
bool_t | col2d_box_point (...) |
bool_t | col2d_box_segment (...) |
bool_t | col2d_box_circle (...) |
bool_t | col2d_box_box (...) |
bool_t | col2d_obb_point (...) |
bool_t | col2d_obb_segment (...) |
bool_t | col2d_obb_circle (...) |
bool_t | col2d_obb_box (...) |
bool_t | col2d_obb_obb (...) |
bool_t | col2d_tri_point (...) |
bool_t | col2d_tri_segment (...) |
bool_t | col2d_tri_circle (...) |
bool_t | col2d_tri_box (...) |
bool_t | col2d_tri_obb (...) |
bool_t | col2d_tri_tri (...) |
bool_t | col2d_poly_point (...) |
bool_t | col2d_poly_segment (...) |
bool_t | col2d_poly_circle (...) |
bool_t | col2d_poly_box (...) |
bool_t | col2d_poly_obb (...) |
bool_t | col2d_poly_tri (...) |
bool_t | col2d_poly_poly (...) |
Collision detection is responsible for studying and developing algorithms that check if two geometric objects intersect at some point. As the general case would be quite complex to implement and inefficient to evaluate, a series of collision volumes (Figure 1) are defined that will enclose the original sets and where the tests can be significantly simplified. The use of these most elementary forms is usually known as broad phase collision detection (Figure 2), since it seeks to detect "non-collision" as quickly as possible. In Hello 2D Collisions! you have an example application.
- Use col2d_poly_obbf to detect the collision between an oriented box and a polygon.
- Use col2d_tri_trif to detect the collision between two triangles.
- Use col2d_circle_segmentf to detect the collision between a circle and a segment.
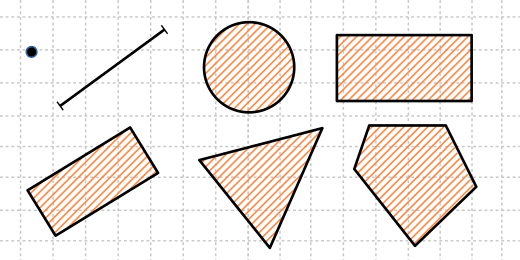
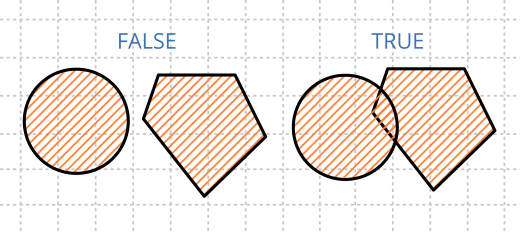
Col2D provides functions to check each pair of previously presented collision volumes. Most of these methods use the Separation Axis Theorem (Figure 3). This theorem indicates, in essence, that if it is possible to find a line where the projections of the vertices do not intersect, then the figures do not intersect. In the specific case of convex polygons, it is only necessary to evaluate n lines, where n is the number of sides of the polygon.
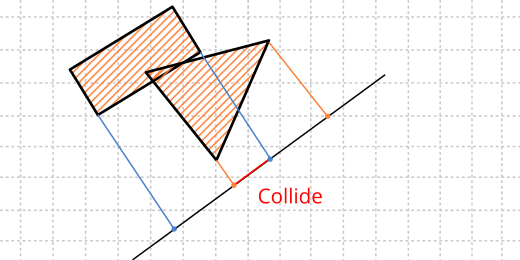
col2d_point_point ()
Point-point collision.
bool_t col2d_point_pointf(const V2Df *pnt1, const V2Df *pnt2, const real32_t tol, Col2Df *col); bool_t col2d_point_pointd(const V2Dd *pnt1, const V2Dd *pnt2, const real64_t tol, Col2Dd *col); bool_t Col2D::point_point(const V2D *pnt1, const V2D *pnt2, const real tol, Col2D *col);
pnt1 | First point. |
pnt2 | Second point. |
tol | Tolerance. Minimum distance to be considered a collision. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_segment_point ()
Segment-point collision.
