Files and directories
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Access to the local file system of the computer.
Functions
uint32_t | bfile_dir_work (...) |
bool_t | bfile_dir_set_work (...) |
uint32_t | bfile_dir_home (...) |
uint32_t | bfile_dir_data (...) |
uint32_t | bfile_dir_exec (...) |
uint32_t | bfile_dir_tmp (...) |
bool_t | bfile_dir_create (...) |
Dir* | bfile_dir_open (...) |
void | bfile_dir_close (...) |
bool_t | bfile_dir_get (...) |
bool_t | bfile_dir_delete (...) |
File* | bfile_create (...) |
File* | bfile_open (...) |
void | bfile_close (...) |
bool_t | bfile_lstat (...) |
bool_t | bfile_fstat (...) |
bool_t | bfile_read (...) |
bool_t | bfile_write (...) |
bool_t | bfile_seek (...) |
uint64_t | bfile_pos (...) |
bool_t | bfile_delete (...) |
bool_t | bfile_rename (...) |
1. File System
The file system (filesystem) is the hierarchical structure composed of directories and files that allows organizing the persistent data of the computer (Figure 1). It is something with which computer users are very familiar, especially after the emergence of graphic systems that introduced the analogy of desktop, folder and document. It starts in a directory called root (/
on Unix or C:\
on Windows) and, from here, all sub-directories and files hang down forming a tree that grows deep. At the programming level, the file system is managed through system calls that allow directories to be created, browse their content, open files, delete them, obtain attributes, etc.
- Use bfile_create to create a new file.
- Use bfile_dir_create to create a directory.
- Use bfile_dir_open to open a directory to explore its contents.
- Use bfile_dir_get to get information about a directory entry.
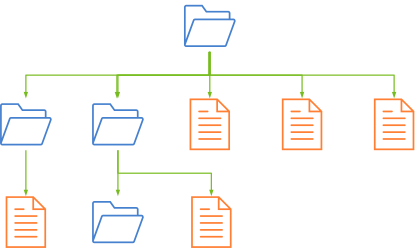
2. Files and data streams
A process can read or write data to a file after opening an I/O (Streams) which provides a stream of binary data to or from the process itself (Figure 2). There is a pointer that moves sequentially each time data is read or written. It is initially in byte 0, but we can modify it to access random positions in the file without reading the content (Figure 3). This can be very useful when working with large files whose data is indexed in some way.
- Use bfile_open to open an existing file.
- Use bfile_read to read binary data from a file.
- Use bfile_write to write binary data to a file.
- Use bfile_seek to modify the file pointer.
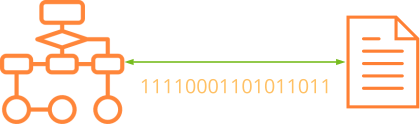
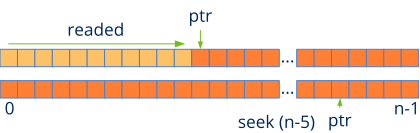
3. Filename and pathname
These two concepts are recurrent and widely used by API functions that manipulate files. When we navigate through the contents of a directory bfile_dir_get, we obtain a sequence of filenames that is the "flat" name of the element (file or subdirectory) without including its path within the file system (without characters '/'
or '\'
). On the other hand the pathname is a sequence of one or several filenames separated by '/', '\'
, which indicates the way forward to locate a certain element. This path can be absolute when it starts with the root directory (C:\Users\john\docs\images\party.png
) or relative (docs\images\party.png
) when it indicates the partial route from the process current working directory.
- Use bfile_dir_work to get the current working directory.
- Use bfile_dir_set_work to set the working directory.
4. Home and AppData
These are two typical directories used by applications to store files relative to a particular user. On the one hand, home indicates the personal directory of the user currently registered in the system, typically C:\Users\john
(Windows), /home/john
(Linux) or /Users/john
(macOS). On the other hand appdata is a directory reserved for saving temporary or configuration data of applications. Typical locations can be C:\Users\john\AppData\Roaming
(Windows), /home/john/.config
(Linux) or /User/john/Library
(macOS). The usual thing will be to create a sub-folder with the name of the application /User/john/Library/TheApp
.
- Use bfile_dir_home to get the user home directory.
- Use bfile_dir_data to get the application data directory.
