TextView
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
TextView* | textview_create (void) |
void | textview_OnFilter (...) |
void | textview_OnFocus (...) |
void | textview_size (...) |
void | textview_clear (...) |
uint32_t | textview_printf (...) |
void | textview_writef (...) |
void | textview_rtf (...) |
void | textview_units (...) |
void | textview_family (...) |
void | textview_fsize (...) |
void | textview_fstyle (...) |
void | textview_color (...) |
void | textview_bgcolor (...) |
void | textview_pgcolor (...) |
void | textview_halign (...) |
void | textview_lspacing (...) |
void | textview_bfspace (...) |
void | textview_afspace (...) |
void | textview_scroll_visible (...) |
void | textview_move_caret (...) |
void | textview_editable (...) |
const char_t* | textview_get_text (...) |
void | textview_copy (...) |
void | textview_cut (...) |
void | textview_paste (...) |
TextView are views designed to work with rich text blocks (Figure 1), where fonts, sizes and colors can be combined. We can consider them as the basis of a text editor. In Hello TextView! you have an example of use.
- Use textview_create to create a text view.
- Use textview_writef to add text to the view.
- Use textview_printf to add text in the format of
printf
. - Use textview_rtf to add content in Microsoft RTF format.
- Use textview_clear to erase all text.
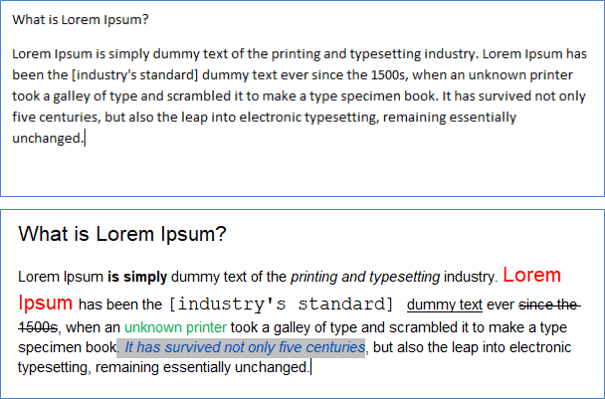
1. Character format
One of the advantages of rich text over plain text is the ability to combine different character formats within the same paragraph (Figure 2). Changes will be applied to new text added to the control.
Use textview_family to change the font.
Use textview_fsize to change the character size.
Use textview_fstyle to change the style.
Use textview_color to change the color of the text.
Use textview_bgcolor to change the background color of the text.
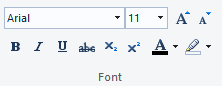
2. Paragraph format
You can also set attributes per paragraph (Figure 3). The new line character '\n'
is considered the closing or end of the paragraph.
Use textview_halign to set to paragraph alignment.
Use textview_lspacing to set line spacing (line spacing).
Use textview_bfspace to indicate the vertical space before the paragraph.
Use textview_afspace to indicate the vertical space after the paragraph.
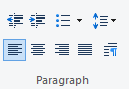
3. Document format
Finally we have several attributes that affect the entire document or control.
Use textview_units to set the text units.
Use textview_pgcolor to set the background color of the control (page).
textview_create ()
Create a text view.
TextView* textview_create(void);
Return
The text view.
textview_OnFilter ()
Set a handler to filter text while editing.
void textview_OnFilter(TextView *view, Listener *listener);
view | The view. |
listener | Callback function that will be called after each key press. In EvTextFilter of event_result the filtered text will be returned. |
Remarks
See Filter texts and GUI Events.
textview_OnFocus ()
Sets a handler for keyboard focus.
void textview_OnFocus(TextView *view, Listener *listener);
view | The view. |
listener | callback function that will be called when keyboard focus is received or lost. |
Remarks
See GUI Events.
textview_size ()
Sets the default size of the view.
void textview_size(TextView *view, const S2Df size);
view | The view. |
size | The size. |
Remarks
It corresponds to the Natural sizing of the control. Default 245x144.
textview_clear ()
Clears all content from view.
void textview_clear(TextView *view);
view | The view. |
textview_printf ()
Writes text to the view, using the format of the printf
.