bool_t col2d_segment_pointf(const Seg2Df *seg, const V2Df *pnt, const real32_t tol, Col2Df *col); bool_t col2d_segment_pointd(const Seg2Dd *seg, const V2Dd *pnt, const real64_t tol, Col2Dd *col); bool_t Col2D::segment_point(const Seg2D *seg, const V2D *pnt, const real tol, Col2D *col);
seg | Segment. |
pnt | Point. |
tol | Tolerance. Minimum distance to be considered a collision. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_segment_segment ()
Segment-segment collision.
bool_t col2d_segment_segmentf(const Seg2Df *seg1, const Seg2Df *seg2, Col2Df *col); bool_t col2d_segment_segmentd(const Seg2Dd *seg1, const Seg2Dd *seg2, Col2Dd *col); bool_t Col2D::segment_segment(const Seg2D *seg1, const Seg2D *seg2, Col2D *col);
seg1 | First segment. |
seg2 | Second segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_circle_point ()
Circle-point collision.
bool_t col2d_circle_pointf(const Cir2Df *cir, const V2Df *pnt, Col2Df *col); bool_t col2d_circle_pointd(const Cir2Dd *cir, const V2Dd *pnt, Col2Dd *col); bool_t Col2D::circle_point(const Cir2D *cir, const V2D *pnt, Col2D *col);
cir | Circle. |
pnt | Point. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_circle_segment ()
Circle-segment collision.
bool_t col2d_circle_segmentf(const Cir2Df *cir, const Seg2Df *seg, Col2Df *col); bool_t col2d_circle_segmentd(const Cir2Dd *cir, const Seg2Dd *seg, Col2Dd *col); bool_t Col2D::circle_segment(const Cir2D *cir, const Seg2D *seg, Col2D *col);
cir | Circle. |
seg | Segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_circle_circle ()
Circle-circle collision.
bool_t col2d_circle_circlef(const Cir2Df *cir1, const Cir2Df *cir2, Col2Df *col); bool_t col2d_circle_circled(const Cir2Dd *cir1, const Cir2Dd *cir2, Col2Dd *col); bool_t Col2D::circle_circle(const Cir2D *cir1, const Cir2D *cir2, Col2D *col);
cir1 | First circle. |
cir2 | Second circle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_box_point ()
Box-point collision.
bool_t col2d_box_pointf(const Box2Df *box, const V2Df *pnt, Col2Df *col); bool_t col2d_box_pointd(const Box2Dd *box, const V2Dd *pnt, Col2Dd *col); bool_t Col2D::box_point(const Box2D *box, const V2D *pnt, Col2D *col);
box | Box. |
pnt | Point. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_box_segment ()
Box-segment collision.
bool_t col2d_box_segmentf(const Box2Df *box, const Seg2Df *seg, Col2Df *col); bool_t col2d_box_segmentd(const Box2Dd *box, const Seg2Dd *seg, Col2Dd *col); bool_t Col2D::box_segment(const Box2D *box, const Seg2D *seg, Col2D *col);
box | Box. |
seg | Segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_box_circle ()
Box-circle collision.
bool_t col2d_box_circlef(const Box2Df *box, const Cir2Df *cir, Col2Df *col); bool_t col2d_box_circled(const Box2Dd *box, const Cir2Dd *cir, Col2Dd *col); bool_t Col2D::box_circle(const Box2D *box, const Cir2D *cir, Col2D *col);
box | Box. |
cir | Circle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_box_box ()
Box-box collision.
bool_t col2d_box_boxf(const Box2Df *box1, const Box2Df *box2, Col2Df *col); bool_t col2d_box_boxd(const Box2Dd *box1, const Box2Dd *box2, Col2Dd *col); bool_t Col2D::box_box(const Box2D *box1, const Box2D *box2, Col2D *col);
box1 | First box. |
box2 | Second box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_obb_point ()
Point-oriented box collision.