- Use bfile_dir_exec to get the current executable directory.
bfile_dir_work ()
Gets the current working directory of the process. It is the directory from which the relative pathnames will be interpreted.
uint32_t bfile_dir_work(char_t *pathname, const uint32_t size);
pathname | Buffer where the directory will be written. |
size | Size in bytes of the buffer |
Return
The number of bytes written in pathname
, including the null character '\0'
.
Remarks
bfile_dir_set_work ()
Change the current working directory of the application. The relative pathnames will be interpreted from here.
bool_t bfile_dir_set_work(const char_t *pathname, ferror_t *error);
pathname | The name of the directory. |
error | Error code if the function fails. Can be |
Return
TRUE
if the working directory has changed, FALSE
if there have been any errors.
Remarks
bfile_dir_home ()
Get the home
directory of the current user.
uint32_t bfile_dir_home(char_t *pathname, const uint32_t size);
pathname | Buffer where the directory will be written. |
size | Size in bytes of the buffer |
Return
The number of bytes written in pathname
, including the null character '\0'
.
Remarks
bfile_dir_data ()
Gets the AppData directory where application configuration data can be saved.
uint32_t bfile_dir_data(char_t *pathname, const uint32_t size);
pathname | Buffer where the directory will be written. |
size | Size in bytes of the buffer |
Return
The number of bytes written in pathname
, including the null character '\0'
.
Remarks
bfile_dir_exec ()
Gets the absolute pathname of the current executable.
uint32_t bfile_dir_exec(char_t *pathname, const uint32_t size);
1 2 3 |
char_t path[512]; bfile_dir_exec(path, 512); path = "C:\Program Files\TheApp\theapp.exe" |
pathname | Buffer where the directory will be written. |
size | Size in bytes of the buffer |
Return
The number of bytes written in pathname
, including the null character '\0'
.
bfile_dir_tmp ()
Gets a directory to store temporary files.
uint32_t bfile_dir_tmp(char_t *pathname, const uint32_t size);
pathname | Buffer where the directory will be written. |
size | Size in bytes of the buffer |
Return
The number of bytes written in pathname
, including the null character '\0'
.
Remarks
Every system defines some path to store temporary files. For example /tmp/
on Linux/macOS systems or C:\Users\USER\AppData\Local\Temp\
on Windows. Files stored here can be deleted at any time by the system.
bfile_dir_create ()
Create a new directory. It will fail if any intermediate directory of pathname
does not exist.
bool_t bfile_dir_create(const char_t *pathname, ferror_t *error);
pathname | Name of the directory to be created, ending in a null character |
error | Error code if the function fails. Can be |
Return
TRUE
if the directory has been created, FALSE
if there have been any errors.
Remarks
hfile_dir_create create all intermediate directories at once.
bfile_dir_open ()
Open a directory to browse its contents. Then you have to use bfile_dir_get to iterate. The filename is not ordered under any criteria. At the end, you should call bfile_dir_close.
Dir* bfile_dir_open(const char_t *pathname, ferror_t *error);
pathname | Name of the directory, ending in a null character |
error | Error code if the function fails. Can be |
Return
The directory handler or NULL
if there has been an error.
bfile_dir_close ()
Close a previously open directory with bfile_dir_open.
void bfile_dir_close(Dir **dir);
dir | The directory handler. It will be set to |
bfile_dir_get ()
Gets the attributes of the current file when we go through a directory. Previously we have to open the directory with bfile_dir_open.
bool_t bfile_dir_get(Dir *dir, char_t *filename, const uint32_t size, file_type_t *type, uint64_t *fsize, Date *updated, ferror_t *error);
dir | Open directory handler. |
filename | Here will write the name of the file or sub-directory, ending in a null character |
size | Size in bytes of the |
type | Get the file type. Can be |
fsize | Gets the file size in bytes. Can be |
updated | Gets the date of the last update of the file. Can be |
error | Error code if the function fails. Can be |
Return
TRUE
if the file attributes have been read correctly. When there are no more files to go, it returns FALSE
with error=
ekFNOFILES.
Remarks
This function will advance to the next file within the open directory after obtaining the current item's data. If there is not enough space in name
, will return FALSE
with error=
ekFBIGNAME and will not advance to the next file. Use hfile_dir_loop to browse the contents of a directory more comfortably.
bfile_dir_delete ()
Delete a directory. It will fail if the directory is not completely empty. Use hfile_dir_destroy to completely and recursively erase a directory that may have content.
bool_t bfile_dir_delete(const char_t *pathname, ferror_t *error);
pathname | Name of the directory, ending in a null character |
error | Error code if the function fails. Can be |
Return
TRUE
if the directory has been deleted, FALSE
otherwise.
bfile_create ()
Create a new file. If previously it already exists its content will be erased. The new file will be opened for writing.