uint32_t textview_printf(TextView *view, const char_t *format, ...);
1 |
textview_printf(view, Code: %-10s Price %5.2f\n", code, price);
|
view | The view. |
format | String in type-printf format with a variable number of parameters. |
... | Printf arguments or variables. |
Return
The number of bytes written.
textview_writef ()
Write a C UTF8 string to the view.
void textview_writef(TextView *view, const char_t *str);
view | The view. |
str | String C UTF8 terminated in null character |
textview_rtf ()
Insert text in Microsoft RTF format.
void textview_rtf(TextView *view, Stream *rtf_in);
view | The view. |
rtf_in | Reading stream with RTF content. |
textview_units ()
Sets the text units.
void textview_units(TextView *view, const uint32_t units);
view | The view. |
units |
Remarks
ekFPOINTS is the default value and the one normally used by word processors. See Size in points.
textview_family ()
Sets the font family of the text ("Arial", "Times New Roman", "Helvetica", etc).
void textview_family(TextView *view, const char_t *family);
view | The view. |
family | The font family. |
Remarks
Not all families will be present on all platforms. Use font_exists_family or font_installed_families to check.
textview_fsize ()
Set the text size.
void textview_fsize(TextView *view, const real32_t size);
view | The view. |
size | The size. |
Remarks
The value is conditional on the units established in textview_units.
textview_fstyle ()
Sets the text style.
void textview_fstyle(TextView *view, const uint32_t fstyle);
view | The view. |
fstyle | Combination of ekFBOLD, ekFITALIC, ekFSTRIKEOUT, ekFUNDERLINE ekFSUBSCRIPT, ekFSUPSCRIPT. To override any previous style use ekFNORMAL. |
textview_color ()
Sets the text color.
void textview_color(TextView *view, const color_t color);
view | The view. |
color | The color. Use kCOLOR_DEFAULT to restore the default color. |
textview_bgcolor ()
Sets the background color of the text.
void textview_bgcolor(TextView *view, const color_t color);
view | The view. |
color | The color. Use kCOLOR_DEFAULT to restore the default color. |
textview_pgcolor ()
Sets the background color of the control.
void textview_pgcolor(TextView *view, const color_t color);
view | The view. |
color | The color. Use kCOLOR_DEFAULT to restore the default color. |
textview_halign ()
Sets the alignment of text in a paragraph.
void textview_halign(TextView *view, const align_t align);
view | The view. |
align | The alignment. By default ekLEFT. |
textview_lspacing ()
Sets the line spacing of the paragraph.
void textview_lspacing(TextView *view, const real32_t scale);
view | The view. |
scale | Scale factor in font height. |
textview_bfspace ()
Sets a vertical space before the paragraph.
void textview_bfspace(TextView *view, const real32_t space);
view | The view. |
space | The space in the preset units. |
textview_afspace ()
Sets a vertical space after the paragraph.
void textview_afspace(TextView *view, const real32_t space);
view | The view. |
space | The space in the preset units. |
textview_scroll_visible ()
Show or hide scroll bars.
void textview_scroll_visible(TextView *view, const bool_t horizontal, const bool_t vertical);
view | The view. |
horizontal | Horizontal bar. |
vertical | Vertical bar. |
textview_move_caret ()
Position the cursor on the indicated character.
void textview_move_caret(TextView *view, const int64_t pos);
view | The view. |
pos | Position. |
textview_editable ()
Sets whether or not the control text is editable.
void textview_editable(TextView *view, const bool_t is_editable);
view | The view. |
is_editable |
|
textview_get_text ()
Gets the text of the control.
const char_t* textview_get_text(const TextView *view);
view | The view. |
Return
Null-terminated UTF8 C string '\0'
.
textview_copy ()
Copies the selected text to the clipboard.
void textview_copy(const TextView *view);
view | The view. |
Remarks
See Clipboard operations.
textview_cut ()
Cuts the selected text, copying it to the clipboard.
void textview_cut(TextView *view);
view | The view. |
Remarks
See Clipboard operations.
textview_paste ()
Pastes the text from the clipboard into the caret position.
void textview_paste(TextView *view);
view | The view. |
Remarks
See Clipboard operations.