bool_t col2d_obb_pointf(const OBB2Df *obb, const V2Df *pnt, Col2Df *col); bool_t col2d_obb_pointd(const OBB2Dd *obb, const V2Dd *pnt, Col2Dd *col); bool_t Col2D::obb_point(const OBB2D *obb, const V2D *pnt, Col2D *col);
obb | Oriented box. |
pnt | Point. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_obb_segment ()
Segment-oriented box collision.
bool_t col2d_obb_segmentf(const OBB2Df *obb, const Seg2Df *seg, Col2Df *col); bool_t col2d_obb_segmentd(const OBB2Dd *obb, const Seg2Dd *seg, Col2Dd *col); bool_t Col2D::obb_segment(const OBB2D *obb, const Seg2D *seg, Col2D *col);
obb | Oriented box. |
seg | Segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_obb_circle ()
Collision-oriented box-circle.
bool_t col2d_obb_circlef(const OBB2Df *obb, const Cir2Df *cir, Col2Df *col); bool_t col2d_obb_circled(const OBB2Dd *obb, const Cir2Dd *cir, Col2Dd *col); bool_t Col2D::obb_circle(const OBB2D *obb, const Cir2D *cir, Col2D *col);
obb | Oriented box. |
cir | Circle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_obb_box ()
Box-oriented box collision.
bool_t col2d_obb_boxf(const OBB2Df *obb, const Box2Df *box, Col2Df *col); bool_t col2d_obb_boxd(const OBB2Dd *obb, const Box2Dd *box, Col2Dd *col); bool_t Col2D::obb_box(const OBB2D *obb, const Box2D *box, Col2D *col);
obb | Oriented box. |
box | Aligned box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_obb_obb ()
Oriented Box-Oriented Box collision.
bool_t col2d_obb_obbf(const OBB2Df *obb1, const OBB2Df *obb2, Col2Df *col); bool_t col2d_obb_obbd(const OBB2Dd *obb1, const OBB2Dd *obb2, Col2Dd *col); bool_t Col2D::obb_obb(const OBB2D *obb1, const OBB2D *obb2, Col2D *col);
obb1 | First oriented box. |
obb2 | Second oriented box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_point ()
Triangle-point collision.
bool_t col2d_tri_pointf(const Tri2Df *tri, const V2Df *pnt, Col2Df *col); bool_t col2d_tri_pointd(const Tri2Dd *tri, const V2Dd *pnt, Col2Dd *col); bool_t Col2D::tri_point(const Tri2D *tri, const V2D *pnt, Col2D *col);
tri | Triangle. |
pnt | Point. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_segment ()
Triangle-segment collision.
bool_t col2d_tri_segmentf(const Tri2Df *tri, const Seg2Df *seg, Col2Df *col); bool_t col2d_tri_segmentd(const Tri2Dd *tri, const Seg2Dd *seg, Col2Dd *col); bool_t Col2D::tri_segment(const Tri2D *tri, const Seg2D *seg, Col2D *col);
tri | Triangle. |
seg | Segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_circle ()
Triangle-circle collision.
bool_t col2d_tri_circlef(const Tri2Df *tri, const Cir2Df *cir, Col2Df *col); bool_t col2d_tri_circled(const Tri2Dd *tri, const Cir2Dd *cir, Col2Dd *col); bool_t Col2D::tri_circle(const Tri2D *tri, const Cir2D *cir, Col2D *col);
tri | Triangle. |
cir | Circle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_box ()
Triangle-box collision.
bool_t col2d_tri_boxf(const Tri2Df *tri, const Box2Df *box, Col2Df *col); bool_t col2d_tri_boxd(const Tri2Dd *tri, const Box2Dd *box, Col2Dd *col); bool_t Col2D::tri_box(const Tri2D *tri, const Box2D *box, Col2D *col);
tri | Triangle. |
box | Aligned box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_obb ()
Triangle-oriented box collision.
