Palettes
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
Palette* | palette_create (...) |
Palette* | palette_cga2 (...) |
Palette* | palette_ega4 (void) |
Palette* | palette_rgb8 (void) |
Palette* | palette_gray1 (void) |
Palette* | palette_gray2 (void) |
Palette* | palette_gray4 (void) |
Palette* | palette_gray8 (void) |
Palette* | palette_binary (...) |
void | palette_destroy (...) |
uint32_t | palette_size (...) |
color_t* | palette_colors (...) |
const color_t* | palette_colors_const (...) |
A palette is nothing more than an indexed list of colors (Figure 1), usually related to Pixel Buffer. Its main utility is to save space in the images representation, since each pixel is encoded by an index of 1, 2, 4 or 8 bits instead of the real color where 24 or 32 bits are necessary. For this reason, it is usual to have palettes of 2, 4, 16 or 256 colors.
- Use palette_create to create a palette.
- Use palette_colors to access the elements.
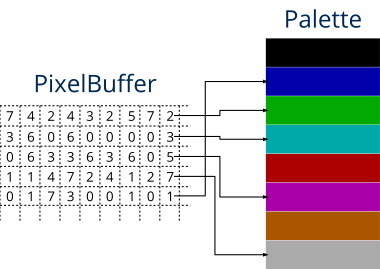
1. Predefined palette
We have several predefined palettes both in color (Figure 2) and in grays (Figure 3). The RGB8 palette has been created by combining 8 tones of red (3bits), 8 tones of green (3bits) and 4 tones of blue (2bits). This is so because the human eye distinguishes much less the variation of blue than the other two colors.
- Use palette_ega4 to create a predefined palette of 16 colors.
- Use palette_rgb8 to create a 256 color palette.
- Use palette_gray4 and similars to create a palette in grays.
- Use palette_binary for a two-color palette.
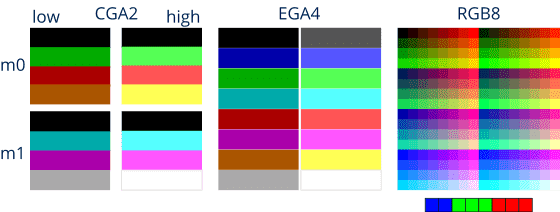
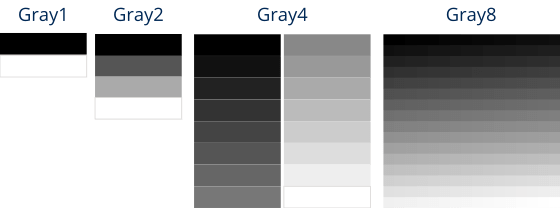
palette_create ()
Create a palette.
Palette* palette_create(const uint32_t size);
size | The number of colors. |
Return
The palette. The initial content is undetermined. Edit with palette_colors.
palette_cga2 ()
Create the 4-color (2-bit) palette of CGA cards.
Palette* palette_cga2(const bool_t mode, const bool_t intense);
mode |
|
intense |
|
Return
The palette.
Remarks
palette_ega4 ()
Create the default palette for EGA cards (16 colors, 4 bits).
Palette* palette_ega4(void);
Return
The palette.
Remarks
palette_rgb8 ()
Create the default 8-bit RGB palette. Colors combine 8 tones of red, 8 green and 4 blue.
Palette* palette_rgb8(void);
Return
The palette.
Remarks
palette_gray1 ()
Create a palette of 2 tones of gray (1 bit). Black (0) and white (1).
Palette* palette_gray1(void);
Return
The palette.
Remarks
palette_gray2 ()
Create a palette of 4 tones of gray (2 bit). Black (0), White (3).
Palette* palette_gray2(void);
Return
The palette.
Remarks
palette_gray4 ()
Create a palette of 16 tones of gray (4 bit). Black (0), White (15).
Palette* palette_gray4(void);
Return
The palette.
Remarks
palette_gray8 ()
Create a palette of 256 shades of gray (8 bit). Black (0), White (255).
Palette* palette_gray8(void);
Return
The palette.
Remarks
palette_binary ()
Create a two-color palette.
Palette* palette_binary(const color_t zero, const color_t one);
zero | Color associated with the |
one | Color associated with the |
Return
The palette.
palette_destroy ()
Destroy the palette.
void palette_destroy(Palette **palette);
palette | The palette. It will be set to |
palette_size ()
Returns the number of colors in the palette.
uint32_t palette_size(const Palette *palette);
palette | The palette. |
Return
The number of colors.
palette_colors ()
Get the color list.
color_t* palette_colors(Palette *palette);
palette | The palette. |
Return
Colors. The size of the array is given by palette_size.
Remarks
The buffer is read/write.
palette_colors_const ()
Get the color list.
const color_t* palette_colors_const(const Palette *palette);
palette | The palette. |
Return
Colors. The size of the array is given by palette_size.