2D Segments
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
Seg2D | seg2d (...) |
Seg2D | seg2d_v (...) |
real | seg2d_length (...) |
real | seg2d_sqlength (...) |
V2D | seg2d_eval (...) |
real | seg2d_close_param (...) |
real | seg2d_point_sqdist (...) |
real | seg2d_sqdist (...) |
Segments are fragments of a line between two points p0 and p1 (Figure 1). They are the simplest geometric primitives, after vectors. We define the t parameter as the normalized position within the segment. Values between 0
and 1
will correspond to internal points of the segment, with the limits t=0
(p0
) and t=1
(p1
). Out of this range we will have the points outside the segment, but within the line that contains it. For example t=2
would be the point after p1
located at a distance equal to the length of the segment.
- Use seg2d_lengthf to get the length of the segment.
- Use seg2d_close_paramf to get the value of the parameter closest to a certain point.
- Use seg2d_evalf to get the point from the parameter.
- Use seg2d_sqdistf to get the distance (squared) between two segments.
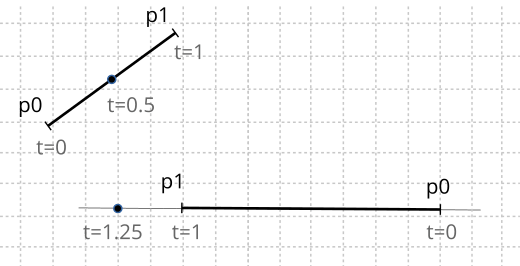
seg2d ()
Create a 2d segment from its components.
Seg2Df seg2df(const real32_t x0, const real32_t y0, const real32_t x1, const real32_t y1); Seg2Dd seg2dd(const real64_t x0, const real64_t y0, const real64_t x1, const real64_t y1); Seg2D Seg2D(const real x0, const real y0, const real x1, const real y1);
x0 | X coordinate of the first point. |
y0 | Y coordinate of the first point. |
x1 | X coordinate of the second point. |
y1 | Y coordinate of the second point. |
Return
The 2d segment.
seg2d_v ()
Create a 2d segment from two points.
Seg2Df seg2d_vf(const V2Df *p0, const V2Df *p1); Seg2Dd seg2d_vd(const V2Dd *p0, const V2Dd *p1); Seg2D Seg2D::v(const V2D *p0, const V2D *p1);
p0 | First point. |
p1 | Second point. |
Return
The 2d segment.
seg2d_length ()
Gets the length of the segment.
real32_t seg2d_lengthf(const Seg2Df *seg); real64_t seg2d_lengthd(const Seg2Dd *seg); real Seg2D::length(const Seg2D *seg);
seg | Segment. |
Return
Length.
seg2d_sqlength ()
Gets the square of the segment length.
real32_t seg2d_sqlengthf(const Seg2Df *seg); real64_t seg2d_sqlengthd(const Seg2Dd *seg); real Seg2D::sqlength(const Seg2D *seg);
seg | Segment. |
Return
Length square.
Remarks
Avoid calculating square roots if we are only interested in comparing measurements.
seg2d_eval ()
Gets the point in the segment based on the parameter.
V2Df seg2d_evalf(const Seg2Df *seg, const real32_t t); V2Dd seg2d_evald(const Seg2Dd *seg, const real64_t t); V2D Seg2D::eval(const Seg2D *seg, const real t);
seg | Segment. |
t | Parameter. |
Return
Point on the segment (or on the line that contains it).
Remarks
If t=0
it returns p0
. If t=1
it returns p1
. Values between (0,1)
points within the segment. Other values, points on the line that contains the segment.
seg2d_close_param ()
Gets the parameter of the segment closest to a given point.
real32_t seg2d_close_paramf(const Seg2Df *seg, const V2Df *pnt); real64_t seg2d_close_paramd(const Seg2Dd *seg, const V2Dd *pnt); real Seg2D::close_param(const Seg2D *seg, const V2D *pnt);
seg | Segment. |
pnt | Point. |
Return
Parameter. See seg2d_evalf.
seg2d_point_sqdist ()
Gets the squared distance from a point to the segment.
real32_t seg2d_point_sqdistf(const Seg2Df *seg, const V2Df *pnt, real32_t *t); real64_t seg2d_point_sqdistd(const Seg2Dd *seg, const V2Dd *pnt, real64_t *t); real Seg2D::point_sqdist(const Seg2D *seg, const V2D *pnt, real *t);
seg | Segment. |
pnt | Point. |
t | Parameter on the line that contains the segment. See seg2d_close_paramf. It can be |
Return
Distance square.
seg2d_sqdist ()
Gets the squared distance between two segments.
real32_t seg2d_sqdistf(const Seg2Df *seg1, const Seg2Df *seg2, real32_t *t1, real32_t *t2); real64_t seg2d_sqdistd(const Seg2Dd *seg1, const Seg2Dd *seg2, real64_t *t1, real64_t *t2); real Seg2D::sqdist(const Seg2D *seg1, const Seg2D *seg2, real *t1, real *t2);
seg1 | First segment. |
seg2 | Second segment. |
t1 | Nearest parameter in |
t2 | Nearest parameter in |
Return
Distance square.