bool_t col2d_tri_obbf(const Tri2Df *tri, const OBB2Df *obb, Col2Df *col); bool_t col2d_tri_obbd(const Tri2Dd *tri, const OBB2Dd *obb, Col2Dd *col); bool_t Col2D::tri_obb(const Tri2D *tri, const OBB2D *obb, Col2D *col);
tri | Triangle. |
obb | Oriented box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_tri_tri ()
Triangle-triangle collision.
bool_t col2d_tri_trif(const Tri2Df *tri1, const Tri2Df *tri2, Col2Df *col); bool_t col2d_tri_trid(const Tri2Dd *tri1, const Tri2Dd *tri2, Col2Dd *col); bool_t Col2D::tri_tri(const Tri2D *tri1, const Tri2D *tri2, Col2D *col);
tri1 | First triangle. |
tri2 | Second triangle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_point ()
Polygon-point collision.
bool_t col2d_poly_pointf(const Pol2Df *pol, const V2Df *pnt, Col2Df *col); bool_t col2d_poly_pointd(const Pol2Dd *pol, const V2Dd *pnt, Col2Dd *col); bool_t Col2D::poly_point(const Pol2D *pol, const V2D *pnt, Col2D *col);
pol | Polygon. |
pnt | Point. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_segment ()
Polygon-segment collision.
bool_t col2d_poly_segmentf(const Pol2Df *pol, const Seg2Df *seg, Col2Df *col); bool_t col2d_poly_segmentd(const Pol2Dd *pol, const Seg2Dd *seg, Col2Dd *col); bool_t Col2D::poly_segment(const Pol2D *pol, const Seg2D *seg, Col2D *col);
pol | Polygon. |
seg | Segment. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_circle ()
Polygon-circle collision.
bool_t col2d_poly_circlef(const Pol2Df *pol, const Cir2Df *cir, Col2Df *col); bool_t col2d_poly_circled(const Pol2Dd *pol, const Cir2Dd *cir, Col2Dd *col); bool_t Col2D::poly_circle(const Pol2D *pol, const Cir2D *cir, Col2D *col);
pol | Polygon. |
cir | Circle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_box ()
Polygon-box collision.
bool_t col2d_poly_boxf(const Pol2Df *pol, const Box2Df *cir, Col2Df *col); bool_t col2d_poly_boxd(const Pol2Dd *pol, const Box2Dd *cir, Col2Dd *col); bool_t Col2D::poly_box(const Pol2D *pol, const Box2D *cir, Col2D *col);
pol | Polygon. |
cir | Box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_obb ()
Polygon-box collision.
bool_t col2d_poly_obbf(const Pol2Df *pol, const OBB2Df *cir, Col2Df *col); bool_t col2d_poly_obbd(const Pol2Dd *pol, const OBB2Dd *cir, Col2Dd *col); bool_t Col2D::poly_obb(const Pol2D *pol, const OBB2D *cir, Col2D *col);
pol | Polygon. |
cir | Oriented box. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_tri ()
Polygon-triangle collision.
bool_t col2d_poly_trif(const Pol2Df *pol, const Tri2Df *tri, Col2Df *col); bool_t col2d_poly_trid(const Pol2Dd *pol, const Tri2Dd *tri, Col2Dd *col); bool_t Col2D::poly_tri(const Pol2D *pol, const Tri2D *tri, Col2D *col);
pol | Polygon. |
tri | Triangle. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.
col2d_poly_poly ()
Polygon-polygon collision.
bool_t col2d_poly_polyf(const Pol2Df *pol1, const Pol2Df *pol2, Col2Df *col); bool_t col2d_poly_polyd(const Pol2Dd *pol1, const Pol2Dd *pol2, Col2Dd *col); bool_t Col2D::poly_poly(const Pol2D *pol1, const Pol2D *pol2, Col2D *col);
pol1 | First polygon. |
pol2 | Second polygon. |
col | Detailed data of the collision. It can be |
Return
TRUE
if the objects intersect, FALSE
otherwise.