Asserts
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
The asserts will perform dynamic code checks when the program is running.
Functions
void | cassert (...) |
void | cassert_msg (...) |
void | cassert_fatal (...) |
void | cassert_fatal_msg (...) |
void | cassert_no_null (...) |
void | cassert_no_nullf (...) |
void | cassert_default (void) |
void | cassert_set_func (...) |
asserts are sentences distributed by the source code that perform an intensive Dynamic analysis, helping to detect errors at runtime. When the assert condition becomes FALSE
, the program execution stops and a warning window is displayed (Figure 1).
- Use cassert to introduce a dynamic check in your code.
- Use cassert_no_null once you have to access the content of a pointer.
1 2 3 4 5 6 |
void layout_vmargin(Layout *layout, const uint32_t row, const real32_t margin) { cassert_no_null(layout); cassert_msg(row < layout->num_rows, "'row' out of range"); ... } |
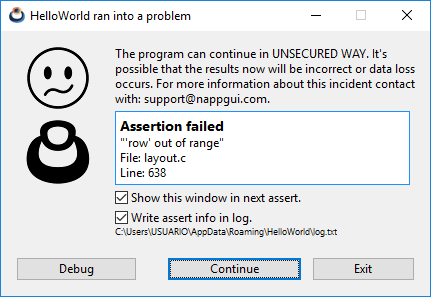
At this time we have three alternatives:
- Debug: Debug the program: Access the call stack, inspect variables, etc. More in Debugging the program.
- Continue: Continue with the execution, ignoring the assert.
- Exit: Exit the program.
To avoid showing this window in futher asserts, deactivate the check 'Show this window in next assert'
. Future incidents will be directed to a log file. You can also omit dumps in this log, deactivating 'Write assert info in log'
.
asserts sentences provide very valuable information about program anomalies and should never be ignored.
In the previous example we have seen a "continuable" assert, that is, the execution of the program can continue if we press [Continue]
. However, as we indicated, they should not be ignored indefinitely. On the other hand we have the critical asserts (Figure 2). Normally they are related to segment violation problems, where it will not be possible to continue running the program.
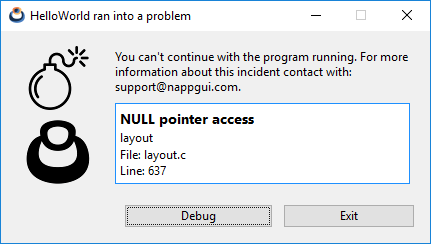
cassert ()
Basic assert sentence. If the condition is evaluated at FALSE
, a "continuable" assert will be launched. The message shown will be the literal of the condition itself.
void cassert(bool_t cond);
1 2 3 |
// "row < arrpt_size(layout->rows)" // will be shown in the assert window cassert(row < arrpt_size(layout->rows)); |
cond | Boolean expression. |
cassert_msg ()
Same as the cassert()
sentence, but using a custom message, instead of the literal condition.
void cassert_msg(bool_t cond, const char_t *msg);
1 2 3 |
// "'row' out of range" // will be shown in the assert window cassert_msg(layout < layout->num_rows, "'row' out of range"); |
cond | Boolean expression. |
msg | Message related to the assert. |
cassert_fatal ()
Same as the cassert()
sentence, but throwing a critical assert (not "continuable").
void cassert_fatal(bool_t cond);
1 2 3 |
// "gravity > 0." // will be shown in the assert window cassert_fatal(gravity > 0.); |
cond | Boolean expression. |
cassert_fatal_msg ()
Same as the cassert_msg()
sentence, but throwing a critical assert (not "continuable").
void cassert_fatal_msg(bool_t cond, const char_t *msg);
1 2 3 |
// "'gravity' can't be negative." // will be shown in the assert window cassert_fatal_msg(gravity > 0., "'gravity' can't be negative"); |
cond | Boolean expression. |
msg | Message related to the assert. |
cassert_no_null ()
Triggers a critical assert if a pointer has NULL
value.
void cassert_no_null(void *ptr);
ptr | Pointer to evaluate. |
cassert_no_nullf ()
Triggers a critical assert if a function pointer has NULL
value.
void cassert_no_nullf(void *fptr);
fptr | Pointer to evaluate. |
cassert_default ()
Triggers a "continuable" assert if the switch statement reaches the default:
state. Useful to ensure that, for example, all the values of an enum have been considered.
void
cassert_default(void);
1 2 3 4 5 6 7 8 9 10 |
switch(align) { case LEFT: // Do something break; case RIGHT: // Do something break; // Others are not allowed. cassert_default(); } |
cassert_set_func ()
Set a custom function to execute an alternative code when an assert occurs. By default, in desktop applications, an informative window is displayed (Figure 1) and the message is saved in a Log file.
void cassert_set_func(void *data, FPtr_assert func_assert);
data | User data or application context. |
func_assert | Callback function called after the activation of an assert. |
Remarks
When using this function, the previous asserts management will be deactivated.