File* bfile_create(const char_t *pathname, ferror_t *error);
pathname | File name including its absolute or relative path. |
error | Error code if the function fails. Can be |
Return
The file handler or NULL
if there has been an error.
bfile_open ()
Open an existing file. Do not create it, if file does not exist this function will fail.
File* bfile_open(const char_t *pathname, const file_mode_t mode, ferror_t *error);
pathname | File name including its absolute or relative path. |
mode | Opening mode. |
error | Error code if the function fails. Can be |
Return
The file handler or NULL
if there has been an error.
bfile_close ()
Close a file previously opened with bfile_create or bfile_open.
void bfile_close(File **file);
file | File handler. It will be set to |
bfile_lstat ()
Get the attributes of a file through its pathname.
bool_t bfile_lstat(const char_t *pathname, file_type_t *type, uint64_t *fsize, Date *updated, ferror_t *error);
pathname | File name including its absolute or relative path. |
type | Get the file type. Can be |
fsize | Gets the file size in bytes. Can be |
updated | Gets the date of the last update of the file. Can be |
error | Error code if the function fails. Can be |
Return
TRUE
if it worked correctly, or FALSE
otherwise.
bfile_fstat ()
Get the attributes of a file through its handler.
bool_t bfile_fstat(File *file, file_type_t *type, uint64_t *fsize, Date *updated, ferror_t *error);
file | File manager. |
type | Get the file type. Can be |
fsize | Gets the file size in bytes. Can be |
updated | Gets the date of the last update of the file. Can be |
error | Error code if the function fails. Can be |
Return
TRUE
if it worked correctly, or FALSE
otherwise.
bfile_read ()
Read data from an open file.
bool_t bfile_read(File *file, byte_t *data, const uint32_t size, uint32_t *rsize, ferror_t *error);
file | File handler. |
data | Buffer where the read data will be written. |
size | The number of maximum bytes to read. |
rsize | Receive the number of bytes actually read. Can be |
error | Error code if the function fails. Can be |
Return
TRUE
if the data has been read correctly. If there is no more data (end of the file) it returns FALSE
with rsize = 0
and error=
ekFOK.
Remarks
File stream implements high-level functions for reading/writing files.
bfile_write ()
Write data in an open file.
bool_t bfile_write(File *file, const byte_t *data, const uint32_t size, uint32_t *wsize, ferror_t *error);
file | File handler. |
data | Buffer that contains the data to write. |
size | The number of bytes to write. |
wsize | It receives the number of bytes actually written. Can be |
error | Error code if the function fails. Can be |
Return
TRUE
if the data has been written, or FALSE
if there have been any errors.
Remarks
File stream implements high-level functions for reading/writing files.
bfile_seek ()
Move a file pointer to a new location.
bool_t bfile_seek(File *file, const int64_t offset, const file_seek_t whence, ferror_t *error);
file | File handler. |
offset | Number of bytes to move the pointer. Can be negative. |
whence | Pointer position from which |
error | Error code if the function fails. Can be |
Return
TRUE
if it worked correctly, FALSE
if not.
Remarks
It will return FALSE
and error
ekFSEEKNEG if the final pointer position is negative. It is not an error to set a pointer to a position beyond the end of the file. The file size does not increase until it is written to. A write operation increases the size of the file to the pointer position plus the size of the write buffer. Intermediate bytes would be left undetermined.
bfile_pos ()
Return the current position of the file pointer.
uint64_t bfile_pos(const File *file);
file | File handler. |
Return
Position from start of file.
bfile_delete ()
Delete a file from the file system.
bool_t bfile_delete(const char_t *pathname, ferror_t *error);
pathname | File name including its absolute or relative path. |
error | Error code if the function fails. Can be |
Return
TRUE
if the file has been deleted, or FALSE
if any error has occurred.
bfile_rename ()
Renames a file in the file system.
bool_t bfile_rename(const char_t *current_pathname, const char_t *new_pathname, ferror_t *error);
current_pathname | Current name of the file including its absolute or relative path. |
new_pathname | New file name including its absolute or relative path. |
error | Error code if the function fails. It can be |
Return
TRUE
if the file has been renamed, or FALSE
if an error has occurred.